Graph Neural Networks (GNNs) have emerged as powerful tools for learning on graph-structured data. Unlike traditional neural networks, which operate on Euclidean data like images and text, GNNs excel at processing data that can be represented as graphs, such as social networks, molecular structures, and knowledge graphs. In this blog, we'll explore the basics of GNNs, their applications, and how to implement them using Python libraries like PyTorch Geometric.
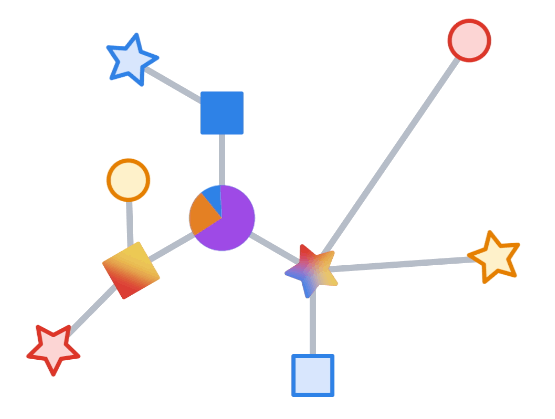
What are Graph Neural Networks?
Graph Neural Networks (GNNs) are a class of neural networks specifically designed to process and analyze data structured as graphs. Unlike traditional neural networks, which work well with grid-like data such as images or text, GNNs excel in capturing the complex relationships and interdependencies between entities represented as nodes in a graph. Each node in a graph can represent an object, while the edges between nodes capture the relationships or interactions between these objects. By iteratively aggregating and updating node features based on their neighbors' information, GNNs can learn rich representations that are highly effective for tasks like node classification, link prediction, and graph classification. This makes them particularly valuable in domains such as social network analysis, molecular chemistry, recommendation systems, and more, where the data naturally forms a graph structure.
What is a Graph?
Before diving into GNNs, let's understand what a graph is. A graph is a collection of nodes (or vertices) connected by edges. Each node can represent an entity, and the edges represent relationships between these entities. Graphs can be directed or undirected, weighted or unweighted.
Why Use Graph Neural Networks?
Graphs are ubiquitous in various domains:
Social Networks: Users (nodes) connected by friendships (edges).
Molecular Structures: Atoms (nodes) connected by chemical bonds (edges).
Knowledge Graphs: Entities (nodes) connected by relationships (edges).
GNNs are designed to leverage the structural information inherent in these graphs, making them suitable for tasks like node classification, link prediction, and graph classification.
How Do Graph Neural Networks Work?
GNNs operate by iteratively updating the representation of each node based on its neighbors' features. The basic idea is to aggregate information from the local neighborhood of each node and then use this aggregated information to update the node's representation. This process is repeated for several layers, allowing the network to capture higher-order dependencies in the graph. The general framework for a GNN can be summarized as follows:
Message Passing: Nodes send messages to their neighbors.
Aggregation: Each node aggregates the received messages.
Update: The node's representation is updated based on the aggregated messages.
Prediction: The final node or graph representation is used for downstream tasks like classification or regression.
Implementing Graph Neural Networks (GNNs) in Python with PyTorch Geometric
PyTorch Geometric is a popular library for building GNNs in Python. It provides easy-to-use tools for creating and working with graph data structures.
Let's walk through a simple example of implementing a GNN for node classification using PyTorch Geometric.
Graph Neural Networks (GNNs)
First, you need to install PyTorch and PyTorch Geometric. You can do this using pip:
pip install torch
pip install torch-geometric
Step 2: Load the Data
We'll use the Cora dataset, a common benchmark for node classification. The dataset is available in PyTorch Geometric.
import torch
from torch_geometric.datasets import Planetoid
# Load the Cora dataset
dataset = Planetoid(root='/tmp/Cora', name='Cora')
# Extract the graph data
data = dataset[0]
print(data)
Output for the above code:
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.x
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.tx
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.allx
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.y
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.ty
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.ally
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.graph
Downloading https://github.com/kimiyoung/planetoid/raw/master/data/ind.cora.test.index
Data(x=[2708, 1433], edge_index=[2, 10556], y=[2708], train_mask=[2708], val_mask=[2708], test_mask=[2708])
Processing...
Done!
Step 3: Define the Graph Neural Networks (GNNs) Model
We'll define a simple Graph Convolutional Network (GCN), a popular type of GNN.
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
class GCN(nn.Module):
def init(self):
super(GCN, self).__init__()
self.conv1 = GCNConv(dataset.num_node_features, 16)
self.conv2 = GCNConv(16, dataset.num_classes)
def forward(self, data):
x, edge_index = data.x, data.edge_index
x = self.conv1(x, edge_index)
x = F.relu(x)
x = self.conv2(x, edge_index)
return F.log_softmax(x, dim=1)
model = GCN()
Step 4: Train the Model
Next, we'll train the model using the Adam optimizer.
optimizer = torch.optim.Adam(model.parameters(), lr=0.01, weight_decay=5e-4)
def train():
model.train()
optimizer.zero_grad()
out = model(data)
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
return loss.item()
for epoch in range(200):
loss = train()
print(f'Epoch {epoch+1}, Loss: {loss:.4f}')
Output for the above code:
Epoch 184, Loss: 0.0109
Epoch 185, Loss: 0.0109
Epoch 186, Loss: 0.0109
Epoch 187, Loss: 0.0108
Epoch 188, Loss: 0.0108
Epoch 189, Loss: 0.0108
Epoch 190, Loss: 0.0107
Epoch 191, Loss: 0.0107
Epoch 192, Loss: 0.0107
Epoch 193, Loss: 0.0106
Epoch 194, Loss: 0.0106
Epoch 195, Loss: 0.0106
Epoch 196, Loss: 0.0106
Epoch 197, Loss: 0.0105
Epoch 198, Loss: 0.0105
Epoch 199, Loss: 0.0105
Epoch 200, Loss: 0.0104
Step 5: Evaluate the Model
Finally, we'll evaluate the model's performance on the test set.
def test():
model.eval()
out = model(data)
pred = out.argmax(dim=1)
correct = pred[data.test_mask] == data.y[data.test_mask]
accuracy = int(correct.sum()) / int(data.test_mask.sum())
return accuracy
accuracy = test()
print(f'Test Accuracy: {accuracy:.4f}')
Output for the above code:
Test Accuracy: 0.8060
Applications of Graph Neural Networks
Graph Neural Networks (GNNs) have a wide range of applications across various domains due to their ability to effectively model and analyze graph-structured data. Some key applications include:
1. Social Network Analysis
Graph Neural Networks (GNNs) are highly effective in social network analysis, where users are represented as nodes, and relationships such as friendships or interactions are represented as edges. GNNs can model the complex, dynamic connections within social networks, enabling tasks like user classification, community detection, and link prediction. For example, GNNs can identify influential users, predict the likelihood of forming new connections, or detect communities of closely connected users. This capability is crucial for applications such as targeted marketing, recommendation systems, and detecting fake accounts or spreading misinformation.
2. Molecular Chemistry
In molecular chemistry, GNNs are used to predict the properties and behaviors of molecules by modeling their atomic structures as graphs. Atoms serve as nodes, while chemical bonds between them are edges. GNNs can learn how the molecular structure influences properties like toxicity, solubility, or reactivity, making them invaluable for tasks like drug discovery and material science. By accurately predicting molecular properties, GNNs help in identifying promising drug candidates and designing new materials with desired characteristics, significantly speeding up the research and development process.
3. Recommender Systems
Recommender systems benefit from GNNs by modeling users and items as nodes in a bipartite graph, where edges represent interactions such as purchases, likes, or ratings. GNNs can capture the complex relationships between users and items, allowing for more accurate predictions of user preferences. By analyzing the structure of the interaction graph, GNNs can recommend items that a user is likely to be interested in, even if they haven't interacted with those items directly. This approach enhances personalization and improves user experience in platforms like e-commerce, streaming services, and social media.
4. Knowledge Graphs
Knowledge graphs, which represent entities and their relationships in a structured form, are another area where GNNs shine. In these graphs, entities are nodes, and the relationships between them are edges. GNNs can be used for tasks such as entity classification, relation prediction, and knowledge graph completion. By leveraging the rich relational structure of knowledge graphs, GNNs can infer missing information, improve the accuracy of search results, and enhance the performance of natural language processing tasks. This makes them essential for applications like semantic search, question answering, and information retrieval.
5. Traffic and Transportation Networks
GNNs are increasingly applied in traffic and transportation networks, where roads, intersections, and vehicles can be modeled as nodes and edges in a graph. By analyzing these networks, GNNs can help in optimizing traffic flow, predicting traffic congestion, and planning efficient routes. For instance, GNNs can model the impact of road closures or accidents on traffic patterns, enabling real-time adjustments to traffic signals or route suggestions. In public transportation, GNNs can optimize schedules and predict passenger demand, improving the efficiency and reliability of transportation systems.
Conclusion
Graph Neural Networks offer a powerful approach to learning from graph-structured data. With libraries like PyTorch Geometric, implementing GNNs has become more accessible. In this blog, we walked through a simple implementation of a GCN for node classification. The flexibility and effectiveness of GNNs make them a valuable tool in many domains, and their potential is only beginning to be tapped. Graph Neural Networks (GNNs) are revolutionizing the way we approach data that naturally forms a graph structure. Their ability to capture and model the intricate relationships between entities in various domains—ranging from social networks to molecular chemistry, recommender systems, knowledge graphs, and traffic networks—makes them an invaluable tool in modern data science and machine learning. As we've seen, GNNs are versatile and powerful, enabling more accurate predictions, better understanding of complex systems, and improved decision-making processes. With ongoing advancements in GNN methodologies and the increasing availability of graph-structured data, the applications of GNNs will continue to expand, unlocking new possibilities across industries. Whether you are looking to enhance recommendations, predict molecular properties, or optimize transportation networks, GNNs offer a robust framework for solving a wide array of real-world challenges
Comentários