Deep Learning in Python: Unleashing the Power of Neural Networks
- Samuel Black
- Aug 6, 2024
- 7 min read
Deep learning, a subset of machine learning, has rapidly evolved into a cornerstone of artificial intelligence (AI). It enables machines to learn from vast amounts of data, uncover complex patterns, and make predictions with remarkable accuracy. Python, with its simplicity and extensive library ecosystem, has become the go-to language for deep learning development. In this blog, we will explore the landscape of deep learning in Python, covering key libraries, fundamental concepts, and practical applications to help you harness the power of neural networks.
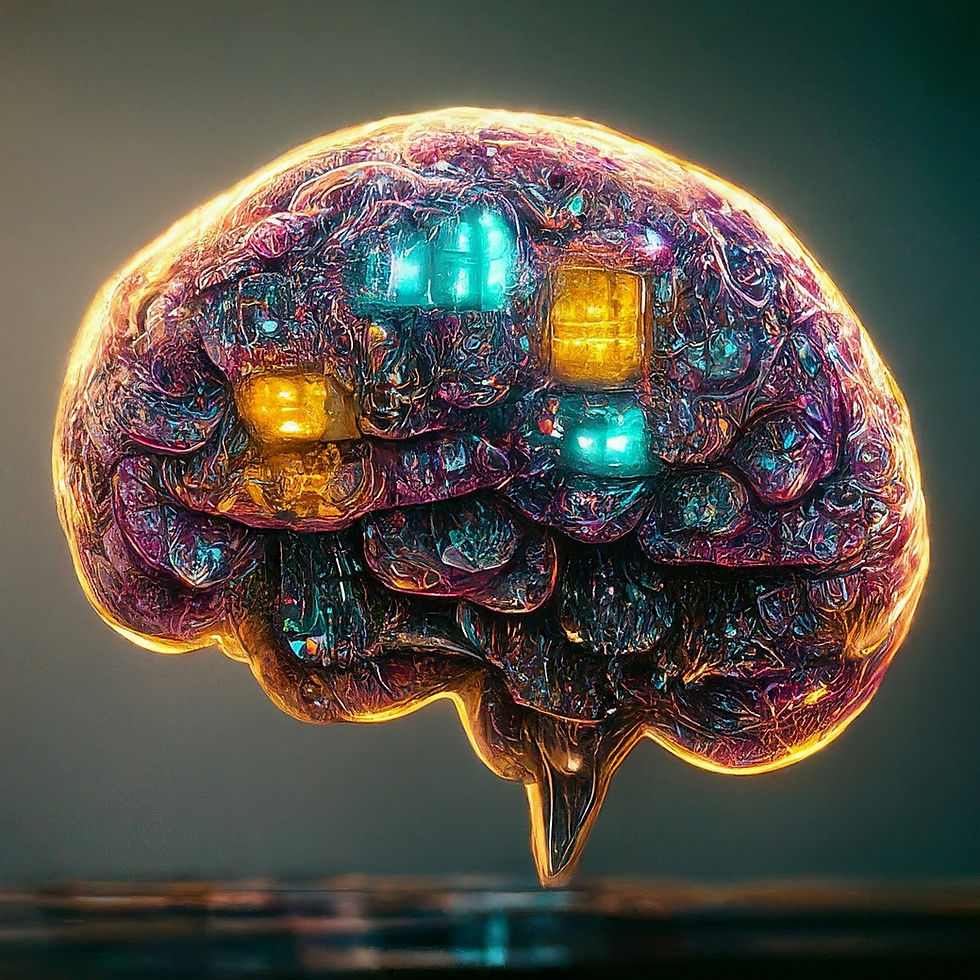
Why Python for Deep Learning?
Python has emerged as the leading language for deep learning due to its simplicity, readability, and the powerful ecosystem of libraries and frameworks it offers. Its straightforward syntax makes it accessible for beginners, while its versatility caters to the needs of seasoned developers. Python's extensive libraries, such as TensorFlow, Keras, and PyTorch, provide comprehensive tools for building, training, and deploying deep learning models, supporting everything from quick prototyping to large-scale production systems. The language also boasts seamless integration with other key data science tools, like NumPy for numerical computations and Pandas for data manipulation, facilitating a smooth workflow from data preprocessing to model evaluation. Moreover, Python's active and supportive community contributes to a wealth of resources, tutorials, and third-party libraries, continuously driving innovation and improving accessibility. This combination of ease of use, powerful capabilities, and community support makes Python the preferred choice for deep learning, enabling rapid development and deployment of sophisticated AI models. Python's popularity in the AI community is no accident. Several factors make it an ideal choice for deep learning:
Ease of Learning and Use: Python's simple syntax and readability make it accessible to both beginners and experienced developers. This ease of use allows for quick prototyping and experimentation.
Extensive Library Ecosystem: Python boasts a rich set of libraries and frameworks that simplify deep learning tasks. These include TensorFlow, Keras, PyTorch, and others, each offering unique features and capabilities.
Active Community and Support: The Python community is vibrant and supportive, with extensive documentation, tutorials, and forums available to help developers at all levels.
Integration with Other Technologies: Python seamlessly integrates with other data science and machine learning tools, such as NumPy, Pandas, and scikit-learn, enabling comprehensive data analysis and model development workflows.
Key Libraries for Deep Learning in Python
1. TensorFlow
TensorFlow, developed by Google, is one of the most popular deep learning frameworks. It provides a comprehensive ecosystem for building and deploying machine learning models. TensorFlow's flexible architecture allows for easy deployment across various platforms, including desktops, mobile devices, and cloud environments. It supports both high-level APIs, like Keras, and low-level operations for fine-grained control over neural network construction.
2. Keras
Keras is a high-level neural networks API that runs on top of TensorFlow (and previously Theano). It is designed to enable fast experimentation and ease of use. Keras provides a simple and consistent interface for building and training deep learning models, making it an excellent choice for beginners and rapid prototyping.
3. PyTorch
PyTorch, developed by Facebook's AI Research lab, has gained popularity for its dynamic computational graph and ease of debugging. PyTorch's intuitive design allows for flexible and efficient model building, making it a favorite among researchers and practitioners. Its ability to seamlessly integrate with Python's ecosystem and native support for GPU acceleration further enhance its appeal.
4. Theano
Theano, although not as widely used today as TensorFlow or PyTorch, was one of the first libraries to provide efficient numerical computation and deep learning capabilities. It laid the groundwork for many subsequent frameworks and remains a valuable tool for those interested in understanding the fundamentals of deep learning.
Getting Started with Deep Learning in Python
Installing Essential Libraries
Before diving into deep learning, you'll need to install the necessary libraries. For example, to set up TensorFlow and Keras, you can use the following commands:
pip install tensorflow
pip install keras
For PyTorch, the installation command depends on your operating system and hardware configuration. You can find the appropriate command on the official PyTorch website.
Building a Simple Neural Network with Keras
Let's walk through a basic example of building a neural network using Keras. We'll create a simple model to classify the famous MNIST dataset of handwritten digits.
import keras
from keras.models import Sequential
from keras.layers import Dense
from keras.datasets import mnist
from keras import utils
# Load and preprocess the MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train = X_train.reshape(X_train.shape[0], 784).astype('float32') / 255
X_test = X_test.reshape(X_test.shape[0], 784).astype('float32') / 255
y_train = utils.to_categorical(y_train)
y_test = utils.to_categorical(y_test)
# Define the model
model = Sequential()
model.add(Dense(512, input_shape=(784,), activation='relu'))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=128, validation_data=(X_test, y_test))
# Evaluate the model
score = model.evaluate(X_test, y_test, verbose=0)
print(f'Test accuracy: {score[1]:.4f}')
Output for the above code:
Epoch 1/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 11s 18ms/step - accuracy: 0.8725 - loss: 0.4589 - val_accuracy: 0.9550 - val_loss: 0.1518
Epoch 2/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 7s 14ms/step - accuracy: 0.9646 - loss: 0.1217 - val_accuracy: 0.9700 - val_loss: 0.0993
Epoch 3/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 6s 12ms/step - accuracy: 0.9785 - loss: 0.0746 - val_accuracy: 0.9748 - val_loss: 0.0765
Epoch 4/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 11s 13ms/step - accuracy: 0.9851 - loss: 0.0519 - val_accuracy: 0.9798 - val_loss: 0.0680
Epoch 5/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 10s 12ms/step - accuracy: 0.9899 - loss: 0.0364 - val_accuracy: 0.9793 - val_loss: 0.0657
Epoch 6/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 7s 15ms/step - accuracy: 0.9930 - loss: 0.0266 - val_accuracy: 0.9803 - val_loss: 0.0572
Epoch 7/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 9s 13ms/step - accuracy: 0.9946 - loss: 0.0210 - val_accuracy: 0.9805 - val_loss: 0.0601
Epoch 8/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 10s 12ms/step - accuracy: 0.9963 - loss: 0.0154 - val_accuracy: 0.9821 - val_loss: 0.0589
Epoch 9/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 11s 14ms/step - accuracy: 0.9972 - loss: 0.0116 - val_accuracy: 0.9812 - val_loss: 0.0582
Epoch 10/10
469/469 ━━━━━━━━━━━━━━━━━━━━ 11s 16ms/step - accuracy: 0.9977 - loss: 0.0093 - val_accuracy: 0.9826 - val_loss: 0.0573
In this example, we:
Loaded the MNIST dataset and preprocessed it by normalizing the pixel values and converting the labels to one-hot encoding.
Defined a simple feedforward neural network with one hidden layer using the Sequential model API.
Compiled the model with categorical cross-entropy as the loss function and Adam as the optimizer.
Trained the model for 10 epochs and evaluated its accuracy on the test set.
Advanced Deep Learning Techniques
While the above example demonstrates a basic neural network, deep learning in Python encompasses much more. You can explore advanced architectures such as Convolutional Neural Networks (CNNs) for image recognition, Recurrent Neural Networks (RNNs) for sequence modeling, and Generative Adversarial Networks (GANs) for data generation. As deep learning continues to evolve, so do the techniques and architectures that push the boundaries of what AI can achieve. Advanced deep learning techniques include a variety of sophisticated models designed to handle specific tasks with high efficiency and accuracy. Convolutional Neural Networks (CNNs) are tailored for image and video analysis, using convolutional layers to automatically detect patterns such as edges, textures, and objects. Recurrent Neural Networks (RNNs), including their variants like Long Short-Term Memory (LSTM) and Gated Recurrent Units (GRUs), excel in processing sequential data, making them ideal for tasks like time series forecasting and natural language processing. Generative Adversarial Networks (GANs) consist of two competing networks—the generator and the discriminator—that work together to create realistic synthetic data, enabling applications in art, music, and more. Transformers, known for their use in NLP, have revolutionized tasks involving long-range dependencies in data, with models like BERT and GPT achieving state-of-the-art results in text understanding and generation. These advanced techniques expand the capabilities of deep learning, allowing for more accurate predictions, innovative applications, and new discoveries across various domains.
Real-World Applications of Deep Learning Using Python
Deep learning, powered by Python's versatile libraries, has permeated a wide range of industries, driving innovation and solving complex problems.
In computer vision, deep learning models like Convolutional Neural Networks (CNNs) are employed in image classification, object detection, and facial recognition. Python frameworks such as TensorFlow and PyTorch enable the development of applications that can automatically tag photos, identify anomalies in medical imaging, or enhance security through facial recognition systems.
In the realm of natural language processing (NLP), deep learning techniques have revolutionized tasks such as language translation, sentiment analysis, and chatbot development. Python's deep learning tools facilitate the creation of models like BERT and GPT, which understand and generate human-like text. These applications power virtual assistants, automate customer service interactions, and provide insights through text analytics.
The healthcare sector benefits immensely from deep learning, with applications ranging from diagnostic imaging to personalized medicine. Python-based models analyze medical scans to detect conditions such as cancer with high accuracy, predict patient outcomes, and assist in the discovery of new drugs by simulating molecular interactions.
In finance, deep learning models are used for predictive analytics, risk assessment, and fraud detection. Python's deep learning frameworks enable the processing of large financial datasets to identify market trends, automate trading strategies, and detect unusual transaction patterns that may indicate fraud.
Autonomous vehicles rely heavily on deep learning for navigating complex environments. Python-based systems process data from sensors like cameras and lidar to recognize objects, predict their movements, and make real-time decisions. This technology is critical for developing self-driving cars and drones that can safely operate in dynamic settings.
In entertainment, deep learning is used to create personalized recommendations on streaming platforms, generate realistic animations, and even compose music. Generative Adversarial Networks (GANs) and other advanced models enable these creative applications, enhancing user experiences and content generation.
These real-world applications highlight the transformative impact of deep learning using Python. By leveraging powerful libraries and frameworks, developers can build sophisticated AI systems that improve efficiency, accuracy, and innovation across diverse fields. As deep learning continues to advance, its applications will only expand, offering new solutions and capabilities in an ever-growing array of domains.
In conclusion, deep learning, facilitated by Python's robust ecosystem, is at the forefront of numerous technological advancements and real-world applications. From revolutionizing healthcare diagnostics and enabling self-driving cars to transforming entertainment and financial industries, the impact of deep learning is profound and far-reaching. Python, with its simplicity, powerful libraries like TensorFlow, Keras, and PyTorch, and an active community, has become the language of choice for developing deep learning models. As the field continues to evolve, staying abreast of the latest techniques and innovations will be crucial for leveraging these technologies to their fullest potential. Whether you're a seasoned practitioner or a newcomer, deep learning in Python offers boundless opportunities to innovate, solve complex problems, and contribute to the cutting-edge developments in artificial intelligence. The future of deep learning is bright, promising even greater advancements and applications across diverse domains.
As you continue your journey in deep learning, remember that the field is constantly evolving. Staying updated with the latest research, tools, and best practices will ensure that you remain at the forefront of this transformative technology.
Comments