When working with web applications, handling files like images, documents, or other media is a common requirement. JavaScript's FileReader API is a powerful tool that allows developers to read the contents of files stored on the user's computer. Whether you want to preview an image before uploading or process data from a text file, FileReader makes it seamless and efficient. In this blog, we'll explore what FileReader is, how it works, and how to use it effectively in real-world scenarios.
What is FileReader in javascript?
FileReader is a built-in JavaScript object that enables asynchronous reading of file contents. It allows you to read file data in various formats, such as text, data URLs (base64), and binary. The primary purpose of FileReader is to provide a way to read files selected by users through an <input type="file"> element or dragged and dropped into a web page.
Why Use FileReader?
Client-Side Processing: Read files without sending them to the server.
Asynchronous Operations: Non-blocking operations, allowing for better performance.
Multiple Formats: Read files as text, data URLs, or binary strings.
How Does FileReader Work?
The FileReader API works in conjunction with the File and Blob interfaces, which represent file-like objects. Here's how the process typically goes:
The user selects a file using an <input type="file"> element or drag-and-drop.
The selected file is represented as a File object.
FileReader reads the contents of the File object asynchronously.
The contents are returned in the chosen format (text, data URL, binary).
Basic Syntax
const reader = new FileReader();
reader.readAsText(file); // Reads the file as text
reader.readAsDataURL(file); // Reads the file as a base64-encoded data URL
reader.readAsArrayBuffer(file); // Reads the file as an ArrayBuffer
reader.readAsBinaryString(file); // Reads the file as a binary string (Deprecated)
Event Handlers
FileReader uses event-driven methods to handle file loading. The most commonly used events are:
onload: Triggered when the file is read successfully.
onerror: Triggered if an error occurs during reading.
onprogress: Triggered periodically during the reading process.
onloadstart: Triggered when the reading starts.
onloadend: Triggered when the reading is completed, regardless of success or failure.
Example 1: Reading Text Files
Let's start with a simple example of reading a text file and displaying its contents on a webpage.
<!DOCTYPE html>
<html>
<head>
<title>Read Text File</title>
</head>
<body>
<input type="file" id="fileInput">
<pre id="fileContent"></pre>
<script>
document.getElementById('fileInput').addEventListener('change',
function(event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = function(e) {
document.getElementById('fileContent').textContent = e.target.result;
};
reader.onerror = function(e) {
console.error('Error reading file:', e);
};
reader.readAsText(file);
}
});
</script>
</body>
</html>
Output:
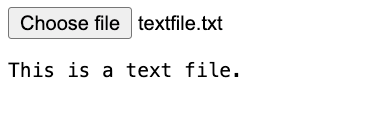
Explanation:
We use an <input> element to allow the user to select a file.
When a file is selected, an event listener triggers the FileReader.
The file is read as text using readAsText().
The contents are displayed within a <pre> element to preserve formatting.
Example 2: Previewing an Image
One of the most common uses of FileReader is to preview images before uploading them. Here's how to do it:
<!DOCTYPE html>
<html>
<head>
<title>Image Preview</title>
</head>
<body>
<input type="file" id="imageInput" accept="image/*">
<img id="imagePreview" style="max-width: 300px; display: none;">
<script>
document.getElementById('imageInput').addEventListener('change', function(event) {
const file = event.target.files[0];
if (file && file.type.startsWith('image/')) {
const reader = new FileReader();
reader.onload = function(e) {
const img = document.getElementById('imagePreview');
img.src = e.target.result;
img.style.display = 'block';
};
reader.readAsDataURL(file);
}
});
</script>
</body>
</html>
Output:
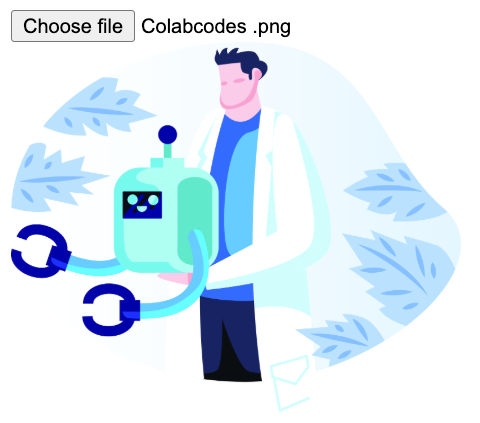
Error Handling
It's essential to handle errors when working with file operations. Here's how to do it:
reader.onerror = function(e) {
alert('Error reading file: ' + e.target.error.message);
};
Common errors include:
NotFoundError: The file could not be found.
SecurityError: A security issue prevented reading the file.
NotReadableError: The file could not be read due to a permission issue.
EncodingError: The file’s encoding is not supported.
Best Practices
File Type Validation: Always check the file type before reading it.
Error Handling: Implement comprehensive error handling.
Performance Optimization: For large files, consider reading in chunks.
Security Concerns: Avoid processing sensitive files on the client side.
Browser Compatibility
The FileReader API is well-supported across modern browsers, including Chrome, Firefox, Safari, and Edge. However, older versions of Internet Explorer (IE 9 and below) do not support it.
Conclusion
The FileReader API is a versatile and powerful tool for client-side file handling in JavaScript. It allows developers to create dynamic and interactive web applications, such as image previewers, text file readers, and more. By understanding its methods and events, you can leverage its full potential while ensuring robust error handling and optimal performance.
With the growing need for client-side processing, mastering FileReader is a valuable skill for modern web developers.
コメント