JavaScript is a versatile and dynamic programming language used extensively in web development. Understanding its data types is essential for writing efficient and error-free code. This guide will walk you through the different types of data in JavaScript, how they work, and examples of their usage.
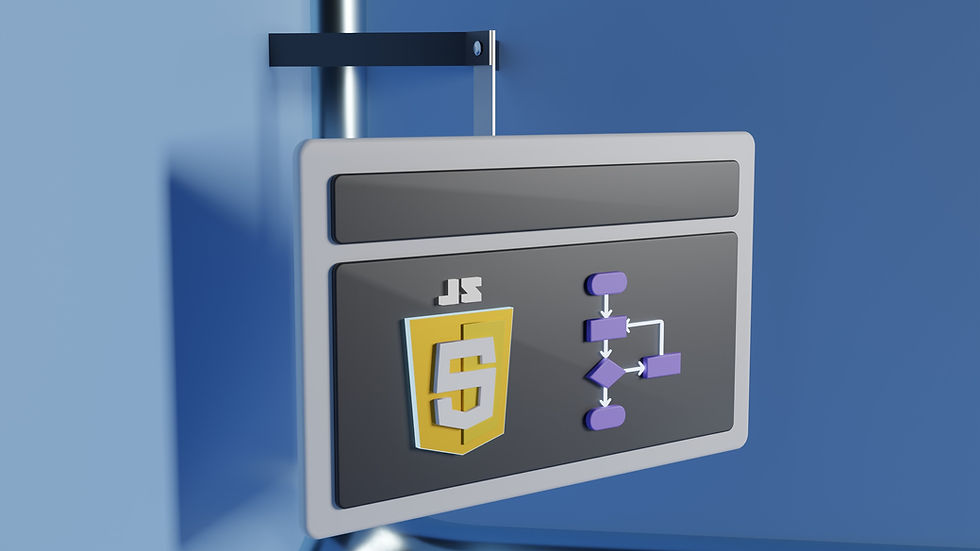
What are Data Types in Javascript?
Data types in JavaScript represent the kind of data that a variable can hold, such as numbers, strings, objects, or booleans. They are essential because they help define how the data can be used and interacted with in a program. For instance, numbers are used for mathematical operations, while strings handle text. JavaScript has two main categories of data types: primitive types (like Number, String, Boolean, Null, Undefined, Symbol, and BigInt) and reference types (like Objects, Arrays, and Functions). Understanding data types is crucial for writing robust, error-free code, as it allows developers to manipulate values correctly and predictably, avoid type-related bugs, and optimize memory usage. Additionally, JavaScript's dynamic typing means variables can hold any data type, making it even more important for programmers to manage data types consciously.
1. Primitive Data Types
Primitive data types in JavaScript are the basic building blocks of data and represent single, immutable values. They include Number, used for numeric values like integers and floating-point numbers; String, for sequences of characters; Boolean, representing true or false values; Undefined, which signifies a variable declared but not assigned a value; Null, a deliberate assignment of "no value"; Symbol, which represents unique, immutable identifiers; and BigInt, used for handling integers larger than the safe limit for the Number type. These data types are called "primitive" because they are not objects and are directly operated upon. They are stored by value, meaning each variable holds its own copy of the data. Understanding primitive types is essential for developers to ensure accurate data handling, memory management, and type-specific operations in their programs.
a. Number
The Number type is used for numeric values, both integers and floating-point numbers.
let age = 25; // Integer
let price = 99.99; // Floating-point number
Special numeric values include:
Infinity
-Infinity
NaN (Not a Number)
Example:
console.log(10 / 0); // Infinity
console.log(-10 / 0); // -Infinity
console.log("hello" * 2); // NaN
b. String
The String type represents a sequence of characters. Strings can be enclosed in single ('), double ("), or backticks (``).
let firstName = 'John';
let lastName = "Doe";
let message = `Hello, ${firstName}!`;
c. Boolean
The Boolean type represents logical values: true or false.
let isLoggedIn = true;
let isAdmin = false;
d. Undefined
A variable is undefined when it has been declared but not assigned a value.
let user;
console.log(user); // undefined
e. Null
Null represents an explicitly empty or non-existent value.
let selectedColor = null;
f. Symbol (ES6)
The Symbol type is used to create unique identifiers for objects.
let uniqueId = Symbol('id');
let anotherId = Symbol('id');
console.log(uniqueId === anotherId); // false
g. BigInt (ES11)
The BigInt type is used for integers that are too large to be represented by the Number type.
let bigNumber = 1234567890123456789012345678901234567890n;
console.log(bigNumber + 10n); // 1234567890123456789012345678901234567900n
2. Non-Primitive (Reference) Data Types
Non-primitive or reference data types in JavaScript are more complex data structures used to store collections of data or more detailed information. Unlike primitive types, which store a single value, reference types hold a reference to the memory location where the data is stored. Common examples include Objects, Arrays, and Functions. Objects are versatile containers that store key-value pairs, while arrays are ordered lists of elements, and functions are reusable blocks of code. These data types are mutable, meaning their contents can be changed without changing the reference itself. Understanding non-primitive data types is essential for tasks like handling dynamic datasets, creating reusable components, and structuring applications effectively. They play a crucial role in JavaScript's object-oriented capabilities and enable developers to solve complex problems with greater flexibility.
a. Object
Objects are collections of key-value pairs.
let user = {
name: 'Alice',
age: 30
};
console.log(user.name); // Alice
b. Array
Arrays are ordered collections of values.
let colors = ['red', 'green', 'blue'];
console.log(colors[0]); // red
c. Function
Functions are first-class objects in JavaScript.
function greet() {
console.log('Hello!');
}
greet();
d. Date
The Date object represents dates and times.
let now = new Date();
console.log(now); // Current date and time
e. Set and Map
Set: A collection of unique values.
let set = new Set([1, 2, 2, 3]);
console.log(set); // Set { 1, 2, 3 }
Map: A collection of key-value pairs.
let map = new Map();
map.set('name', 'John');
console.log(map.get('name')); // John
Type Checking in Javascript
Type checking in JavaScript refers to the process of verifying the data type of a value to ensure it behaves as expected in a program. JavaScript is dynamically typed, meaning variables can hold values of any type without strict declarations. However, this flexibility can lead to unexpected errors if types are not properly managed.
For basic type checking, JavaScript provides the typeof operator, which returns a string indicating the type of a value (e.g., "string", "number", "object"). The instanceof operator is also used to check whether an object is an instance of a specific class or constructor. For more robust type checking, especially in large projects, developers use tools like TypeScript, a superset of JavaScript that enforces static typing during development. Proper type checking helps prevent type-related bugs, improves code readability, and ensures smoother collaboration in larger teams by clearly defining the expected data types.
a. Using typeof
The typeof operator returns the type of a value.
console.log(typeof 42); // "number"
console.log(typeof 'hello'); // "string"
console.log(typeof true); // "boolean"
console.log(typeof undefined); // "undefined"
console.log(typeof null); // "object" (quirk in JavaScript)
console.log(typeof Symbol()); // "symbol"
console.log(typeof {}); // "object"
console.log(typeof []); // "object"
b. Using instanceof
The instanceof operator checks if an object is an instance of a particular class.
console.log([] instanceof Array); // true
console.log({} instanceof Object); // true
Dynamic Typing in JavaScript
JavaScript is dynamically typed, meaning variables can hold values of any type and can change type during runtime.
let value = 42; // number
value = 'hello'; // string
value = true; // boolean
console.log(value); // true
Type Conversion
Type conversion in JavaScript refers to the process of converting a value from one data type to another. It can occur explicitly (when the programmer intentionally converts the type) or implicitly (when JavaScript automatically converts types during operations). Explicit type conversion is achieved using methods like String(), Number(), or Boolean(), allowing developers to control how values are cast. For example, converting "123" (a string) to a number can be done using Number("123").
Implicit type conversion, also called type coercion, happens when JavaScript converts types during operations like concatenation or comparisons. For instance, when adding a string and a number ("5" + 3), JavaScript coerces the number into a string, resulting in "53". Similarly, in comparisons, == performs type coercion, while === avoids it by enforcing strict equality. Understanding type conversion is vital for debugging unexpected behavior and writing predictable, efficient code.
a. Implicit Conversion (Type Coercion)
JavaScript automatically converts data types in certain operations.
console.log('5' + 10); // "510" (string concatenation)
console.log('5' - 2); // 3 (string to number)
b. Explicit Conversion
Use global functions like Number(), String(), and Boolean() for explicit conversion.
console.log(Number('42')); // 42
console.log(String(42)); // "42"
console.log(Boolean(0)); // false
Conclusion
Data types form the backbone of any programming language, and JavaScript is no exception. They dictate how values are stored, manipulated, and interpreted by the program. Mastering JavaScript’s data types not only enhances code readability and maintainability but also minimizes bugs and unexpected behavior. By understanding the nuances of both primitive and non-primitive types, you can take full advantage of JavaScript's flexibility and power. As JavaScript evolves, features like BigInt and Symbol highlight the language's capability to handle complex programming needs. Whether you're working on a small script or a large-scale application, a solid grasp of data types ensures you can build reliable and efficient solutions.
Comments