Exploring the A* Search Algorithm with Python
- Samul Black
- Aug 19, 2024
- 4 min read
The A* search algorithm is one of the most widely used algorithms for pathfinding and graph traversal. It combines the strengths of Dijkstra’s algorithm and Greedy Best-First Search, making it highly effective for finding the shortest path from a start node to a goal node in a weighted graph. In this blog, we’ll dive into the details of the A* algorithm, understand its components, and walk through its implementation in Python.
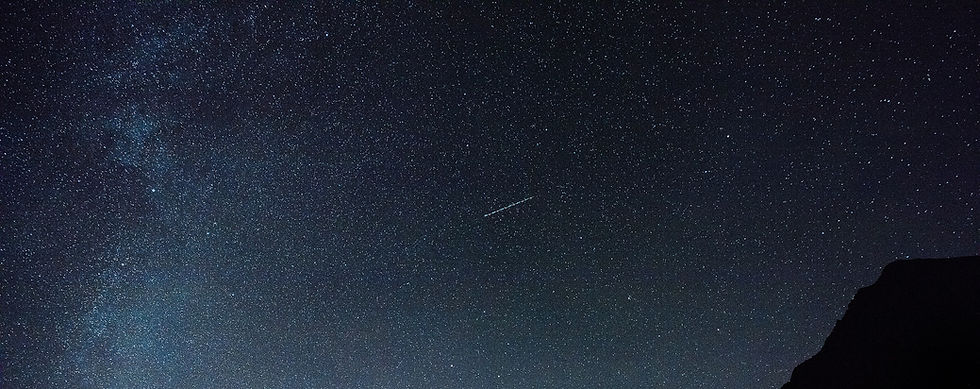
What is the A* Search Algorithm?
The A* search algorithm is an informed search algorithm that uses heuristics to guide the search process. It is widely used in various applications such as GPS navigation systems, game development, and robotics. The key idea behind A* is to evaluate nodes based on two functions:
g(n): The cost of the path from the start node to node n.
h(n): The estimated cost from node n to the goal node, provided by a heuristic function.
The algorithm uses these functions to compute a score, f(n), for each node:
f(n)=g(n)+h(n)
Where:
g(n) is the actual cost from the start node to node n.
h(n) is the estimated cost from node n to the goal.
The node with the lowest f(n) is expanded first, ensuring that the search is both optimal and efficient.
Key Concepts in A* Search Algorithm
Heuristic Function (h): A function that estimates the cost to reach the goal. It should be admissible (never overestimate) and ideally consistent (satisfies the triangle inequality) to ensure optimality.
Priority Queue: A data structure used to efficiently retrieve the node with the lowest f(n) score.
A* Search Algorithm - Algorithm Steps
Initialization: Start with the initial node and initialize the open list (nodes to be evaluated) and the closed list (nodes already evaluated).
Loop:
Remove the node with the lowest f(n) from the open list.
If the node is the goal, reconstruct the path and return it.
Generate the node's neighbors and calculate their g, h, and f values.
Update the open and closed lists accordingly.
Termination: The algorithm terminates when the goal node is found or the open list is empty (indicating no path exists).
Python Implementation for A* Search Algorithm
Let’s implement the A* search algorithm in Python. For simplicity, we’ll use a grid-based example where each cell represents a node and neighbors are adjacent cells.
import heapq
class Node:
def init(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 # Cost from start to this node
self.h = 0 # Heuristic cost from this node to goal
self.f = 0 # Total cost
def lt(self, other):
return self.f < other.f
def a_star_search(start, goal, grid):
open_list = []
closed_list = set()
start_node = Node(start)
goal_node = Node(goal)
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node.position)
if current_node.position == goal_node.position:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]: # Adjacent cells
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if (0 <= node_position[0] < len(grid)) and (0 <= node_position[1] < len(grid[0])) and grid[node_position[0]][node_position[1]] == 0:
if node_position in closed_list:
continue
neighbor = Node(node_position, current_node)
neighbor.g = current_node.g + 1
neighbor.h = ((goal_node.position[0] - neighbor.position[0]) 2) + ((goal_node.position[1] - neighbor.position[1]) 2)
neighbor.f = neighbor.g + neighbor.h
if any(open_node.position == neighbor.position and open_node.f <= neighbor.f for open_node in open_list):
continue
heapq.heappush(open_list, neighbor)
return None
# Example usage
grid = [[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 0, 0]]
start = (0, 0)
goal = (4, 4)
path = a_star_search(start, goal, grid)
print("Path:", path)
Output for the above code:
Path: [(0, 0), (0, 1), (0, 2), (0, 3), (0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
Explanation
Node Class: Represents each node in the search with attributes for position, parent, cost, and heuristic values.
Initialization: The start and goal nodes are initialized, and the open list (priority queue) is populated with the start node.
Search Loop: The main loop removes the node with the lowest f(n) from the open list, checks if it’s the goal, and processes its neighbors.
Path Reconstruction: If the goal node is reached, the path is reconstructed by tracing back from the goal node to the start node.
Applications of A* Search Algorithm
Pathfinding in Games: Used for character movement and navigation in grid-based games.
Navigation Systems: Helps in finding the shortest routes in GPS systems.
Robotics: Assists in planning paths for robots to navigate around obstacles.
Conclusion
The A* search algorithm is a powerful and versatile tool for finding optimal paths in weighted graphs. Its combination of Dijkstra’s algorithm’s completeness and Greedy Best-First Search’s efficiency makes it a go-to choice for many real-world applications. With the provided Python implementation, you can start using A* for your own pathfinding problems and explore its various applications.
Comentarios