The console object in JavaScript is a developer's best friend when it comes to debugging, logging, and understanding code behavior. It provides a variety of methods that allow developers to interact with their applications via the browser's Developer Tools or Node.js environment.
In this tutorial, we’ll explore the console object and its most commonly used methods with examples.
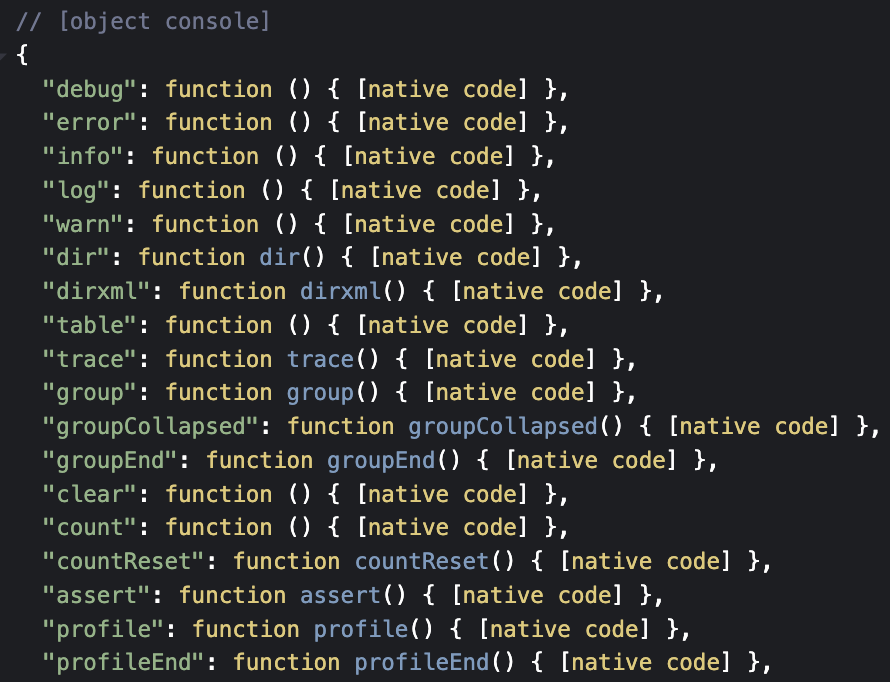
What is the Console Object in JavaScript?
The console object is built into JavaScript and provides access to the browser or runtime's debugging console. It acts as an interface for developers to output messages, log data, and interact with the execution environment during development.
In browsers, the console object is a part of the Web APIs provided by the browser's JavaScript engine, while in Node.js, it is implemented in the runtime environment itself. Both implementations offer similar methods but may have slight differences in behavior and output formatting. The console object can:
Help identify issues in code through error messages.
Provide insights into data structures like arrays, objects, and functions.
Measure the performance of code execution.
Organize output into structured formats like tables and groups.
While its most famous method is console.log, the console object includes many other methods tailored to specific debugging needs, making it an indispensable tool for developers.
Common Methods of the console Object
By using the console object effectively, developers can streamline troubleshooting and gain insights into their code execution. Below, we'll explore some common methods of the console object, such as log(), error(), warn(), info(), and more, and understand how they can enhance debugging and provide meaningful feedback.
1. console.log()
Logs general information to the console.
Commonly used for debugging and inspecting variables.
Example:
console.log("Hello, world!");
let number = 42;
console.log("The number is:", number);
2. console.error()
Logs an error message in red (browser-dependent).
Useful for highlighting issues or exceptions.
Example:
console.error("An error occurred!");
3. console.warn()
Logs warnings, usually highlighted with a yellow background or warning icon.
Helps to differentiate between critical errors and warnings.
Example:
console.warn("This is a warning!");
4. console.info()
Logs informational messages (similar to console.log in many browsers).
Example:
console.info("This is some information.");
5. console.table()
Displays tabular data in a table format, making it easier to visualize objects and arrays.
Example:
const users = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Charlie", age: 35 }
];
console.table(users);
6. console.group() and console.groupEnd()
Organizes related logs into collapsible groups for better readability.
Example:
console.group("User Details");
console.log("Name: Alice");
console.log("Age: 25");
console.groupEnd();
7. console.time() and console.timeEnd()
Measures the time taken for an operation or code block to execute.
Example:
console.time("Timer");
for (let i = 0; i < 1000000; i++) {} // Simulating a task
console.timeEnd("Timer");
8. console.assert()
Logs a message only if the condition provided evaluates to false.
Example:
let x = 10;
console.assert(x > 15, "x is not greater than 15");
9. console.clear()
Clears the console. Use it to declutter logs during development.
Example:
console.clear();
10. console.trace()
Prints the stack trace of the code, showing where a specific function was called.
Example:
function firstFunction() {
secondFunction();
}
function secondFunction() {
console.trace("Trace example");
}
firstFunction();
11. Using String Substitutions
You can format output with placeholders.
%s for strings, %d for numbers, %o for objects.
Example:
console.log("Hello, %s!", "World");
console.log("I have %d apples.", 5);
console.log("Object: %o", { a: 1, b: 2 });
12. Styling Logs
CSS can be used to style console messages.
Example:
console.log("%cStyled Text", "color: blue; font-size: 20px; background: yellow;");
13. Custom Debugging
Combine multiple methods to create an effective debugging workflow.
Example:
console.group("Debugging");
console.log("Variable X:", 10);
console.warn("Potential issue with Y.");
console.error("Critical error occurred!");
console.groupEnd();
Conclusion
In conclusion, the console object is far more versatile than just console.log. With its array of methods for categorizing errors, visualizing data, and measuring performance, it can transform debugging into a streamlined and efficient process. Tools like console.table() for clear data representation and console.time() for precise performance tracking are just the tip of the iceberg.
By mastering these features, you can enhance your productivity and make troubleshooting less daunting. So, the next time you're debugging, explore beyond the basics and unlock the full potential of the console object—it’s a game-changer for developers aiming to write better, more efficient code!
Comments