MNIST dataset in tensorflow consists of a large collection of handwritten digits and serves as a benchmark for evaluating various machine learning models. In this blog, we’ll explore the MNIST dataset and demonstrate how to build a simple digit classification model using TensorFlow in python, one of the most powerful deep learning frameworks.
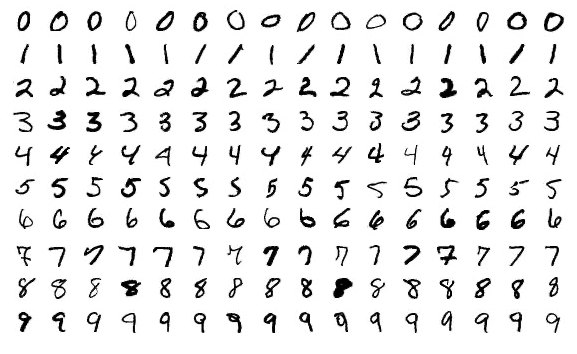
Classification of MNIST Dataset with TensorFlow
Classification of the MNIST dataset with TensorFlow in Python involves a systematic approach to building a machine learning model that can accurately identify handwritten digits. The process begins by loading the MNIST dataset, which contains 70,000 grayscale images of digits ranging from 0 to 9, each represented as a 28x28 pixel grid. In TensorFlow, this dataset is easily accessible through the tf.keras.datasets.mnist module. Once loaded, the dataset is typically split into training and testing sets, with the training set used to train the model and the testing set used to evaluate its performance. The pixel values of the images are normalized to be between 0 and 1 to improve the efficiency and convergence of the model during training. The model itself is usually a simple neural network built using TensorFlow’s Keras API. A common architecture involves a sequential model with a flattened input layer (to convert the 28x28 grid into a 784-element vector), one or more dense hidden layers with ReLU activation functions, and an output layer with a softmax activation function that produces probabilities for each of the 10 digit classes. The model is compiled with an optimizer like Adam and a loss function such as sparse categorical crossentropy, which is well-suited for multi-class classification problems. Training the model involves passing the training data through the network for a set number of epochs, during which the model’s weights are adjusted to minimize the loss function. After training, the model is evaluated on the test set to determine its accuracy. Finally, the trained model can be used to make predictions on new data, demonstrating its ability to generalize from the training examples to unseen images. This process not only showcases the power of TensorFlow in handling image classification tasks but also provides a foundation for more complex applications in deep learning.
What is MNIST Dataset?
The MNIST dataset, an acronym for Modified National Institute of Standards and Technology, is one of the most famous datasets in the realm of machine learning. It's often considered the "Hello World" of machine learning because of its simplicity and the wealth of resources available to work with it. If you’re just starting out with machine learning or deep learning, this dataset is an excellent place to begin. In this guide, we’ll explore what the MNIST dataset is, why it’s important, and how to work with it using TensorFlow, a popular deep learning framework.
The MNIST dataset is a collection of 70,000 images of handwritten digits from 0 to 9. Each image is 28x28 pixels, which means each image contains 784 pixels. The images are in grayscale, and each pixel has a value between 0 and 255, representing the intensity of the color (0 is black, and 255 is white).
The dataset is split into two parts:
Training Set: 60,000 images used to train the model.
Test Set: 10,000 images used to test the model’s accuracy.
The MNIST dataset is crucial for several reasons:
Ease of Access: It’s readily available in most machine learning frameworks, including TensorFlow.
Simplicity: The dataset is small and manageable, making it ideal for learning and experimentation.
Benchmarking: Since it’s a widely-used dataset, it’s often used to benchmark new machine learning models.
Community Support: Given its popularity, there’s a vast amount of community support, including tutorials, forums, and pre-trained models.
Implementing Deep Learning Neural Networks over MNIST Dataset
Implementing deep learning neural networks over the MNIST dataset involves creating a model with multiple layers, including input, hidden, and output layers, to classify handwritten digits. TensorFlow's Keras API simplifies this process, allowing efficient model building, training, and evaluation. This approach demonstrates the capability of neural networks in handling image classification tasks with high accuracy.
Loading the MNIST Dataset with TensorFlow
TensorFlow, an open-source machine learning library, provides easy access to the MNIST dataset through its tf.keras.datasets module. Here’s how you can load and prepare the dataset:
import tensorflow as tf
# Load the MNIST dataset
mnist = tf.keras.datasets.mnist
# Split into training and testing data
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize the pixel values to be between 0 and 1
x_train, x_test = x_train / 255.0, x_test / 255.0
Visualizing MNIST Dataset
Before diving into model training, it’s helpful to visualize some of the images in the dataset to get a sense of what you’re working with.
import matplotlib.pyplot as plt
# Display the first 10 images from the training dataset
for i in range(10):
plt.subplot(2, 5, i+1)
plt.imshow(x_train[i], cmap='gray')
plt.title(f"Label: {y_train[i]}")
plt.axis('off')
plt.show()
Output for the above code:
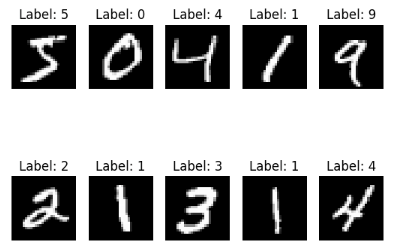
Building a Simple Neural Network with TensorFlow
After loading and visualizing the data, you can build a neural network model to classify the handwritten digits. Here’s a simple model using TensorFlow’s Keras API:
# Build the model
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)), # Flatten the input
tf.keras.layers.Dense(128, activation='relu'), # First hidden layer
tf.keras.layers.Dense(64, activation='relu'), # Second hidden layer
tf.keras.layers.Dense(10, activation='softmax') # Output layer
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Training Neural Network Model
Next, you’ll train the model using the training data. This process adjusts the weights of the neural network to minimize the loss function, improving the model’s accuracy.
# Train the model
model.fit(x_train, y_train, epochs=5)
Output for the above code:
Epoch 1/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 10s 4ms/step - accuracy: 0.8792 - loss: 0.4182
Epoch 2/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 6s 3ms/step - accuracy: 0.9676 - loss: 0.1046
Epoch 3/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 10s 3ms/step - accuracy: 0.9781 - loss: 0.0709
Epoch 4/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 8s 4ms/step - accuracy: 0.9836 - loss: 0.0515
Epoch 5/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 6s 3ms/step - accuracy: 0.9870 - loss: 0.0392
Evaluating Neural Network Model
After training, it’s essential to evaluate how well the model performs on unseen data. This is where the test set comes into play.
# Evaluate the model
test_loss, test_acc = model.evaluate(x_test, y_test)
print('\nTest accuracy:', test_acc)
Output for the above code:
313/313 ━━━━━━━━━━━━━━━━━━━━ 1s 1ms/step - accuracy: 0.9712 - loss: 0.1001
Test accuracy: 0.9751999974250793
Making Predictions
Finally, you can use the trained model to make predictions on new images. Here’s how to predict the label of the first test image:
# Make predictions
predictions = model.predict(x_test)
# Print some predictions
for i in range(5):
print('Predicted value: ', predictions[i].argmax())
Output for the above code:
313/313 ━━━━━━━━━━━━━━━━━━━━ 1s 2ms/step
Predicted value: 7
Predicted value: 2
Predicted value: 1
Predicted value: 0
Predicted value: 4
Full Code for Classification of MNIST Dataset with TensorFlow in Python
The full code for classifying the MNIST dataset with TensorFlow in Python includes loading and preprocessing the data, building a neural network model using TensorFlow's Keras API, training the model, and evaluating its performance on test data. This end-to-end implementation demonstrates the process of creating an effective digit classification system. The code is concise and accessible, making it an excellent starting point for understanding deep learning.
# Import libraries
import tensorflow as tf
import matplotlib.pyplot as plt
# Load the MNIST dataset
mnist = tf.keras.datasets.mnist
# Split into training and testing data
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize the pixel values to be between 0 and 1
x_train, x_test = x_train / 255.0, x_test / 255.0
# Display the first 10 images from the training dataset
for i in range(10):
plt.subplot(2, 5, i+1)
plt.imshow(x_train[i], cmap='gray')
plt.title(f"Label: {y_train[i]}")
plt.axis('off')
plt.show()
# Build the model
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)), # Flatten the input
tf.keras.layers.Dense(128, activation='relu'), # First hidden layer
tf.keras.layers.Dense(64, activation='relu'), # Second hidden layer
tf.keras.layers.Dense(10, activation='softmax') # Output layer
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=5)
# Evaluate the model
test_loss, test_acc = model.evaluate(x_test, y_test)
print('\nTest accuracy:', test_acc)
# Make predictions
predictions = model.predict(x_test)
# Print some predictions
for i in range(5):
print('Predicted value: ', predictions[i].argmax())
Comments