JavaScript is one of the most popular programming languages in the world, widely used for web development to make web pages interactive, build complex applications, and manage dynamic content. This tutorial provides a beginner-friendly introduction to JavaScript, its key features, and basic syntax to get you started.
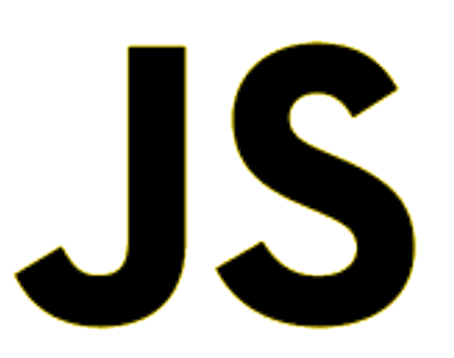
Why Learn JavaScript?
JavaScript is essential for web development and has several advantages:
Client-Side Scripting: Runs directly in the browser, providing fast and interactive user experiences.
Versatile: Can be used for frontend (via frameworks like React) and backend (using Node.js).
Rich Ecosystem: Offers a vast library of tools and frameworks to streamline development.
Cross-Platform: Works across different browsers and devices without modifications.
Learning JavaScript also opens doors to mastering frameworks and libraries such as React, Angular, and Vue, which are widely used in modern web development. Additionally, its use in backend development with Node.js ensures you can create full-stack applications using just one language.
JavaScript and Web Development
JavaScript is at the heart of web development. It enables developers to create dynamic and interactive user interfaces that respond to user input without requiring page reloads. Alongside HTML and CSS, JavaScript forms the foundation of modern web technologies. Frameworks like React, Angular, and Vue leverage JavaScript to simplify the development of complex applications, while libraries like jQuery offer utilities for handling common tasks with ease. Moreover, JavaScript is crucial for developing Single Page Applications (SPAs) and integrating with APIs to fetch or send data, making it a key player in both frontend and backend ecosystems.
Getting Started
To use JavaScript, all you need is a text editor (like VS Code) and a browser (like Chrome). JavaScript code can be embedded directly into HTML files or written in separate .js files.
Adding JavaScript to an HTML File
Here is an example of how to include JavaScript in an HTML file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Example</title>
</head>
<body>
<h1>Welcome to JavaScript</h1>
<p id="greeting"></p>
<script>
document.getElementById('greeting').textContent = 'Hello, JavaScript!';
</script>
</body>
</html>
When you open this file in a browser, the <p> tag will display "Hello, JavaScript!" because the script dynamically changes its content.
Basic Syntax
Let’s explore some fundamental concepts and syntax in JavaScript.
Variables
Variables are used to store data. You can declare variables using var, let, or const:
let name = "Alice"; // Can be reassigned
const age = 25; // Cannot be reassigned
var city = "New York"; // Older syntax
Data Types
JavaScript supports various data types:
String: Text data (e.g., "Hello")
Number: Numeric values (e.g., 42, 3.14)
Boolean: Logical values (true or false)
Array: Ordered collection of values (e.g., [1, 2, 3])
Object: Key-value pairs (e.g., { key: 'value' })
Functions
Functions allow you to encapsulate code and reuse it:
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice")); // Outputs: Hello, Alice!
Control Structures
Conditional Statements
Use if, else if, and else to control the flow of your program:
let time = 10;
if (time < 12) {
console.log("Good Morning");
} else if (time < 18) {
console.log("Good Afternoon");
} else {
console.log("Good Evening");
}
Loops
JavaScript provides several types of loops:
For Loop:
for (let i = 0; i < 5; i++) {
console.log(i);
}
While Loop:
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
DOM Manipulation
The Document Object Model (DOM) represents the structure of a web page, and JavaScript can be used to interact with it dynamically.
Selecting Elements
You can use methods like getElementById, querySelector, and getElementsByClassName to select elements:
let header = document.getElementById('greeting');
header.style.color = 'blue';
Adding Event Listeners
You can make web pages interactive by listening for user events:
document.querySelector('button').addEventListener('click', () => {
alert('Button Clicked!');
});
Conclusion
JavaScript is a powerful language for creating dynamic and interactive web pages. With the basics covered here, you can start building small applications and experimenting with your ideas. As you progress, explore advanced topics like:
JavaScript Frameworks (React, Angular, Vue)
Asynchronous Programming (Promises, Async/Await)
APIs and Fetch
Additionally, JavaScript's ecosystem continues to expand, offering new tools and libraries to tackle emerging challenges in web development. By mastering JavaScript, you open the door to opportunities in building cutting-edge applications, optimizing web performance, and contributing to open-source projects. Whether you aim to specialize in frontend, backend, or full-stack development, JavaScript remains an indispensable skill. Start your journey today and unlock the potential of the web!
Comments