Natural Language Processing (NLP) is an evolving field that bridges the gap between human communication and machine understanding. As more applications require the ability to process and analyze large amounts of text, efficient NLP tools have become essential. One such tool is spaCy, a popular Python library known for its speed, efficiency, and ease of use in NLP tasks. In this blog, we’ll explore spaCy, its key features, and how it can be used to process and analyze text data.
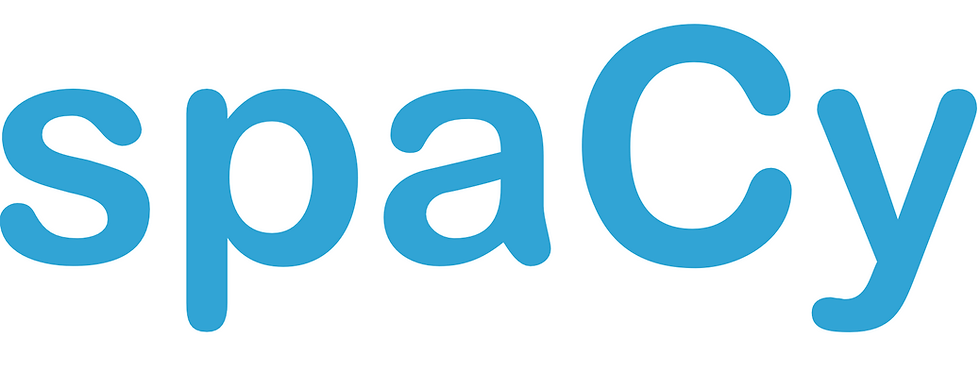
What is spaCy in Python?
spaCy is a powerful and fast open-source library in Python, specifically designed for advanced Natural Language Processing (NLP) tasks. Unlike traditional NLP libraries that focus on research and academic purposes, spaCy is built with a strong emphasis on real-world applications, making it a preferred choice for developers and data scientists who need to process large volumes of text efficiently. It provides a suite of tools and pre-trained models for tasks such as tokenization, part-of-speech (POS) tagging, named entity recognition (NER), dependency parsing, and lemmatization, all optimized for speed and accuracy. spaCy’s intuitive API allows users to easily integrate these capabilities into their applications, making it possible to build complex NLP pipelines and systems with minimal effort. Moreover, spaCy is designed to handle multilingual data, offering models for various languages, and can be extended with custom components to suit specific project requirements. This combination of speed, flexibility, and ease of use has made spaCy a go-to library for NLP projects in both academic research and industry applications.
Installing spaCy
To get started with spaCy, you first need to install the library. You can do this using pip:
pip install spacy
After installing spaCy, you’ll need to download a language model. spaCy offers several models for different languages, with varying sizes depending on the task:
python -m spacy download en_core_web_sm
This command downloads the small English model, which is suitable for many basic NLP tasks.
Key Features of spaCy
spaCy offers a rich set of key features that make it a standout tool for Natural Language Processing (NLP) in Python. One of its primary strengths is its highly efficient tokenization, which breaks down text into individual words and punctuation with speed and precision. Another essential feature is part-of-speech (POS) tagging, which identifies the grammatical role of each word in a sentence, helping to understand the structure of the text. spaCy also excels in named entity recognition (NER), automatically identifying and classifying entities like names, dates, and locations within the text. Its dependency parsing feature analyzes sentence structure, revealing relationships between words and helping to understand the syntax. Additionally, lemmatization reduces words to their base forms, ensuring consistency across different word forms. spaCy’s models are pre-trained and optimized for performance, allowing for real-time processing, and its extensible architecture supports custom pipelines and components, making it adaptable to a wide range of NLP tasks.
1. Text Tokenization
Tokenization is the process of splitting text into individual tokens (words, punctuation, etc.). spaCy’s tokenizer is highly efficient and handles a wide range of languages and special cases.
import spacy
# Load the English language model
nlp = spacy.load('en_core_web_sm')
# Sample text
text = "spaCy is an amazing NLP library in Python!"
# Tokenization
doc = nlp(text)
tokens = [token.text for token in doc]
print("Tokens:", tokens)
Output for the above code:
Tokens: ['spaCy', 'is', 'an', 'amazing', 'NLP', 'library', 'in', 'Python', '!']
2. Part-of-Speech (POS) Tagging
POS tagging assigns parts of speech (e.g., noun, verb, adjective) to each token in the text, which is crucial for understanding the grammatical structure of sentences.
for token in doc:
print(f"{token.text}: {token.pos_}")
Output for the above code:
spaCy: INTJ
is: AUX
an: DET
amazing: ADJ
NLP: PROPN
library: NOUN
in: ADP
Python: PROPN
!: PUNCT
3. Named Entity Recognition (NER)
NER identifies entities such as people, organizations, dates, and locations in the text. spaCy’s pre-trained models can recognize a variety of named entities out-of-the-box.
for ent in doc.ents:
print(f"{ent.text}: {ent.label_}")
Output for the above code:
NLP: ORG
Python: GPE
4. Dependency Parsing
Dependency parsing analyzes the grammatical structure of a sentence, showing relationships between "head" words and words that modify those heads.
for token in doc:
print(f"{token.text}: {token.dep_} (head: {token.head.text})")
Output for the above code:
spaCy: nsubj (head: is)
is: ROOT (head: is)
an: det (head: library)
amazing: amod (head: library)
NLP: compound (head: library)
library: attr (head: is)
in: prep (head: library)
Python: pobj (head: in)
!: punct (head: is)
5. Text Lemmatization
Lemmatization reduces words to their base or dictionary form. This is useful for normalizing text and reducing different forms of a word to a common base.
lemmas = [token.lemma_ for token in doc]
print("Lemmas:", lemmas)
Output for the above code:
Lemmas: ['spacy', 'be', 'an', 'amazing', 'NLP', 'library', 'in', 'Python', '!']
6. Sentence Boundary Detection
spaCy can automatically detect sentence boundaries, which is useful for tasks like summarization and text segmentation.
sentences = list(doc.sents)
print("Sentences:", sentences)
Output for the above code:
Sentences: [spaCy is an amazing NLP library in Python!]
Working with Custom Pipelines with spaCy
spaCy allows you to create custom NLP pipelines tailored to specific tasks. You can add or remove components like tokenization, lemmatization, and NER according to your needs.
from spacy.language import Language
# Create a custom pipeline
@Language.component("custom_component")
def custom_component(doc):
# Custom processing logic here
print("Custom component applied")
return doc
# Add the custom component to the pipeline
nlp.add_pipe("custom_component", last=True)
# Process text with the custom pipeline
doc = nlp("Custom pipelines in spaCy are flexible and powerful!")
Output for the above code:
Custom component applied
Use Cases of spaCy
spaCy is versatile and can be applied to various NLP tasks:
Information Extraction: Extracting key pieces of information from large volumes of text, such as extracting names, dates, and locations from documents.
Sentiment Analysis: Analyzing the sentiment of text data, such as determining whether customer reviews are positive or negative.
Chatbots: Building conversational agents that can understand and generate human language.
Text Summarization: Creating concise summaries of longer texts, useful for news aggregation and content curation.
Machine Translation: Translating text from one language to another using statistical or neural models.
Document Categorization: Automatically categorizing documents into predefined categories, such as spam detection or topic classification.
Conclusion
spaCy stands out as a powerful and efficient NLP library in Python, designed for real-world applications. Its ease of use, combined with advanced features like tokenization, POS tagging, NER, and dependency parsing, makes it an excellent choice for developers and data scientists alike. Whether you’re building a chatbot, analyzing social media sentiment, or extracting information from text, spaCy provides the tools you need to handle NLP tasks effectively. As you continue to explore and implement NLP solutions, spaCy’s speed and flexibility will undoubtedly enhance your projects and enable you to deliver high-quality results in production environments.
Start experimenting with spaCy today and discover the potential of advanced NLP in your Python applications!
Comments