Functions in JavaScript
- Samul Black
- Dec 21, 2024
- 4 min read
Functions are one of the fundamental building blocks in JavaScript. They allow you to encapsulate blocks of code that perform specific tasks, making your programs more modular, reusable, and easier to read. In this tutorial, we will explore the concept of functions, their syntax, different types, and practical examples.
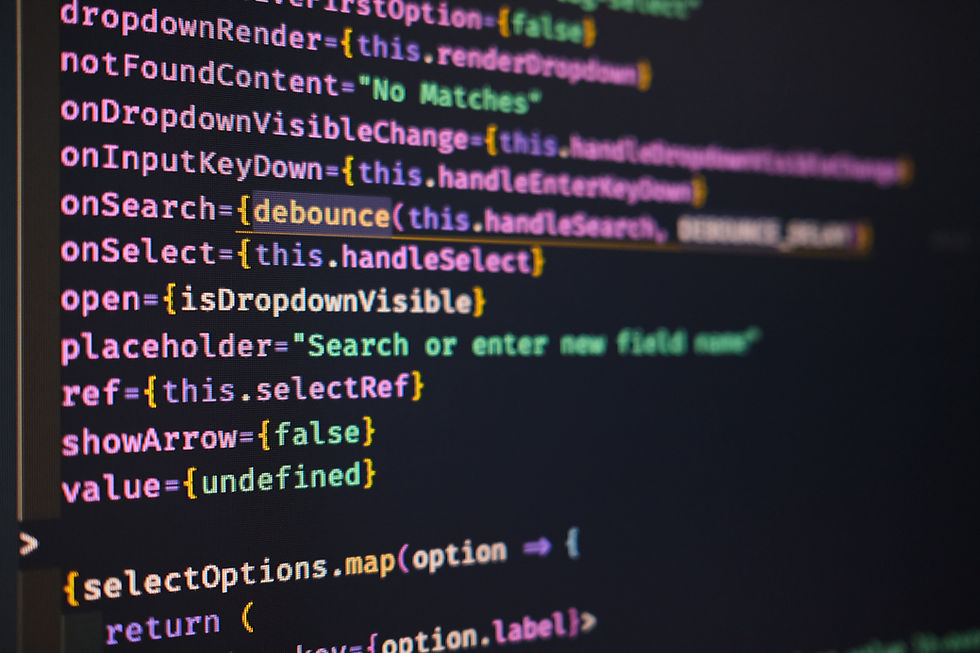
What is a Function in JavaScript?
A function in JavaScript is a reusable block of code designed to perform a particular task. Functions are executed when they are invoked (called). They are essential for reducing code redundancy and improving maintainability. By using functions, you can organize your code into logical units, making it easier to debug and update. Additionally, functions enhance the readability of your code by abstracting complex logic into simpler components.
Basic Syntax
Here is the basic syntax of a function in JavaScript:
function functionName(parameters) {
// Function body
// Code to be executed
return value; // Optional
}
function: The keyword to define a function.
functionName: The name of the function (used when calling it).
parameters: Optional input values passed to the function.
return: Specifies the output of the function (optional).
Example
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice")); // Output: Hello, Alice!
Types of Functions
1. Function Declarations
A function declaration defines a named function. These functions are hoisted, meaning they can be invoked before their definition in the code.
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // Output: 8
2. Function Expressions
A function expression defines a function as part of an expression. Unlike declarations, these are not hoisted.
const multiply = function (a, b) {
return a * b;
};
console.log(multiply(4, 3)); // Output: 12
3. Arrow Functions
Arrow functions provide a more concise syntax and do not have their own this binding.
const divide = (a, b) => a / b;
console.log(divide(10, 2)); // Output: 5
Multi-line Arrow Functions
const area = (length, width) => {
const result = length * width;
return result;
};
console.log(area(5, 4)); // Output: 20
4. Anonymous Functions
Anonymous functions are unnamed and are often used as arguments to other functions.
setTimeout(function () {
console.log("This message appears after 2 seconds");
}, 2000);
5. Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that is executed immediately after its definition.
(function () {
console.log("This is an IIFE");
})();
6. Higher-Order Functions
Functions that accept other functions as arguments or return functions are called higher-order functions.
Example: Using map
const numbers = [1, 2, 3, 4];
const squares = numbers.map(function (num) {
return num * num;
});
console.log(squares); // Output: [1, 4, 9, 16]
Parameters and Arguments
Default Parameters
You can provide default values for parameters.
function greet(name = "Guest") {
return `Hello, ${name}!`;
}
console.log(greet()); // Output: Hello, Guest!
console.log(greet("Alice")); // Output: Hello, Alice!
Rest Parameters
Rest parameters allow functions to accept an indefinite number of arguments as an array.
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4)); // Output: 10
Returning Values
Functions can return values using the return statement. If a function does not have a return, it returns undefined by default.
function double(num) {
return num * 2;
}
console.log(double(4)); // Output: 8
Scope and Closures
Scope
Scope determines the visibility of variables. JavaScript has two main types of scope:
Global Scope: Variables declared outside any function.
Local Scope: Variables declared inside a function.
let globalVar = "I am global";
function demoScope() {
let localVar = "I am local";
console.log(globalVar); // Accessible
console.log(localVar); // Accessible
}
console.log(globalVar); // Accessible
Closures
A closure is a function that retains access to its parent scope, even after the parent function has executed.
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log(`Outer: ${outerVariable}, Inner: ${innerVariable}`);
};
}
const newFunction = outerFunction("outside");
newFunction("inside"); // Output: Outer: outside, Inner: inside
Function Examples
Example 1: Calculator
This example implements a simple calculator that supports basic arithmetic operations. The function takes two numbers and an operation type (add, subtract, multiply, or divide) and performs the specified operation.
function calculator(a, b, operation) {
switch (operation) {
case "add":
return a + b;
case "subtract":
return a - b;
case "multiply":
return a * b;
case "divide":
return a / b;
default:
return "Invalid operation";
}
}
console.log(calculator(10, 5, "add")); // Output: 15
console.log(calculator(10, 5, "divide")); // Output: 2
Example 2: Palindrome Checker
This function checks if a given string is a palindrome. It reverses the input string and compares it to the original to determine if they are identical.
function isPalindrome(str) {
const reversed = str.split("").reverse().join("");
return str === reversed;
}
console.log(isPalindrome("racecar")); // Output: true
console.log(isPalindrome("hello")); // Output: false
Example 3: Factorial
This recursive function calculates the factorial of a given number. If the input number is 0, it returns 1; otherwise, it multiplies the number by the factorial of (number - 1).
function factorial(num) {
if (num === 0) return 1;
return num * factorial(num - 1);
}
console.log(factorial(5)); // Output: 120
Example 4: Sorting an Array
This example demonstrates sorting an array of numbers in ascending order. The sort method uses a comparison function to arrange the elements.
const numbers = [4, 2, 9, 1, 5];
numbers.sort((a, b) => a - b);
console.log(numbers); // Output: [1, 2, 4, 5, 9]
Example 5: Fetching Data (Async Function)
This asynchronous function fetches data from a given API and logs the result. It uses fetch to send a request and handles errors with a try-catch block.
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchData("https://jsonplaceholder.typicode.com/posts/1");
Conclusion
Functions in JavaScript are powerful tools that help you write clean, efficient, and reusable code. From simple arithmetic to complex asynchronous operations, functions form the backbone of any JavaScript application. By mastering functions, you can significantly enhance your ability to write robust and maintainable programs. Continue practicing by experimenting with different types of functions and exploring real-world scenarios to solidify your understanding!
Comments