Looping is one of the most fundamental programming concepts. In Python, iteration allows you to execute a block of code repeatedly based on certain conditions. Python provides multiple ways to create loops, including for loops, whileloops, and various iteration tools for efficient looping. In this blog, we’ll explore the different looping structures in Python, best practices, and advanced features like loop control statements.
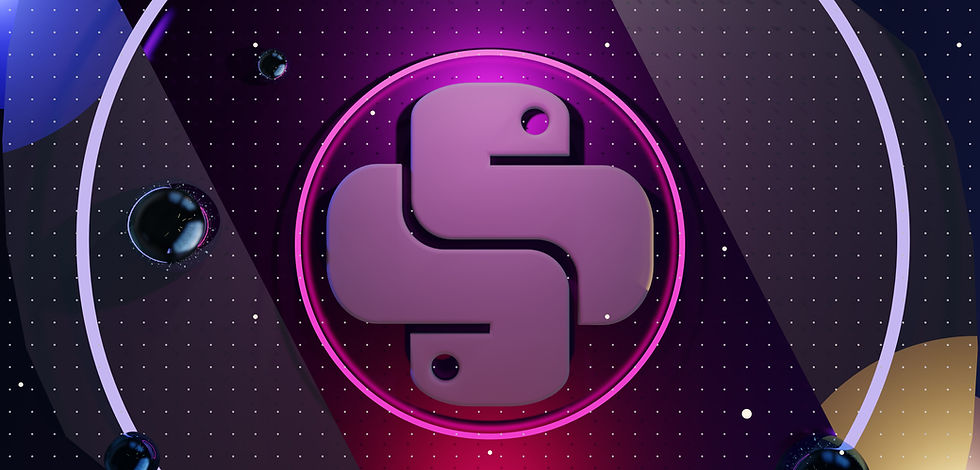
What are Loops in Python?
In Python, loops are used to repeatedly execute a block of code. The two main types are for and while loops. The forloop iterates over a sequence (like lists or strings) and executes code for each item. The while loop continues running as long as a specified condition remains true. Loops can be controlled using break to exit prematurely, continue to skip the current iteration, and else which executes if the loop completes without a break. Loops are essential for tasks like traversing collections or automating repetitive operations.
Types of Loops in Python
Python supports two primary types of loops:
for Loop: Used to iterate over a sequence (like a list, tuple, dictionary, or string).
while Loop: Repeats a block of code as long as a certain condition is true.
1. The for Loop
The for loop in Python is used to iterate over a sequence of items, such as a list, tuple, string, or any iterable object.
Example: Iterating Over a List
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
Example: Iterating Over a String
word = "Python"
for char in word:
print(char)
Output:
P
y
t
h
o
n
2. The while Loop
A while loop continues to execute as long as the given condition is True. When the condition becomes False, the loop stops.
Example: Using a while Loop
count = 0
while count < 5:
print("Count is:", count)
count += 1
Output:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
Count is: 4
The range() Function
Python’s built-in range() function is commonly used with for loops. It generates a sequence of numbers and is particularly useful for iterating a specific number of times.
Syntax:
range(start, stop, step)
start: The starting number of the sequence (inclusive).
stop: The ending number (exclusive).
step: The difference between each number in the sequence (default is 1).
Example: Using range() with a for Loop
for i in range(5):
print(i)
Output:
0
1
2
3
4
Example: Specifying Start, Stop, and Step
for i in range(1, 10, 2):
print(i)
Output:
1
3
5
7
9
Loop Control Statements
Python provides control statements that allow you to modify the behavior of loops.
1. break
The break statement is used to exit a loop prematurely when a certain condition is met.
Example: Using break
for i in range(10):
if == 5:
break
print(i)
Output:
0
1
2
3
4
2. continue
The continue statement skips the current iteration and continues with the next iteration.
Example: Using continue
for i in range(5):
if i == 3:
continue
print(i)
Output:
0
1
2
4
3. else in Loops
Python allows the use of an else block in both for and while loops. The else block is executed when the loop finishes normally (i.e., not interrupted by break).
Example: Using else with a Loop
for i in range(5):
print(i)
else:
print("Loop finished!")
Output:
0
1
2
3
4
Loop finished!
4. pass
The pass statement does nothing and is useful when a statement is syntactically required but you want to leave it empty.
Example: Using pass
for i in range(5):
if i == 3:
pass
print(i)
Output:
0
1
2
3
4
Nested Loops
You can place loops inside other loops, which is referred to as nested loops.
Example: Nested for Loops
for i in range(3):
for j in range(2):
print(f"i = {i}, j = {j}")
Output:
i = 0, j = 0
i = 0, j = 1
i = 1, j = 0
i = 1, j = 1
i = 2, j = 0
i = 2, j = 1
Example: Nested for and while Loop
i = 0
while i < 3:
for j in range(2):
print(f"i = {i}, j = {j}")
i += 1
Output:
i = 0, j = 0
i = 0, j = 1
i = 1, j = 0
i = 1, j = 1
i = 2, j = 0
i = 2, j = 1
Iterating Over Dictionaries
You can iterate over the keys, values, or both in a dictionary using the items(), keys(), and values() methods.
Example: Iterating Over Keys and Values
person = {"name": "Alice", "age": 30, "city": "New York"}
for key, value in person.items():
print(key, ":", value)
Output:
ame : Alice
age : 30
city : New York
Using enumerate() for Indexed Iteration
The enumerate() function is a useful tool when you need to iterate over an iterable and keep track of the index.
Example: Using enumerate()
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits):
print(index, fruit)
Output:
0 apple
1 banana
2 cherry
Using zip() for Parallel Iteration
The zip() function allows you to iterate over multiple sequences in parallel.
Example: Using zip()
names = ["Alice", "Bob", "Charlie"]
ages = [25, 30, 35]
for name, age in zip(names, ages):
print(f"{name} is {age} years old.")
Output:
Alice is 25 years old.
Bob is 30 years old.
Charlie is 35 years old.
Conclusion
Python offers flexible and powerful tools for iteration and looping. Whether you're working with sequences, dictionaries, or custom iterables, Python's loops provide a clear and concise way to perform repetitive tasks. Key points to remember include:
Use for loops for iterating over sequences or collections.
Use while loops when you need to loop based on a condition.
Take advantage of control statements like break, continue, and else to manage the flow of loops.
Use zip(), enumerate(), and nested loops for more complex scenarios.
By mastering these looping techniques, you can efficiently handle various iterative tasks in Python with ease!
Comments