Exception handling is a critical concept in programming that allows you to manage errors gracefully without crashing your application. In JavaScript, exceptions occur when something goes wrong during code execution, like calling a function that doesn’t exist or attempting to parse invalid JSON. This tutorial will guide you through the basics of exception handling in JavaScript.
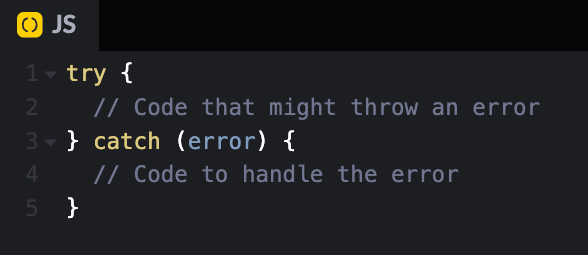
What is Exception Handling in JavaScript?
Exception handling refers to the process of managing errors and exceptions in your code. Instead of letting the program crash when an error occurs, you can handle it using JavaScript's built-in try...catch mechanism. It is a structured way to detect, catch, and resolve errors during runtime, allowing your application to recover or degrade gracefully. By incorporating exception handling, developers can anticipate potential errors, maintain application stability, and provide a better user experience. Properly handled exceptions also make debugging easier, as you can log meaningful error messages and track down the root cause of issues without guesswork.
Why is Error Handling Important?
Error handling is a critical aspect of software development that ensures the robustness and reliability of your programs. It involves anticipating and gracefully managing unexpected situations or errors that might occur during the execution of your code.
Enhanced User Experience: By gracefully handling errors, you can prevent your program from crashing unexpectedly, providing a smoother and more user-friendly experience.
Improved Program Stability: Robust error handling helps to prevent unexpected program behavior, making your software more stable and less prone to crashes.
Easier Debugging: Well-implemented error handling can make it easier to identify and fix bugs, as errors are often caught and reported in a controlled manner.
Data Integrity: Error handling can help to protect the integrity of your data by preventing invalid or corrupted data from being processed or stored.
Common Error Handling Techniques
Try-Catch Blocks:
try: Encloses the code that might throw an exception.
catch: Executes if an exception is thrown within the try block, allowing you to handle the error gracefully.
finally: (Optional) Executes regardless of whether an exception occurred or not, often used for cleanup operations.
Error Objects:
Represent specific errors with details like error messages, error codes, and potential solutions.
Allow you to provide more informative error messages to users or for debugging purposes.
Assertion Statements:
Used to check for conditions that should always be true.
If the condition is false, an assertion error is raised, indicating a potential bug in your code.
Logging:
Recording error messages and other relevant information to a log file.
Helps in debugging and identifying the root cause of errors.
The try...catch Syntax
The try...catch block is the most commonly used structure for exception handling in JavaScript. It helps isolate potentially error-prone code and provides a mechanism to handle errors without disrupting the rest of the application.
Syntax:
try {
// Code that might throw an error
} catch (error) {
// Code to handle the error
}
try block: Contains the code you want to execute. If an error occurs, JavaScript stops executing this block and jumps to the catch block.
catch block: Executes when an error is thrown in the try block. You can access the error object for more details, which includes properties like message, name, and stack for debugging.
Error Object: The error object in the catch block gives you valuable details about what went wrong and can be used to debug or log the error efficiently.
Example:
try {
let result = 10 / 0; // No error
console.log(result);
JSON.parse("{invalidJson}"); // This will throw an error
} catch (error) {
console.error("An error occurred:", error.message);
}
Throwing Your Own Exceptions
Throwing your own exceptions in JavaScript allows you to handle specific error conditions and communicate them effectively within your code. In the example provided, the divide function checks if the denominator (b) is zero. If it is, the function throws a custom error using throw new Error("Division by zero is not allowed.");. This stops the execution of the function and sends the error to be handled. The try block is used to execute the function, and if an exception occurs, the catch block captures the error. Inside the catch block, the error's message is logged with a custom prefix, "Custom Error:". This approach helps in maintaining code reliability and provides meaningful feedback when invalid conditions occur.
Example:
function divide(a, b) {
if (b === 0) {
throw new Error("Division by zero is not allowed.");
}
return a / b;
}
try {
console.log(divide(10, 0));
} catch (error) {
console.error("Custom Error:", error.message);
}
The finally Block
The finally block is used to execute code after the try and catch blocks, regardless of whether an error was thrown or not.
Syntax:
try {
// Code that might throw an error
} catch (error) {
// Code to handle the error
} finally {
// Code that will always run
}
Example:
try {
console.log("Trying...");
throw new Error("Oops!");
} catch (error) {
console.error("Caught an error:", error.message);
} finally {
console.log("This will always run.");
}
Common Use Cases of Exception Handling
Exception handling plays a crucial role in making applications robust and user-friendly. It allows developers to anticipate and manage errors in real-time, preventing them from escalating into critical failures. By catching and resolving errors, you can maintain seamless functionality, enhance user experience, and ensure your application behaves predictably under unexpected circumstances. Here are some scenarios where exception handling is commonly used:
Handling API Errors
async function fetchData(url) {
try {
let response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Failed to fetch data:", error.message);
}
}
fetchData("https://api.example.com/data");
Validating User Input
function validateAge(age) {
try {
if (isNaN(age)) {
throw new Error("Age must be a number.");
}
if (age < 18) {
throw new Error("You must be 18 or older.");
}
console.log("Age is valid.");
} catch (error) {
console.error("Validation Error:", error.message);
}
}
validateAge("abc");
Best Practices for Exception Handling
Effective exception handling ensures that your code is robust, maintainable, and user-friendly. By following best practices, you can minimize the risk of unhandled errors disrupting your application and improve its overall reliability. This section highlights key strategies for writing clean and efficient error-handling code.
Be Specific with Error Handling
Catch specific errors rather than using a generic catch-all.
try {
// Specific error handling
} catch (error) {
if (error instanceof TypeError) {
console.error("Type Error:", error.message);
} else {
console.error("General Error:", error.message);
}
}
Avoid Using Exceptions for Flow Control
Don't use exceptions to manage normal application flow. They should only be used for unexpected situations.
Log Errors for Debugging
Always log errors with enough context to make debugging easier.
Graceful Degradation
Provide fallback functionality when an error occurs to improve user experience.
Conclusion
Exception handling in JavaScript is an essential skill that helps you write robust and reliable applications. By using try...catch, finally, and the throw keyword, you can catch errors gracefully and ensure your application continues running smoothly. Proper error management not only enhances the user experience but also makes debugging and maintaining your codebase much easier.
Additionally, exception handling empowers developers to anticipate potential issues and design applications that degrade gracefully under failure conditions. Whether you’re fetching data from an API, validating user inputs, or handling critical errors in your application logic, mastering exception handling will help you create more resilient and professional software.
Remember to apply best practices to make your code clean, efficient, and maintainable. Exception handling is not just about fixing errors—it's about writing code that gracefully adapts to unforeseen situations and keeps your application stable.
Comments