Pandas is one of the most powerful and versatile libraries in Python, specifically designed for data manipulation and analysis. Whether you're a data scientist, analyst, or just a Python enthusiast, understanding how to use Pandas effectively can significantly boost your productivity and efficiency when working with data. In this blog, we'll explore the fundamentals of Pandas, covering key concepts, functions, and real-world examples to help you get started.
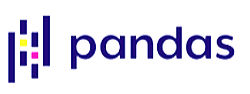
What is Pandas in Python?
Pandas is an open-source data analysis and manipulation library built on top of Python’s NumPy library. It provides high-performance, easy-to-use data structures like DataFrames and Series, which are essential for handling structured data. With Pandas, you can load data from various file formats, clean and preprocess it, perform complex operations, and even visualize your data.
Pandas is widely used in the data science community because it simplifies many data-related tasks, allowing users to focus more on analysis and less on coding. Pandas primarily relies on two core data structures:
Pandas Series
A one-dimensional labeled array capable of holding any data type. It’s like a column in a spreadsheet or a database table. Each element in a Series has an associated label, or index.
import pandas as pd
# Creating a Series
data = pd.Series([10, 20, 30, 40], index=['a', 'b', 'c', 'd'])
print(data)
Output for the above code:
a 10
b 20
c 30
d 40
dtype: int64
Pandas DataFrame
A two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). A DataFrame is essentially a collection of Series that share the same index.
# Creating a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [24, 27, 22, 32],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston']
}
df = pd.DataFrame(data)
print(df)
Output for the above code:
Name Age City
0 Alice 24 New York
1 Bob 27 Los Angeles
2 Charlie 22 Chicago
3 David 32 Houston
Essential Pandas Operations
Pandas offers a wide array of functions and methods that make data manipulation straightforward and efficient. Below are some of the most commonly used operations:
1. Loading CSV file in Pandas
Pandas can load data from various formats, including CSV, Excel, JSON, and SQL databases. Here's how to read data from a CSV file:
# Load csv
df = pd.read_csv('data.csv')
2. Inspecting Data in Pandas
Once you have your data loaded into a DataFrame, you can quickly get a sense of what it looks like:
df.head() - Displays the first few rows of the DataFrame.
df.info() - Provides a concise summary of the DataFrame.
df.describe() - Generates descriptive statistics for numerical columns.
3. Data Selection in Pandas
You can select specific rows, columns, or subsets of data using various techniques:
Selecting Columns: df['ColumnName']
Selecting Rows by Index: df.iloc[0] or df.loc['RowLabel']
Filtering Data: df[df['Age'] > 25]
4. Data Cleaning in Pandas
Cleaning data is a crucial step in any data analysis project. Pandas provides numerous tools for this:
Handling Missing Data: df.dropna() removes missing values, while df.fillna(value) replaces them with a specified value.
Renaming Columns: df.rename(columns={'OldName': 'NewName'})
Dropping Columns: df.drop('ColumnName', axis=1)
5. Data Aggregation in Pandas
You can easily group and aggregate data to perform operations like sum, mean, or count:
# Data aggregation grouped = df.groupby('City')['Age'].mean()
print(grouped)
Output for the above code:
City
Chicago 22.0
Houston 32.0
Los Angeles 27.0
New York 24.0
Name: Age, dtype: float64
6. Merging and Joining
Combining multiple DataFrames is often necessary when dealing with large datasets:
Merging: pd.merge(df1, df2, on='KeyColumn')
Joining: df1.join(df2, how='inner')
Practical Example: Analyzing Sales Data with Pandas in Python
Let’s consider a practical example where you analyze a dataset of sales transactions to gain insights.
# Sample DataFrame
data = {
'Date': ['2024-01-01', '2024-01-02', '2024-01-03', '2024-01-04'],
'Product': ['A', 'B', 'A', 'C'],
'Sales': [150, 200, 100, 250]
}
df = pd.DataFrame(data)
# Convert 'Date' to datetime
df['Date'] = pd.to_datetime(df['Date'])
# Calculate total sales for each product
total_sales = df.groupby('Product')['Sales'].sum()
print(total_sales)
# Filter sales above a certain threshold
high_sales = df[df['Sales'] > 150]
print(high_sales)
Output for the above code:
Product
A 250
B 200
C 250
Name: Sales, dtype: int64
Date Product Sales
1 2024-01-02 B 200
3 2024-01-04 C 250
This example demonstrates how Pandas can be used to preprocess data, perform aggregations, and filter results to derive meaningful insights.
Conclusion
Pandas is an indispensable tool for anyone working with data in Python. Its intuitive syntax and powerful data structures make it easy to clean, manipulate, and analyze data, allowing you to focus on uncovering insights rather than writing complex code. Whether you're dealing with small datasets or large-scale data processing tasks, Pandas provides the functionality you need to handle data efficiently.
By mastering Pandas, you'll be well-equipped to tackle a wide range of data challenges, from simple data exploration to complex data science workflows. Start experimenting with Pandas today, and unlock the full potential of your data!
Comentários