PyTorch is one of the most popular and widely used libraries for deep learning in Python. Developed by Facebook's AI Research lab (FAIR), PyTorch has become the go-to tool for researchers and developers alike due to its flexibility, dynamic computation graph, and strong community support. In this blog, we'll explore what PyTorch is, why it's so popular, and how you can get started with it.
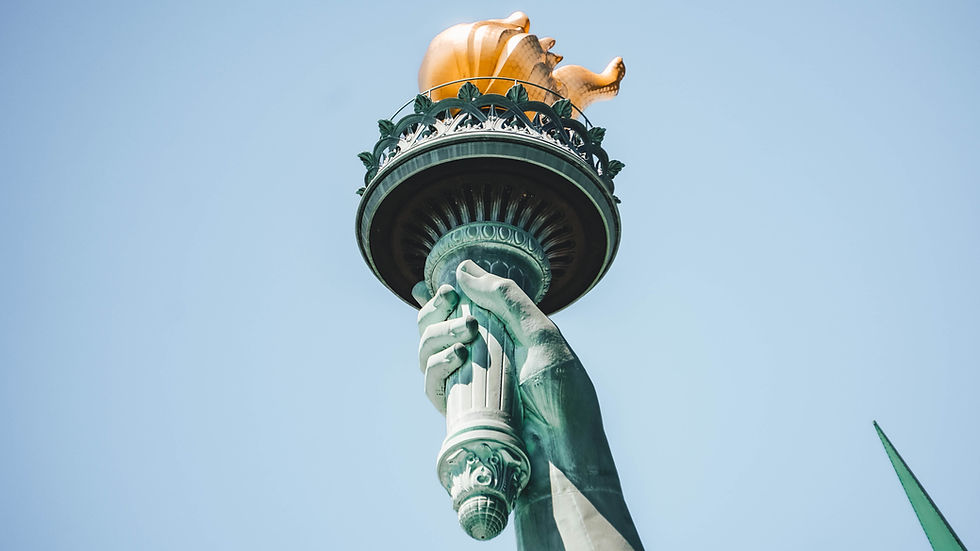
What is PyTorch in Python?
PyTorch is an open-source deep learning library developed by Facebook's AI Research lab (FAIR) that has become one of the most popular tools for machine learning and artificial intelligence applications in Python. It provides a flexible and dynamic platform for building, training, and deploying neural networks. Unlike other deep learning libraries that rely on static computation graphs, PyTorch uses dynamic computation graphs, which are constructed on-the-fly during runtime. This feature makes PyTorch particularly user-friendly and well-suited for research, as it allows for more intuitive model debugging and modification. PyTorch integrates seamlessly with Python, making it feel like a natural extension of the language, which reduces the learning curve for new users. It supports GPU acceleration, enabling faster computation, which is essential for training large-scale models. PyTorch also includes a rich ecosystem of libraries and tools, such as TorchVision for computer vision tasks and TorchText for natural language processing, further expanding its utility in various domains of deep learning. Overall, PyTorch's combination of flexibility, ease of use, and powerful capabilities has made it a preferred choice for researchers, developers, and data scientists working on cutting-edge AI projects. Before diving into the technical aspects, let's first understand why PyTorch has gained such immense popularity:
Dynamic Computation Graphs: PyTorch uses dynamic computation graphs, meaning the graph is generated on the fly as operations are performed. This makes debugging easier and allows for more flexibility in designing complex models.
Pythonic and Intuitive: PyTorch feels like a natural extension of Python, making it easy to learn and use. Its API is designed to be intuitive, enabling developers to focus more on the model's logic rather than on handling technical complexities.
Strong Community and Ecosystem: PyTorch has a strong community, with extensive documentation, tutorials, and third-party libraries that extend its functionality. This community support makes it easier to find solutions and get started quickly.
Integration with Python Libraries: PyTorch integrates seamlessly with popular Python libraries like NumPy, SciPy, and Matplotlib, allowing for easy data manipulation, analysis, and visualization.
Getting Started with PyTorch in Python
Getting started with PyTorch in Python is straightforward, making it an excellent choice for both beginners and experienced developers in the field of deep learning. To begin, you need to install PyTorch, which can be easily done using package managers like pip or conda. Once installed, the core concept to understand in PyTorch is the tensor, a multi-dimensional array similar to NumPy arrays, but with the added capability of being processed on a GPU for faster computation. PyTorch’s API is designed to be intuitive and Pythonic, allowing you to quickly perform operations on tensors, such as arithmetic, reshaping, and broadcasting. After familiarizing yourself with tensors, you can move on to building neural networks by leveraging PyTorch’s nn module, which provides a simple way to define and train models. PyTorch’s dynamic computation graph allows you to modify models during runtime, making it easier to debug and experiment with different architectures. Additionally, PyTorch integrates well with popular Python libraries like NumPy and Matplotlib, enabling seamless data manipulation and visualization. With a robust community and extensive documentation, PyTorch provides a comprehensive environment to dive into deep learning projects, from simple experiments to complex research endeavors. Let’s walk through the basics of setting up and using PyTorch in Python.
1. Installing PyTorch
Before you can start using PyTorch, you need to install it. PyTorch can be installed via pip:
pip install torch torchvision
Alternatively, you can install it using conda:
conda install pytorch torchvision torchaudio -c pytorch
2. Tensors: The Building Blocks of PyTorch
Tensors are the fundamental data structure in PyTorch, serving as the building blocks for all computations in deep learning models. Similar to NumPy arrays, tensors are multi-dimensional arrays that can store data in various shapes and sizes, but they come with added capabilities that make them indispensable for machine learning tasks. One of the key advantages of tensors in PyTorch is their ability to leverage GPU acceleration, allowing for faster processing of large datasets and complex operations. Tensors can be easily created from Python lists or existing arrays and can be manipulated through a wide range of operations, including arithmetic, slicing, and matrix multiplication. PyTorch provides a flexible interface to work with tensors, enabling seamless integration with other Python libraries, which makes data preprocessing, transformation, and augmentation straightforward. Furthermore, tensors in PyTorch support automatic differentiation, which is crucial for training neural networks. This means that gradients can be calculated automatically, making backpropagation efficient and easy to implement. Overall, tensors are the core abstraction that powers PyTorch's ability to perform high-performance computations, making them essential for building and training sophisticated machine learning models. Here's a quick overview of how to create and manipulate tensors in PyTorch:
import torch
# Create a tensor from a list
x = torch.tensor([1.0, 2.0, 3.0])
# Create a tensor filled with zeros
y = torch.zeros(3, 3)
# Create a tensor with random values
z = torch.rand(3, 3)
# Basic operations
sum_tensor = x + z
dot_product = torch.dot(x, torch.tensor([4.0, 5.0, 6.0]))
3. Building a Simple Neural Network
Building a Neural Network using PyTorch in Python involves defining a model architecture using the nn.Module class, which allows you to easily create layers like fully connected (linear) layers. After defining the architecture, you specify the forward pass, where the input data flows through the network layers. With the model set up, you can then define a loss function, such as Cross-Entropy Loss, and choose an optimizer like Stochastic Gradient Descent (SGD) to update the model's weights based on the loss. Finally, you train the network by looping through your data, performing forward and backward passes, and adjusting the model's parameters until it learns to make accurate predictions. This process exemplifies the core of deep learning: iteratively improving the model's performance through training. Let's build a simple neural network to classify digits from the MNIST dataset using PyTorch.
Step 1: Load the Dataset
PyTorch provides torchvision for loading and preprocessing datasets. We'll use it to load the MNIST dataset.
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
# Transform to normalize the data
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
# Download and load the training and test datasets
trainset = torchvision.datasets.MNIST(root='./data', train=True, download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
testset = torchvision.datasets.MNIST(root='./data', train=False, download=True, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=64, shuffle=False)
Output for the code above:
Downloading https://ossci-datasets.s3.amazonaws.com/mnist/t10k-labels-idx1-ubyte.gz
Downloading https://ossci-datasets.s3.amazonaws.com/mnist/t10k-labels-idx1-ubyte.gz to ./data/MNIST/raw/t10k-labels-idx1-ubyte.gz
100%|██████████| 4542/4542 [00:00<00:00, 5616311.55it/s]Extracting ./data/MNIST/raw/t10k-labels-idx1-ubyte.gz to ./data/MNIST/raw
Step 2: Define the Neural Network
We'll define a simple neural network with one hidden layer.
class SimpleNN(nn.Module):
def init(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(28 * 28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 28 * 28) # Flatten the image
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
Step 3: Train the Model
We'll use Cross-Entropy Loss and Stochastic Gradient Descent (SGD) to train the model.
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Training loop
for epoch in range(10): # 10 epochs
running_loss = 0.0
for inputs, labels in trainloader:
optimizer.zero_grad() # Zero the parameter gradients
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}, Loss: {running_loss/len(trainloader)}")
Output for the code above:
Epoch 1, Loss: 0.7743404764991834
Epoch 2, Loss: 0.3684149981974793
Epoch 3, Loss: 0.32268288911087933
Epoch 4, Loss: 0.2961855503811892
Epoch 5, Loss: 0.2750281660176162
Epoch 6, Loss: 0.25595922380495173
Epoch 7, Loss: 0.23872971742439753
Epoch 8, Loss: 0.22337837101442853
Epoch 9, Loss: 0.20959772887641687
Epoch 10, Loss: 0.19758689776460117
Step 4: Evaluate the Model
Finally, we’ll evaluate the model on the test dataset.
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in testloader:
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f'Accuracy of the network on the test images: {100 * correct / total}%')
Output for the code above:
Accuracy of the network on the test images: 94.38%
Full Code for Building a Simple Neural Network using PyTorch in Python
The full code for building a simple neural network in PyTorch involves loading your dataset, defining the neural network architecture with nn.Module, specifying a loss function and optimizer, and then training the model through multiple epochs by iterating over the dataset, performing forward and backward passes, and updating the model's parameters. Finally, you evaluate the model's performance on a test dataset to check its accuracy. This code provides a foundation for more complex deep learning projects.
# Import dependencies
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
# Transform to normalize the data
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
# Download and load the training and test datasets
trainset = torchvision.datasets.MNIST(root='./data', train=True, download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
testset = torchvision.datasets.MNIST(root='./data', train=False, download=True, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=64, shuffle=False)
# Neural Network
class SimpleNN(nn.Module):
def init(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(28 * 28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 28 * 28) # Flatten the image
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Training loop
for epoch in range(10): # 10 epochs
running_loss = 0.0
for inputs, labels in trainloader:
optimizer.zero_grad() # Zero the parameter gradients
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}, Loss: {running_loss/len(trainloader)}")
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in testloader:
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f'Accuracy of the network on the test images: {100 * correct / total}%')
Conclusion
In conclusion, PyTorch stands out as a powerful and flexible deep learning framework that has become a favorite among developers and researchers alike. Its dynamic computation graph, ease of use, and seamless integration with Python make it an ideal tool for both experimentation and production-level projects. From understanding the foundational role of tensors to building and training neural networks, PyTorch simplifies the deep learning process, allowing users to focus on innovating and solving complex problems. Whether you're a beginner exploring the basics or an advanced user tackling sophisticated models, PyTorch provides the tools and community support needed to succeed in the ever-evolving field of artificial intelligence.
Comments