Python’s generator functions provide an efficient and powerful way to iterate over data without storing it in memory all at once. When working with large datasets or streams of data, generators offer an elegant solution by producing values on demand, saving memory and enhancing performance. In this blog, we'll dive into the fundamentals of generators, how they differ from regular functions and iterators, and their practical applications.
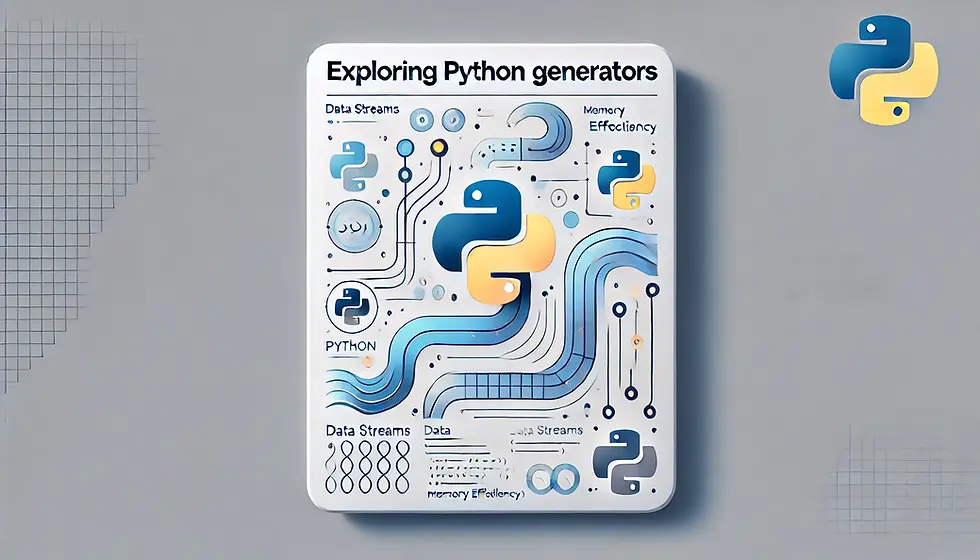
What Are Generators in Python?
Generators are a type of iterable, like lists or tuples, but they allow you to iterate over data without storing everything in memory. They generate values on the fly using the yield keyword, making them suitable for operations on large datasets where memory efficiency is crucial.
A generator is defined using a function, but instead of returning a value and ending the function, it uses yield to return a value and pause the function, allowing it to resume where it left off. Generators work by maintaining the state of the function between successive calls. When a generator is called, it returns an iterator object, but unlike a regular function, it does not execute the function immediately. Instead, it returns an object that controls the execution.
Here’s a simple example:
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
for value in gen:
print(value)
Output:
1
2
3
In this example, yield pauses the function after each return. When the function is resumed (by iterating over it), it continues from where it was last paused. Difference Between yield and return:
return: Terminates the function and returns a value.
yield: Pauses the function, saving the state of the local variables, and can be resumed later.
The key difference is that yield allows the function to be resumed, whereas return finalizes the function and exits.
Practical Use Cases of Generators in Python
Generators shine when dealing with large datasets or when working in resource-constrained environments. Here are some common use cases:
1. Reading Large Files Line by Line
Instead of loading an entire file into memory, which might be impractical for large files, you can use a generator to read it one line at a time.
def read_large_file(file_name):
with open(file_name) as file:
for line in file:
yield line.strip()
for line in read_large_file('hello.txt'):
print(line)
Output:
This is a new line. The echo command outputs the string Hello, World!, ...
This approach ensures that only one line is loaded into memory at any given time, making it highly efficient for large files.
2. Generating Infinite Sequences
Generators can produce values on the fly, which makes them ideal for creating infinite sequences like Fibonacci numbers or other mathematical series.
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib_gen = fibonacci()
for _ in range(10):
print(next(fib_gen))
Output:
0
1
1
2
3
5
8
13
21
34
3. Streaming Data Processing
In applications that need to process data streams (like real-time log analysis or sensor data collection), generators can provide an efficient way to handle continuous data streams without overwhelming memory.
import random
def sensor_data():
while True:
yield random.randint(1, 100)
data_stream = sensor_data()
for _ in range(5):
print(next(data_stream))
Output(random values):
45
56
65
52
56
Combining Generators for Pipelines
You can chain multiple generators to create data processing pipelines. This is a great way to modularize data processing and apply transformations in a memory-efficient manner.
For example, let's create two generators, one to generate squares and another to filter even numbers:
def generate_numbers(limit):
for i in range(limit):
yield i
def square_numbers(numbers):
for num in numbers:
yield num * num
def filter_even_numbers(numbers):
for num in numbers:
if num % 2 == 0:
yield num
# Chaining generators
numbers = generate_numbers(10)
squares = square_numbers(numbers)
even_squares = filter_even_numbers(squares)
for square in even_squares:
print(square)
Output:
0
4
16
36
64
In this example, each generator passes its output to the next, creating an efficient and memory-friendly pipeline that processes the data step by step.
Generator Expressions
Generator expressions offer a concise way to create generators, similar to list comprehensions but with the memory efficiency of a generator. You use parentheses () instead of square brackets [].
Example of a generator expression:
gen_exp = (x * x for x in range(10))
print(list(gen_exp)) # Outputs: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
This is similar to:
def square_gen():
for x in range(10):
yield x * x
print(list(square_gen())) # Outputs: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Generator expressions are useful when you want to create simple generators in a single line of code.
send() and close() Methods for Generators in Python
In addition to yield, Python generators also support two important methods: send() and close().
1. send() Method:
This method allows the calling code to send a value back into the generator, resuming execution at the yield expression and passing the value sent.
def echo():
while True:
value = yield # Wait for a value to be sent
print(f"Received: {value}")
# Create a generator
generator = echo()
# Start the generator
next(generator)
# Send values to the generator
generator.send("Hello")
generator.send("World")
Output:
Received: Hello
Received: World
2. close() Method:
This method is used to stop the generator, raising a GeneratorExit exception inside the generator function.
def my_generator():
while True:
yield 1
gen = my_generator()
print(next(gen)) # output1
gen.close()
Advantages of Generators
Generators in Python offer significant advantages, particularly in terms of memory efficiency and performance. By yielding values one at a time, they avoid storing entire data sets in memory, making them ideal for large data processing. Generators support lazy evaluation, producing values only when needed, which improves efficiency. Additionally, they simplify the creation of data processing pipelines, allowing you to chain operations without loading everything at once, leading to faster, more scalable code.
Memory Efficiency: Since generators don’t hold the entire data in memory, they are perfect for processing large datasets or continuous data streams.
Lazy Evaluation: Generators generate values on-the-fly, which means that they evaluate data only when needed.
Chaining and Pipelining: Generators can be chained together, creating a memory-efficient data processing pipeline.
Improved Performance: With generators, there's no need to wait for an entire dataset to be loaded before processing begins. You can start consuming data immediately.
Conclusion
Generators are a powerful feature in Python that allows you to handle large data streams and complex processing tasks with ease. Their memory efficiency and ability to work in pipelines make them indispensable in scenarios where performance and resource management are critical. By mastering generators, you can write Python code that is both more elegant and highly efficient, especially when dealing with large datasets or continuous streams of data.
Comments