Decision trees are a powerful and intuitive method for both classification and regression tasks in machine learning. They are used widely due to their simplicity and interpretability. In this blog, we will explore the fundamentals of decision trees, their advantages and disadvantages, and how to implement them in Python using popular libraries like scikit-learn.
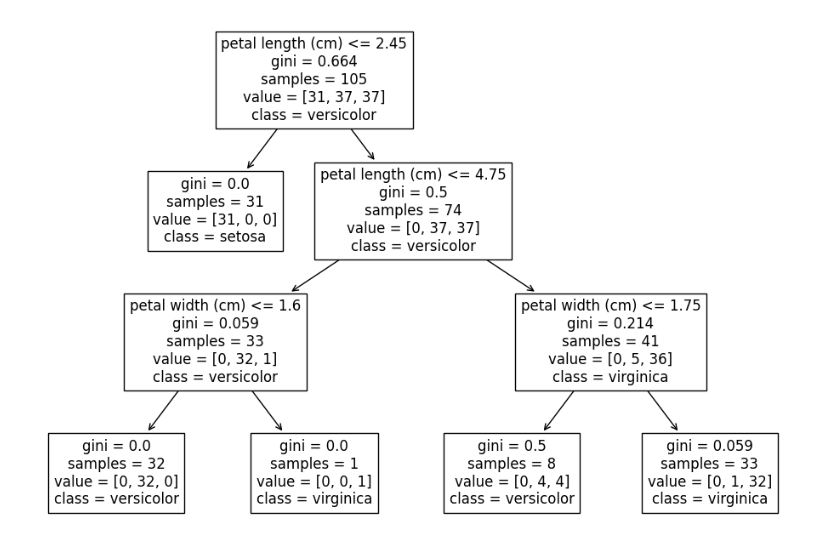
What is a Decision Tree?
A decision tree is a flowchart-like structure where each internal node represents a decision based on a feature, each branch represents the outcome of the decision, and each leaf node represents a final output (label). The tree starts from a root node and splits into branches, eventually leading to a decision at the leaf nodes.
In classification tasks, the decision tree assigns the class label at each leaf node, while in regression tasks, it predicts a continuous value. The goal of a decision tree is to create a model that predicts the value of a target variable by learning simple decision rules inferred from the data features.
How Does a Decision Tree Work?
Decision trees are a supervised machine learning algorithm that operates like a flowchart to make predictions or decisions. They start with a root node, branching into internal nodes representing decisions based on data attributes. These decisions lead to further branches until leaf nodes are reached, which provide the final outcome or prediction. The tree is constructed by selecting the best attribute at each node to split the data, aiming to create purer subsets. This process continues recursively until a stopping criterion is met, such as reaching a maximum depth or pure nodes. Decision trees are popular due to their interpretability and ability to handle both classification and regression tasks.
Feature Selection: The process begins by selecting the best feature to split the data. The selection is based on a criterion that measures the quality of the split, such as Gini impurity, information gain (entropy), or mean squared error (for regression).
Splitting: The selected feature is used to split the dataset into subsets, which are then recursively split further based on other features. This process continues until a stopping criterion is met, such as a maximum depth of the tree or a minimum number of samples per leaf.
Decision Making: Once the tree is built, it can be used to make predictions. For a given input, the decision tree follows the decisions in the nodes, based on the feature values, until it reaches a leaf node, where the final prediction is made.
Advantages and Disadvantages of Decision Trees
Decision trees offer several advantages, including ease of interpretation, the ability to handle both numerical and categorical data, and their capacity to capture non-linear relationships. However, they also have drawbacks. Decision trees are prone to overfitting, meaning they can become too complex and perform poorly on new data. Additionally, they can be unstable, as small changes in the data can lead to significantly different trees. To mitigate these issues, techniques like pruning and ensemble methods are often employed.
Advantages of Decision Trees:
Easy to Interpret: Decision trees are easy to understand and visualize, making them a good choice for explaining model predictions.
No Need for Feature Scaling: Decision trees do not require feature scaling or normalization.
Handles Both Numerical and Categorical Data: Decision trees can handle a mix of both numerical and categorical features.
Non-parametric: They do not assume any underlying distribution of the data.
Disadvantages of Decision Trees:
Overfitting: Decision trees can easily overfit, especially if they are too deep. This can be mitigated through pruning or setting constraints like maximum depth.
Instability: Small changes in the data can result in a completely different tree, making them sensitive to data variations.
Bias: Decision trees can be biased towards features with more levels. Techniques like Random Forests can help overcome this issue.
Implementing Decision Trees in Python
Implementing decision trees in Python is straightforward using libraries like Scikit-learn. You start by importing necessary libraries and loading your dataset. Then, split the data into training and testing sets. The core step involves creating a decision tree model using algorithms like ID3, C4.5, or CART, which are available in Scikit-learn. Once trained, the model can make predictions on the test set. To evaluate performance, metrics like accuracy, precision, recall,and F1-score are commonly used. Additionally, visualizing the decision tree can provide insights into the model's decision-making process. Let's dive into implementing decision trees using Python and the scikit-learn library. We'll use the Iris dataset as an example for a classification task.
1. Loading Iris Dataset from sklearn
First, we need to load the Iris dataset, which is conveniently available in scikit-learn.
from sklearn.datasets import load_iris
import pandas as pd
# Load the iris dataset
iris = load_iris()
df = pd.DataFrame(data=iris.data, columns=iris.feature_names)
df['species'] = iris.target
2. Splitting the Dataset
Next, we split the data into training and testing sets.
from sklearn.model_selection import train_test_split
X = df.drop('species', axis=1)
y = df['species']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
3. Training the Decision Tree Model
We can now train a decision tree classifier using the training data.
from sklearn.tree import DecisionTreeClassifier
# Initialize the model
tree = DecisionTreeClassifier(criterion='gini', max_depth=3, random_state=42)
# Train the model
tree.fit(X_train, y_train)
4. Visualizing the Decision Tree
scikit-learn provides a convenient way to visualize decision trees using plot_tree.
from sklearn.tree import plot_tree
import matplotlib.pyplot as plt
plt.figure(figsize=(12, 8))
plot_tree(tree, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
Output of the code above:
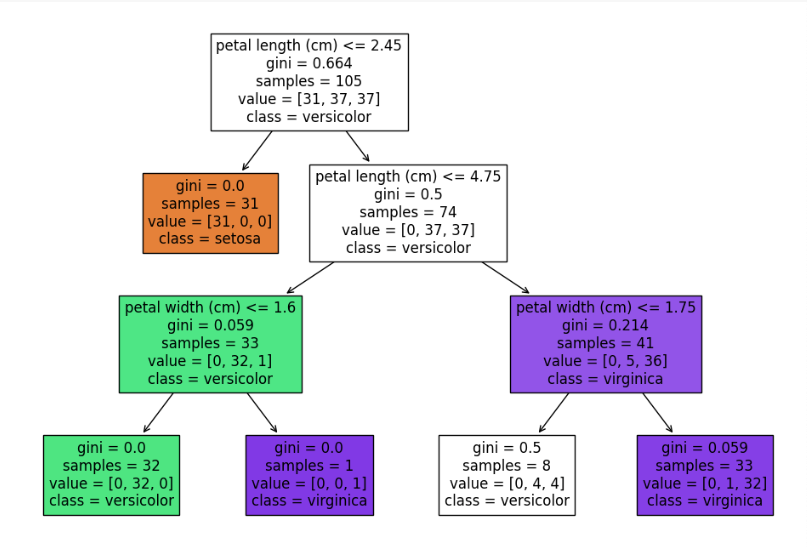
5. Evaluating the Decision Tree Model
Finally, we evaluate the model's performance on the test set.
from sklearn.metrics import accuracy_score
# Predict the species for the test set
y_pred = tree.predict(X_test)
# Calculate the accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In conclusion, decision trees are a fundamental and versatile tool in the machine learning toolkit, offering a straightforward approach to classification and regression problems. They stand out for their interpretability, as they provide clear, visual representations of decision-making processes. While they do not require feature scaling and can handle both numerical and categorical data, decision trees can easily overfit, especially if they become too complex. Techniques such as pruning, setting a maximum depth, or using ensemble methods like Random Forests can mitigate this issue and enhance performance.
In this blog, we've covered the basics of decision trees, their advantages and disadvantages, and how to implement them in Python using the scikit-learn library. As you continue to explore decision trees, consider experimenting with different datasets and tuning parameters to see how they affect the model's performance.
Comments