A Comprehensive Guide to JavaScript Class Method Types
- Samul Black
- Jan 24
- 4 min read
JavaScript classes are a core feature of modern JavaScript that enable developers to use object-oriented programming paradigms effectively. A class can have various types of methods, each serving a specific purpose. In this tutorial, we will explore the different types of class methods in JavaScript, including instance methods, static methods, and getter/setter methods.
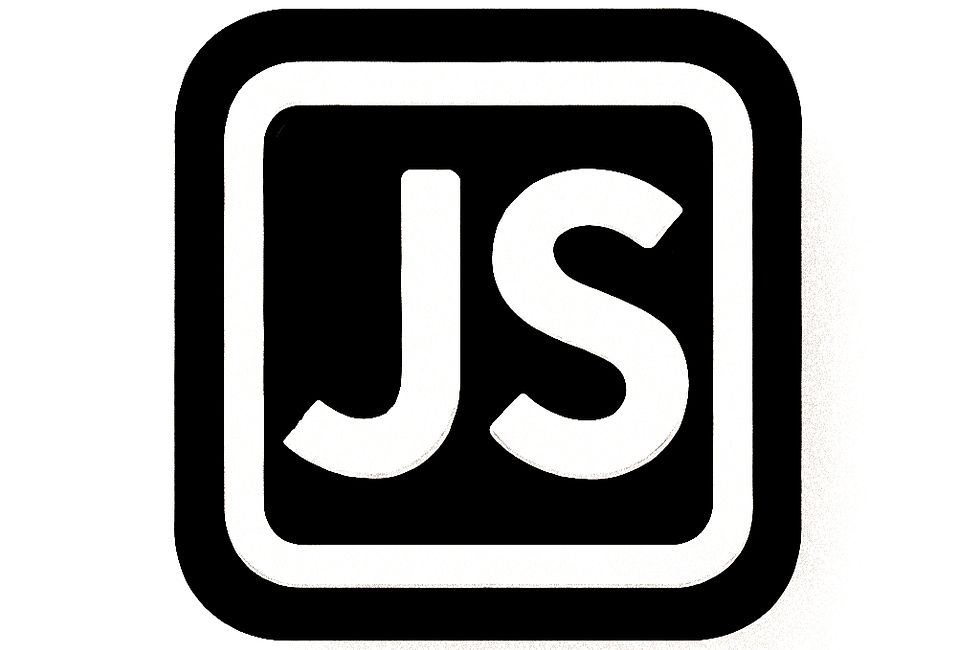
What are Classes in JavaScript?
Classes in JavaScript are a blueprint for creating objects with shared structure and behavior. They encapsulate data and methods that operate on that data, making it easier to organize and manage code. Introduced in ES6 (ECMAScript 2015), classes provide a cleaner and more intuitive syntax for creating objects and implementing inheritance compared to the older prototype-based approach. By using classes, developers can write reusable, modular, and maintainable code. They are especially useful in complex applications where multiple objects share similar behavior or need to interact with each other in a consistent way.
Class Methods in JavaScript
JavaScript classes are a cornerstone of modern web development, providing a clean and structured way to build reusable components and organize your code. But within these classes lies a rich variety of methods, each designed to handle specific tasks and interactions. From instance methods that define the behavior of objects to static methods that belong to the class itself, these tools help developers tackle problems with precision and clarity. There’s also the elegance of getters and setters for property management and private methods for safeguarding sensitive operations. Understanding these different types of methods isn’t just about mastering syntax—it’s about unlocking the potential to write code that feels intuitive, efficient, and a joy to work with. Let’s explore these methods and see how they can empower you to create smarter, more maintainable applications. Some of these class methods are listed below:
1. Instance Methods
Instance methods are regular methods that belong to the instance of the class. They can only be called on objects created from the class using the new keyword.
Syntax:
class MyClass {
instanceMethod() {
console.log('This is an instance method.');
}
}
const obj = new MyClass();
obj.instanceMethod(); // Output: This is an instance method.
Key Characteristics:
They are defined without the static keyword.
Accessed via class instances, not the class itself.
Useful for operations specific to a particular instance.
2. Static Methods
Static methods belong to the class itself, rather than instances of the class. They are typically used for utility or helper functions.
Syntax:
class MyClass {
static staticMethod() {
console.log('This is a static method.');
}
}
MyClass.staticMethod(); // Output: This is a static method.
Key Characteristics:
Defined using the static keyword.
Cannot be called on class instances.
Commonly used for functionality that does not depend on instance properties.
Example Use Case:
Static methods are often used to create utility libraries.
class MathUtils {
static add(a, b) {
return a + b;
}
}
console.log(MathUtils.add(5, 3)); // Output: 8
3. Getter and Setter Methods
Getters and setters allow you to define methods that behave like properties. These are useful for controlling access to an object's properties.
Syntax:
class MyClass {
constructor(value) {
this._value = value;
}
get value() {
return this._value;
}
set value(newValue) {
this._value = newValue;
}
}
const obj = new MyClass(10);
console.log(obj.value); // Output: 10
obj.value = 20;
console.log(obj.value); // Output: 20
Key Characteristics:
get and set keywords define these methods.
Getters are used to retrieve values, and setters are used to modify them.
They provide an abstraction layer for managing properties.
4. Private Methods
Private methods are methods that can only be accessed within the class. They are defined using the # symbol.
Syntax:
class MyClass {
#privateMethod() {
console.log('This is a private method.');
}
publicMethod() {
this.#privateMethod();
}
}
const obj = new MyClass();
obj.publicMethod(); // Output: This is a private method.
// obj.#privateMethod(); // Error: Private field '#privateMethod' must be declared in an enclosing class
Key Characteristics:
Defined with a # prefix.
Cannot be accessed outside the class.
Useful for encapsulation.
5. Asynchronous Methods
Asynchronous methods are defined using the async keyword and allow the use of await inside the method body.
Syntax:
class MyClass {
async fetchData() {
const data = await fetch('https://jsonplaceholder.typicode.com/posts/1');
return data.json();
}
}
const obj = new MyClass();
obj.fetchData().then(console.log);
/* {
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur
expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
*/
Key Characteristics:
Return a Promise.
Facilitate working with asynchronous code inside a class.
Conclusion
Understanding the different types of methods in JavaScript classes helps you write more organised, reusable, and maintainable code. Each method type serves a specific purpose, allowing you to tailor your implementation to the unique requirements of your application. By leveraging these method types effectively, you can create modular and efficient solutions, reduce code duplication, and make debugging and scaling much easier.
Additionally, exploring and experimenting with these method types in real-world scenarios provides invaluable hands-on experience. You'll gain a deeper understanding of their behavior and applications, from encapsulating logic within instance methods to utilizing static methods for utility functions. For example, getter and setter methods can enhance how you interact with object properties, while private methods and fields can enforce encapsulation and ensure your class's internal workings remain secure.
Incorporating these concepts into your coding practices not only improves your programming skills but also enhances the quality of your applications. As you continue to practice and integrate these method types into your projects, you’ll find yourself writing code that is not just functional but also elegant and future-proof. Take the time to master these tools, and you'll unlock new levels of productivity and creativity in your development journey.
Comments