In this section, we’ll dive into how to create a simple binary classification model using Keras. This type of model is useful when you're trying to predict one of two classes (e.g., yes/no, true/false, 0/1). We'll go through the steps of creating the model architecture, compiling it, and setting up the necessary configurations.
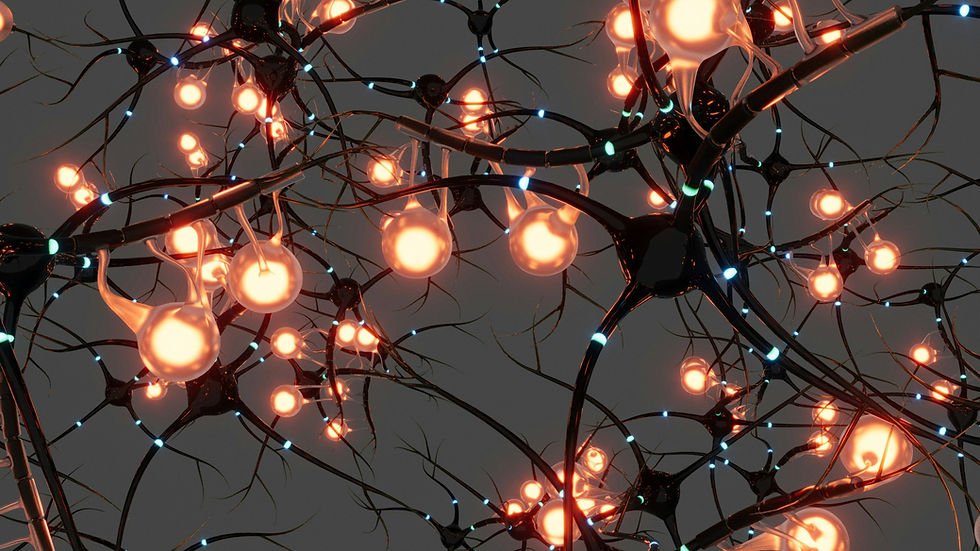
Introduction to Keras and TensorFlow
Keras is a high-level deep learning API that runs on top of TensorFlow, designed to simplify the process of building and training deep learning models. It provides an intuitive interface for defining neural networks and streamlines tasks such as model creation, training, and evaluation. TensorFlow, on the other hand, is a powerful and flexible open-source machine learning framework developed by Google, which serves as the backbone for Keras. TensorFlow offers comprehensive support for various machine learning and deep learning tasks, including natural language processing, computer vision, and reinforcement learning. By combining the ease of Keras with the robustness of TensorFlow, developers can quickly build complex models while leveraging the computational power and scalability that TensorFlow provides. Whether you're a beginner or an expert, the Keras API makes it easier to implement machine learning algorithms without getting lost in the complexities of the underlying TensorFlow framework.
Implementing Binary Classification Model with Keras in Python
To implement a binary classification model with Keras, you start by defining the architecture using a Sequential model. The model consists of an input layer that accepts the feature vector, followed by one or more hidden layers with ReLU activation functions to introduce non-linearity. Finally, the output layer uses a sigmoid activation function, which outputs a probability value between 0 and 1, representing the likelihood of the input belonging to one of the two classes. After defining the model architecture, you compile it with an appropriate optimizer, like RMSprop, and a loss function, such as binary cross-entropy, which is ideal for binary classification tasks.
Step 1: Importing Required Libraries
Before we begin building the model, we need to import TensorFlow and Keras. The code snippet uses keras from TensorFlow, which is a high-level API that simplifies neural network construction.
from tensorflow import keras
from tensorflow.keras import layers
Step 2: Defining the Model Architecture
Now, let’s build the architecture for our binary classification model. This model will have the following components:
Input Layer: We define the shape of the input data. For binary classification, each input can be a vector of features (e.g., numerical data like age, height, etc.).
Hidden Layers: We use two hidden layers, each with 32 neurons and ReLU (Rectified Linear Unit) activation function. ReLU is a common activation function that helps the model learn non-linear relationships in the data.
Output Layer: The output layer contains one neuron with a sigmoid activation function. Sigmoid is ideal for binary classification tasks, as it outputs a value between 0 and 1, representing the probability of belonging to one class.
inputs = keras.Input(shape=(num_input_features,))
x = layers.Dense(32, activation="relu")(inputs)
x = layers.Dense(32, activation="relu")(x)
outputs = layers.Dense(1, activation="sigmoid")(x)
In this code:
num_input_features refers to the number of features in your input data (for example, if you’re predicting whether someone buys a product, num_input_features might include variables like age, income, product price, etc.).
The Dense layer is a fully connected layer where each neuron is connected to every neuron in the previous layer. Here, we use 32 neurons in each of the two hidden layers.
activation="relu" applies the ReLU activation function, which sets any negative input to zero and lets positive inputs pass through.
The output layer uses activation="sigmoid", which is perfect for binary classification as it outputs a probability between 0 and 1.
Step 3: Compiling the Model
After defining the architecture, the next step is to compile the model. In Keras, compiling involves configuring the model for training. Specifically, you need to specify the optimizer and the loss function. For binary classification tasks, the commonly used loss function is binary cross-entropy, and for optimization, we are using RMSprop, which is an adaptive learning rate optimizer.
model = keras.Model(inputs, outputs) model.compile(optimizer="rmsprop", loss="binary_crossentropy")
In this code:
keras.Model(inputs, outputs) creates the Keras model, linking the input and output layers.
optimizer="rmsprop" specifies the optimizer. RMSprop is often used for training deep learning models because it adapts the learning rate during training.
loss="binary_crossentropy" tells the model to use binary cross-entropy as the loss function, which is a good fit for binary classification tasks where the output is 0 or 1.
Step 4: Summary
In summary, this model:
Takes a set of input features (defined by num_input_features).
Passes the inputs through two hidden layers, each with 32 neurons and ReLU activation.
Outputs a single probability value (between 0 and 1) for binary classification using a sigmoid activation.
Example Use Case
This model could be used for various binary classification tasks, such as:
Spam Detection: Classifying whether an email is spam or not.
Customer Churn Prediction: Predicting if a customer will churn or stay.
Disease Diagnosis: Classifying whether a patient has a certain disease or not.
Once you’ve compiled the model, you can train it on your data using the model.fit() function, which will optimize the model parameters (weights) using the training data.
This is a simple and effective way to create a binary classification model in Keras. You can experiment with the number of layers, neurons, activation functions, and other configurations to tune the model for your specific task.
Conclusion
In this blog, we explored the process of building a Binary Classification with Keras in Python, a high-level neural network API within TensorFlow. Binary classification involves predicting one of two possible outcomes, such as yes/no, true/false, or 0/1. We started by importing the necessary libraries, namely TensorFlow and Keras, to facilitate building the model. The architecture of the model was carefully designed with an input layer that accepts feature vectors, followed by two fully connected hidden layers, each containing 32 neurons with ReLU activation. The output layer consists of a single neuron with a sigmoid activation function, ideal for binary classification tasks since it outputs a probability between 0 and 1, representing the likelihood of belonging to one class. After defining the architecture, the model was compiled using the RMSprop optimizer, which adapts the learning rate during training, and the binary cross-entropy loss function, a standard choice for binary classification problems. The blog serves as a comprehensive guide to building a basic binary classification model with Keras, providing insight into the key components, including the choice of activation functions, loss functions, and optimizers, as well as the flexibility Keras offers for adjusting the model for different classification tasks.
Comments