The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of an HTML or XML document as a tree of objects, allowing developers to access, manipulate, and update the content and structure of a webpage dynamically. This tutorial will help you get started with DOM manipulation in JavaScript, including practical examples.
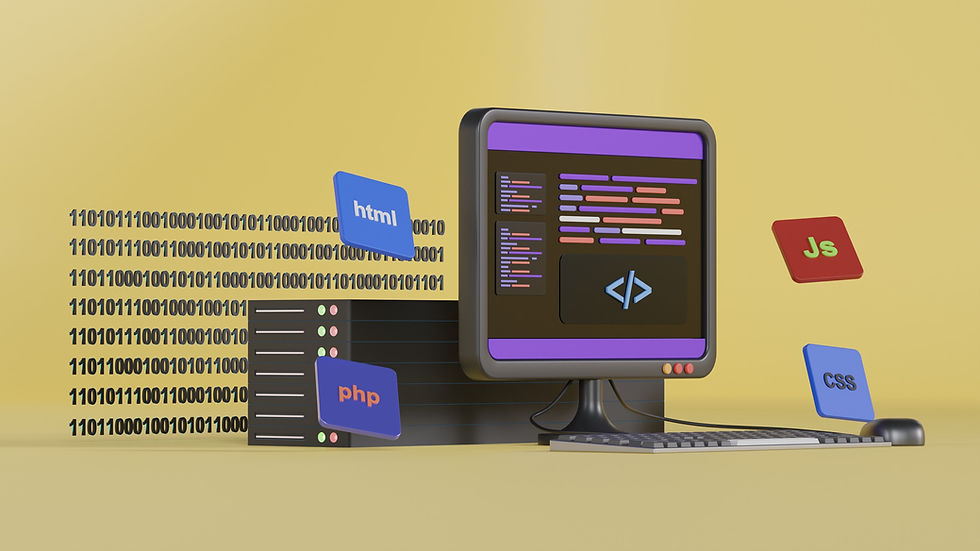
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM is a hierarchical structure representing the content of a webpage. It provides a way to:
Access HTML elements and attributes.
Modify content dynamically.
Respond to user interactions.
In the DOM (Document Object Model), the terms node and element are fundamental concepts that represent the building blocks of a document.
Node
A node is the most basic unit in the DOM tree. It represents everything in the document, including elements, text, comments, and even the document itself. Nodes can be of various types, defined by the Node interface.
Common Node Types:
Element Node (Node.ELEMENT_NODE): Represents an HTML or XML element (e.g., <div>, <p>).
Text Node (Node.TEXT_NODE): Represents the text content inside an element.
Comment Node (Node.COMMENT_NODE): Represents comments in the document (e.g., <!-- Comment -->).
Document Node (Node.DOCUMENT_NODE): Represents the entire document itself (e.g., document object).
Element
An element is a specific type of node that represents HTML or XML tags. It is a subset of nodes and corresponds to the tags in your document (e.g., <body>, <h1>, <p>).
Characteristics of Elements:
Attributes: Elements can have attributes (e.g., class, id, src).
Children: Elements can contain other nodes as children (e.g., other elements or text nodes).
Methods: Elements provide specific methods like getAttribute, setAttribute, appendChild, and removeChild.
Accessing Document Object Model (DOM) Elements
Accessing the Document Object Model (DOM) allows developers to dynamically interact with web pages by selecting elements to read or modify their content, attributes, and styles. JavaScript provides several methods for accessing DOM elements, such as getElementById for selecting elements by their ID, getElementsByClassName for selecting by class, and querySelector for versatile CSS-style selectors. Once accessed, these elements can be manipulated to create dynamic, interactive web experiences. JavaScript provides several methods to select and access elements in the DOM.
a. By ID
Use getElementById to select an element by its ID.
<!DOCTYPE html><html><body>
<h1 id="title">Hello, DOM!</h1>
<script>
const title = document.getElementById("title");
console.log(title.textContent); // Output: Hello, DOM!
</script></body>
</html>
b. By Class
Use getElementsByClassName to select elements by their class name.
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<script>
const items = document.getElementsByClassName("item");
console.log(items[0].textContent); // Output: Item 1
</script>
c. By Tag
Use getElementsByTagName to select elements by their tag name.
<p>Paragraph 1</p>
<p>Paragraph 2</p>
<script>
const paragraphs = document.getElementsByTagName("p");
console.log(paragraphs.length); // Output: 2
</script>
d. Query Selectors
Use querySelector or querySelectorAll for more flexible selection.
<div class="container">
<p class="text">Hello, World!</p></div>
<script>
const text = document.querySelector(".container .text");
console.log(text.textContent); // Output: Hello, World!</script>
Manipulating Document Object Model (DOM) Elements
Manipulating Document Object Model (DOM) elements allows developers to dynamically update the content, structure, and style of web pages. Using JavaScript, you can access elements through methods like getElementById or querySelector, modify their content with properties like textContent or innerHTML, update attributes with setAttribute, and style them using the style property. DOM manipulation is essential for creating interactive and responsive web experiences, enabling real-time updates based on user interactions or external data.Once you access an element, you can modify its content, attributes, or style.
a. Modify Content
Use textContent or innerHTML to change an element's content.
<p id="message">Original Message</p><script>
const message = document.getElementById("message");
message.textContent = "Updated Message";
</script>
b. Modify Attributes
Use setAttribute or direct property access to update attributes.
<img id="image" src="old.jpg" alt="Old Image"><script>
const image = document.getElementById("image");
image.setAttribute("src", "new.jpg");
</script>
c. Modify Styles
Use the style property to change an element's CSS.
<div id="box">Box</div><script>
const box = document.getElementById("box");
box.style.backgroundColor = "blue";
box.style.color = "white";
</script>
Adding and Removing Elements
Adding and removing elements in the DOM allows developers to dynamically modify the structure of a webpage. New elements can be created using the createElement method and added to the document with appendChild or insertBefore. Conversely, elements can be removed using the removeChild method or directly with remove. These operations are essential for building dynamic content, such as adding tasks in a to-do list or removing items from a shopping cart, enabling a more interactive and user-friendly web experience.
a. Create Elements
Use createElement to create new DOM elements.
<div id="container"></div><script>
const container = document.getElementById("container");
const newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph.";
container.appendChild(newElement);
</script>
b. Remove Elements
Use removeChild or remove to delete elements.
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
</ul>
<script>
const list = document.getElementById("list");
const firstItem = list.children[0];
list.removeChild(firstItem);
</script>
Event Handling
Events allow you to add interactivity to your web pages.
a. Add Event Listeners
Use addEventListener to attach event handlers.
<button id="btn">Click Me</button>
<script>
const button = document.getElementById("btn");
button.addEventListener("click", () => {
alert("Button clicked!");
});
</script>
b. Respond to Input Events
<input id="input" type="text" placeholder="Type something">
<script>
const input = document.getElementById("input");
input.addEventListener("input", (event) => {
console.log("You typed:", event.target.value);
});
</script>
Traversing the Document Object Model (DOM)
Navigate through elements using properties like parentNode, childNodes, nextSibling, and previousSibling.
Example:
<ul id="list">
<li>First</li>
<li>Second</li>
</ul>
<script>
const list = document.getElementById("list");
console.log(list.firstElementChild.textContent); // Output: First
console.log(list.lastElementChild.textContent); // Output: Second
</script>
Conclusion
The DOM is an essential part of web development, providing the foundation for creating interactive and dynamic web pages. By mastering DOM manipulation, you can build engaging user experiences. Practice with the examples above and explore more advanced techniques as you grow your skills.
Comments