Whether you're a beginner looking to understand basic programming concepts or an experienced developer brushing up on your skills, this guide has something for you.
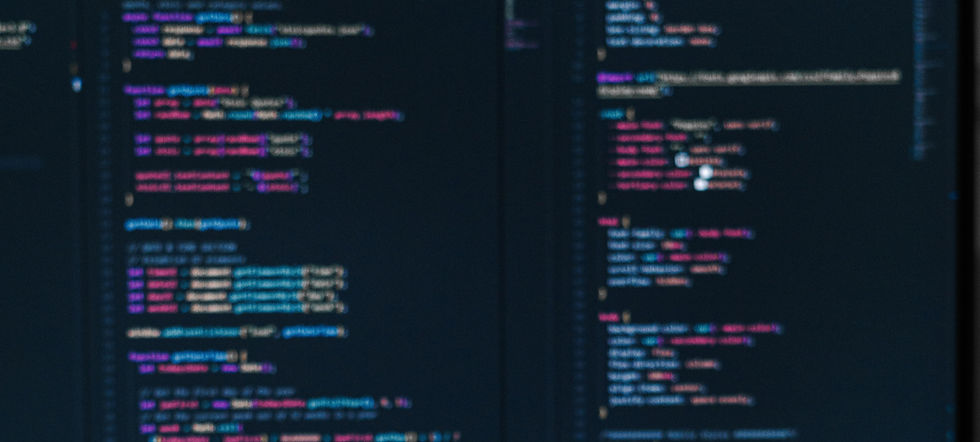
What is a Factorial?
The concept of a factorial is fundamental in mathematics and has a wide range of applications, from combinatorics to probability theory. In this blog post, we’ll delve into what a factorial is and explore different ways to calculate it using Python. A factorial of a non-negative integer nn is the product of all positive integers less than or equal to nn. It is denoted by n!n!. For example:
5!=5×4×3×2×1=120
3!=3×2×1=6
0!=1 (by definition)
Calculating Factorials in Python
Python, with its simplicity and powerful libraries, offers multiple ways to calculate factorials. We'll explore three different methods: iterative, recursive, and using Python’s built-in libraries.
Factorials in Python; Iterative Approach
The iterative approach involves using a loop to multiply the numbers in the sequence. In following script, we initialize result to 1 and then use a for loop to multiply result by each integer up to n.
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
# Example usage
n = 5
print(f"The factorial of {n} is {factorial_iterative(n)}")
Output for above script:
The factorial of 5 is 120
Factorials in Python; Recursive Approach
The recursive approach defines the factorial in terms of itself. It’s a more elegant solution but can be less efficient for large values of n. In following script, the function calls itself with decremented values of n until it reaches 0, at which point it returns 1.
def factorial_recursive(n):
if n == 0:
return 1
else:
return n * factorial_recursive(n - 1)
# Example usage
n = 5
print(f"The factorial of {n} is {factorial_recursive(n)}")
Output for above script:
The factorial of 5 is 120
Factorials in Python; Using Python’s Built-in Libraries
Python’s math library provides a built-in function to calculate factorials, which is highly optimized. This is the simplest and most efficient way to calculate a factorial in Python, leveraging the built-in capabilities of the math library.
import math
# Example usage
n = 5
print(f"The factorial of {n} is {math.factorial(n)}")
Output for above script:
The factorial of 5 is 120
Comparing the Methods for Calculating Factorials in Python
Each method has its own strengths:
Iterative Approach: Simple and efficient for most use cases.
Recursive Approach: Elegant and easy to understand, but can be inefficient for large values of nn due to deep recursion.
Built-in Libraries: The most efficient and recommended method for practical purposes.
In conclusion, understanding how to calculate factorials is a fundamental skill in programming and mathematics. Python offers several ways to compute factorials, from simple iterative loops to elegant recursive functions and efficient built-in methods. Each method has its own use cases and can be chosen based on the problem at hand.
By exploring these different approaches, you can deepen your understanding of Python programming and the mathematical concept of factorials. Happy coding!
Comments