A Beginner's Guide to JavaScript Classes
- Samul Black
- Jan 22
- 6 min read
JavaScript, a versatile and widely used programming language, introduces the concept of classes to bring a more structured approach to object-oriented programming (OOP). Classes, introduced in ECMAScript 2015 (ES6), provide a convenient syntax for creating and managing objects and their associated behaviours.
This tutorial will walk you through the fundamentals of JavaScript classes with clear examples to help you get started.
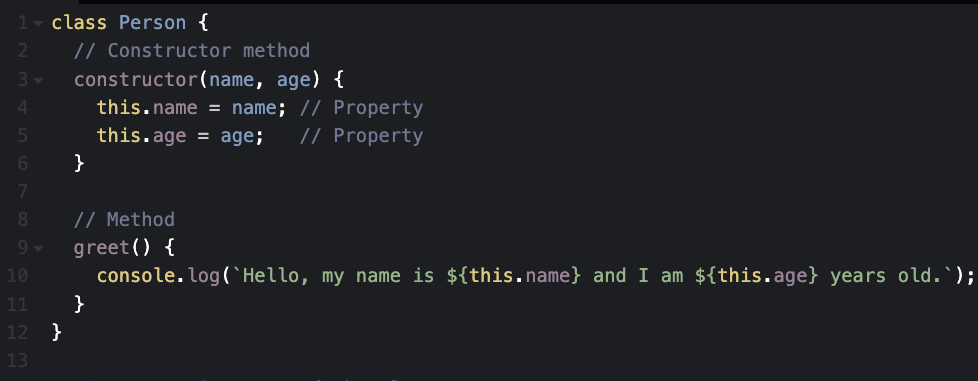
What Are Classes in JavaScript?
Classes in JavaScript are a template for creating objects, enabling developers to implement object-oriented programming principles. Introduced in ECMAScript 2015 (ES6), classes provide a more structured and readable way to define and manage objects compared to the traditional prototype-based approach. A class encapsulates data (properties) and behaviors (methods) into a single construct, making it easier to model real-world entities. They include key components like constructors, which initialize the object, and methods, which define its behavior. Classes also support inheritance, allowing one class to extend another, promoting code reuse and modular design. Despite their modern syntax, JavaScript classes are syntactic sugar over its prototype-based inheritance model.
Importance of Classes in JavaScript
Classes in JavaScript are a fundamental feature for implementing object-oriented programming (OOP) in web development. They provide a clear and concise way to create and manage reusable, modular code by defining templates for objects. Classes enable developers to encapsulate data (properties) and behavior (methods) within a single unit, making it easier to model real-world entities and interactions. They also simplify inheritance, allowing one class to extend another and promote code reusability. This makes classes particularly valuable for building complex applications with a clean and maintainable architecture. By leveraging classes, developers can enhance readability, reduce redundancy, and ensure more efficient debugging and testing of their code.
Defining a Class
In JavaScript, classes provide a blueprint for creating objects that encapsulate data (properties) and behavior (methods). Classes are defined using the class keyword, followed by the class name and a set of curly braces {} containing its definition. Here's an example:
class Person {
// Constructor method
constructor(name, age) {
this.name = name; // Property
this.age = age; // Property
}
// Method
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
// Create an instance of the class
const person1 = new Person("Alice", 30);
person1.greet(); // Output: Hello, my name is Alice and I am 30 years old.
The provided code demonstrates the definition and usage of a class in JavaScript. The Person class serves as a blueprint for creating objects with properties and methods. It includes a special constructor method that initializes the name and age properties when a new instance of the class is created. The greet method is defined within the class to access these properties and display a personalized message. An instance of the class, person1, is created using the new keyword, passing "Alice" and 30 as arguments to the constructor. This initializes the instance's name and age properties. When the greet method is called on person1, it outputs a message to the console, demonstrating how classes encapsulate data and behavior for reusable and organised code.
A Typical Class consists of following key components:
1. Constructor
The constructor method is a special method used for initialising objects created by the class. It runs automatically when a new instance is created.
2. Methods
Classes can have methods that define the behaviour of objects.
class Calculator {
add(a, b) {
return a + b;
}
}
const calc = new Calculator();
console.log(calc.add(5, 3)); // Output: 8
3. Static Methods
Static methods belong to the class itself rather than instances of the class. They are defined using the static keyword.
class MathUtils {
static square(number) {
return number * number;
}
}
console.log(MathUtils.square(4)); // Output: 16
Inheritance in Classes
Classes support inheritance, allowing you to create subclasses that inherit properties and methods from a parent class. Use the extends keyword for inheritance and the super keyword to call the parent class's constructor or methods.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog("Buddy");
dog.speak(); // Output: Buddy barks.
The provided code demonstrates how inheritance is implemented in JavaScript using classes. The Animal class is defined as a base class with a constructor method that initializes the name property and a speak method that outputs a generic message indicating the animal makes a sound. The Dog class extends the Animal class, inheriting its properties and methods. By overriding the speak method, the Dog class provides a more specific implementation that outputs a message indicating the dog's barking behavior. An instance of the Dog class, dog, is created with the name "Buddy". When dog.speak() is called, it invokes the overridden speak method in the Dog class, outputting Buddy barks. This example illustrates how inheritance allows subclasses to customize or extend the functionality of a base class, enabling code reuse and a hierarchical structure for related objects.
Getters and Setters
Getters and setters allow you to define how properties are accessed or modified.
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
get area() {
return this.width * this.height;
}
set dimensions({ width, height }) {
this.width = width;
this.height = height;
}
}
const rect = new Rectangle(5, 10);
console.log(rect.area); // Output: 50
rect.dimensions = { width: 7, height: 14 };
console.log(rect.area); // Output: 98
The provided code illustrates how getters and setters are used in JavaScript classes to manage properties dynamically. The Rectangle class is defined with a constructor that initializes the width and height properties when a new instance is created. The get area accessor method calculates and returns the area of the rectangle by multiplying its width and height. This allows the area to be accessed as a property rather than a method call. The set dimensions mutator method updates the width and height properties using an object with new values.
An instance of the class, rect, is created with an initial width of 5 and height of 10, resulting in an area of 50. When the dimensions setter is invoked with new values { width: 7, height: 14 }, the properties of the instance are updated. Subsequently, accessing the area getter reflects the updated dimensions, returning an area of 98. This example demonstrates how getters and setters enhance encapsulation and provide a clean, intuitive interface for interacting with an object's properties.
Private Fields and Methods
Introduced in ES2022, private fields and methods start with a # symbol and are accessible only within the class.
class BankAccount {
#balance = 0; // Private field
deposit(amount) {
this.#balance += amount;
console.log(`Deposited ${amount}. New balance: ${this.#balance}`);
}
getBalance() {
return this.#balance;
}
}
const account = new BankAccount();
account.deposit(100); // Output: Deposited 100. New balance: 100
console.log(account.getBalance()); // Output: 100
The provided code showcases the use of private fields in JavaScript classes to enforce encapsulation. The BankAccount class includes a private field, #balance, which can only be accessed or modified within the class itself. This field is initialized to 0 and is not directly accessible from outside the class, ensuring sensitive data remains secure.
The class provides a deposit method that accepts an amount, adds it to the #balance, and logs the updated balance. Additionally, the getBalance method is defined to return the current balance, providing controlled access to the private field.
An instance of the BankAccount class, account, is created. Using the deposit method, 100 is added to the account, and the updated balance is displayed. The getBalance method confirms the current balance is 100. Attempts to access #balance directly (e.g., account.#balance) would result in an error, demonstrating the power of private fields in encapsulating and safeguarding internal data.
Conclusion
JavaScript classes provide a clean and efficient way to create and manage objects in an object-oriented style. Whether you're building small utilities or complex applications, mastering classes will enhance your ability to write structured, maintainable, and reusable code. By encapsulating data and behavior within a single unit, classes promote better organization and reduce redundancy, making your projects easier to scale and debug.
By exploring the concepts covered in this guide, you’re now equipped to start using JavaScript classes in your projects. Experiment with different features such as inheritance, private fields, getters, setters etc to fully utilize the capabilities of classes. As you delve deeper, you’ll discover how classes can simplify complex coding tasks and provide a robust foundation for modern JavaScript applications. Mastery of classes is a valuable skill that will empower you to write clean, efficient, and professional-grade code.
Comments