In the world of programming, errors are inevitable. Whether it’s a simple typo, an unexpected input, or an issue with an external resource, exceptions can disrupt the normal flow of a program. Python offers robust mechanisms for managing these situations through exception handling. In this blog, we’ll explore what exceptions are, how to handle them in Python, and best practices to follow for effective error management.
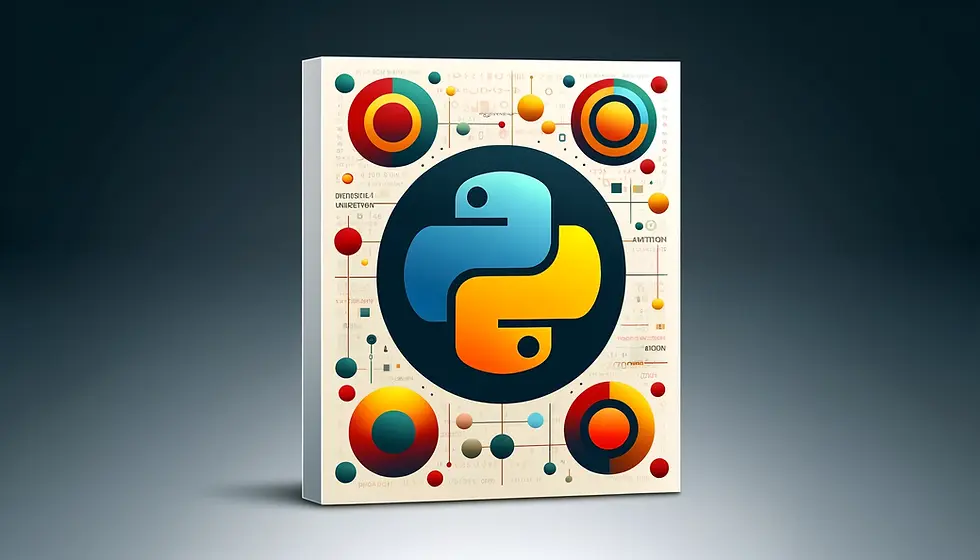
What Are Exceptions in Python?
Exceptions in Python are events that occur during the execution of a program that disrupt the normal flow of instructions. They are typically raised when the interpreter encounters an error, such as trying to divide by zero, accessing a non-existent file, or performing an operation on incompatible types.
When an exception occurs, Python creates an exception object that contains information about the error, such as its type and a traceback that shows where the error occurred in the code. If these exceptions are not handled, they will lead to program crashes.
An exception is an event that occurs during the execution of a program that disrupts its normal flow. In Python, exceptions can be raised for various reasons, such as:
Attempting to divide by zero
Accessing an invalid index in a list
Trying to open a file that doesn’t exist
When an exception occurs, Python raises an error and halts the execution unless the error is handled.
The Basics of Exception Handling in Python
Python uses a set of keywords to manage exceptions: try, except, else, and finally. Let’s break down how each of these works.
1. The try Block
The try block contains code that may raise an exception. If no exceptions occur, the program continues executing normally.
2. The except Block
The except block allows you to handle specific exceptions that may arise in the try block. You can catch multiple exceptions or handle them individually.
3. The else Block
The else block is executed if no exceptions are raised in the try block. This is useful for code that should only run when no errors occur.
4. The finally Block
The finally block is executed after the try and except blocks, regardless of whether an exception was raised. This is often used for cleanup actions, such as closing files or releasing resources.
Example of Exception Handling in Python
Here’s a practical example to demonstrate how exception handling works in Python:
def divide(a, b):
try:
result = a / b
except ZeroDivisionError:
return "Error: Cannot divide by zero."
except TypeError:
return "Error: Both inputs must be numbers."
else:
return result
finally:
print("Execution of divide function complete.")
# Usage
print(divide(10, 2)) # Outputs: 5.0
print(divide(10, 0)) # Outputs: Error: Cannot divide by zero.
print(divide(10, "a")) # Outputs: Error: Both inputs must be numbers.
Output:
Execution of divide function complete.
5.0
Execution of divide function complete.
Error: Cannot divide by zero.
Execution of divide function complete.
Error: Both inputs must be numbers.
In this example:
The try block attempts to perform the division.
If a ZeroDivisionError is raised, it’s caught in the first except block.
If a TypeError occurs (due to incompatible types), it’s handled in the second except block.
If no exceptions occur, the result is returned in the else block.
The finally block runs regardless of the outcome, indicating the function's execution is complete.
Why Handle Exceptions?
Effective exception handling is crucial for several reasons:
Prevent Crashes: Proper handling allows your program to recover from errors without crashing.
User Experience: Providing meaningful error messages improves user experience by guiding users through issues instead of leaving them in the dark.
Debugging: Logging exceptions helps developers diagnose problems quickly and improve the code over time.
Best Practices for Exception Handling
Catch Specific Exceptions: Always aim to catch specific exceptions rather than using a general except: clause. This helps avoid masking unrelated issues.
try:
# Risky operation
except ValueError:
# Handle ValueError specifically
Avoid Silent Failures: Don’t ignore exceptions. Always handle them appropriately or log them for further analysis.
Use the finally Block for Cleanup: Utilize the finally block for any cleanup actions, ensuring that resources are released even when exceptions occur.
Document Exceptions: Clearly document any exceptions that your functions might raise. This helps other developers (and future you) understand potential issues.
Use Custom Exceptions: If your program has specific error conditions, consider creating custom exception classes. This can make your code more readable and maintainable.
class CustomError(Exception):
pass
Conclusion
In summary, exceptions in Python are a powerful mechanism for handling errors and managing program flow. They allow developers to anticipate and respond to potential issues in a controlled manner, enhancing the robustness of applications. By leveraging exception handling, you can avoid abrupt program crashes, providing users with a smoother experience and clearer feedback when something goes wrong.
Moreover, understanding how to use exceptions effectively not only improves error management but also enhances code readability and maintainability. When exceptions are documented and handled properly, other developers (and future you) can easily understand the potential pitfalls of your code.
Additionally, mastering exceptions opens the door to advanced programming techniques, such as creating custom exception classes tailored to your application's specific needs. This can lead to clearer error reporting and more meaningful responses to different failure scenarios.
Ultimately, exceptions are not just about catching errors; they are about improving the overall quality and reliability of your software. By incorporating proper exception handling practices into your coding habits, you can create applications that are not only functional but also resilient, paving the way for a better user experience and a more professional codebase.
Comentários