If you’ve been diving into JavaScript, you’ve likely encountered objects everywhere. They’re the unsung heroes of the language, quietly powering everything from simple data structures to full-blown applications. But let’s be honest—objects can feel a bit intimidating at first. What’s a property? A method? And what’s the deal with prototypes?
Don’t worry—you’re not alone. In this guide, we’re going to break it all down in a way that’s clear, approachable, and (dare we say) even fun. You’ll learn what objects are, how to use them, and why they’re such a big deal in JavaScript. Whether you’re just getting started or looking to level up your skills, this is your go-to resource for understanding JavaScript objects. Let’s get started!
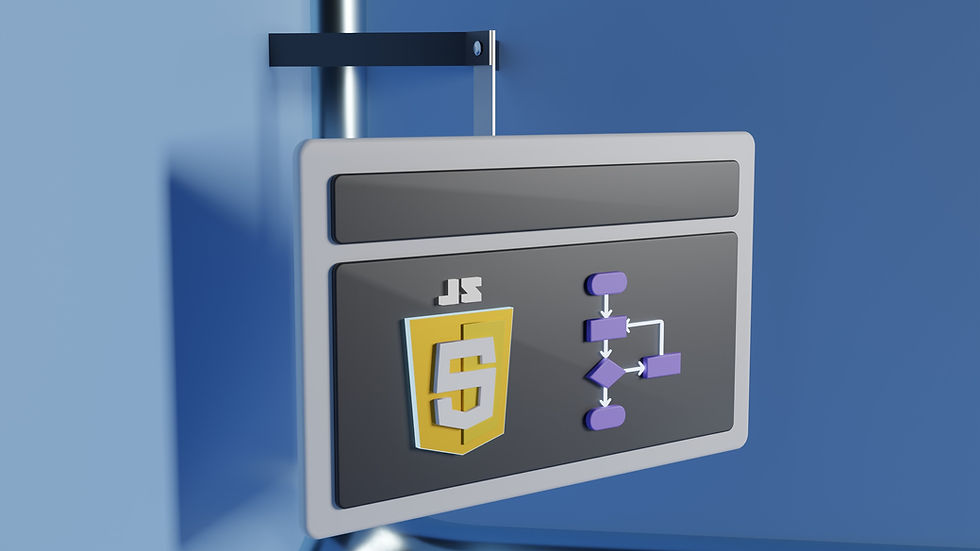
What is an Object in JavaScript?
In JavaScript, an object is a collection of related data and functionality. These are stored in the form of key-value pairs, where the keys are known as properties (when associated with data) and methods (when associated with functions). Objects allow you to group data and behaviors together, making your code more organized and easier to manage.
Example of an Object
const person = {
name: "Alice",
age: 25,
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
Creating Objects in JavaScript
Creating objects is a fundamental aspect of JavaScript, allowing developers to structure and organize their code efficiently. Objects in JavaScript are collections of key-value pairs, where keys are strings (or Symbols) and values can be of any data type, including functions. They enable you to represent real-world entities or logical structures, making your code modular and reusable. Whether using object literals, constructors, or modern classes, understanding how to create and manipulate objects is essential for writing effective JavaScript applications.
1. Using Object Literals
The most common way to create an object:
const person = {
name: "Alice",
age: 30,
greet() {
console.log(`Hello, my name is ${this.name}.`);
}
};
console.log(person.name); // Access property
person.greet(); // Call method
2. Using the new Object() Constructor
This is less common but still valid:
const car = new Object();
car.brand = "Toyota";
car.model = "Corolla";
car.drive = function() {
console.log("Driving the car!");
};
console.log(car.brand); // "Toyota"
car.drive(); // Call method
3. Using a Constructor Function
A reusable way to create objects with similar structures:
function Person(name, age) {
this.name = name;
this.age = age;
this.greet = function() {
console.log(`Hi, I'm ${this.name} and I'm ${this.age} years
old.`);
};
}
const john = new Person("John", 25);
const jane = new Person("Jane", 28);
john.greet(); // "Hi, I'm John and I'm 25 years old."
jane.greet(); // "Hi, I'm Jane and I'm 28 years old."
Accessing Object Properties in JavaScript
In JavaScript, objects are a fundamental way to store and manage data using key-value pairs. Accessing the properties of an object is a common task that allows developers to retrieve or update specific pieces of information. You can access object properties using either dot notation or bracket notation, depending on the context and the property name. Both approaches offer flexibility and are essential for efficient manipulation of objects in JavaScript. Let's dive into how they work and when to use them.
1. Dot Notation
console.log(person.name); // "Alice"
2. Bracket Notation
Useful when the key is dynamic or contains special characters:
javascript
console.log(person["name"]); // "Alice"
Adding, Modifying, and Deleting Properties
When working with objects in programming, properties play a crucial role in defining the characteristics and behaviors of those objects. Adding, modifying, and deleting properties allows developers to dynamically update objects to suit evolving requirements. Whether you're building a simple application or a complex system, these operations provide the flexibility to manage object structures effectively, ensuring adaptability and efficiency in your code.
1. Add a Property
person.job = "Engineer";
console.log(person.job); // "Engineer"
2. Modify a Property
person.age = 31;
console.log(person.age); // 31
3. Delete a Property
delete person.age;
console.log(person.age); // undefined
Object Methods
Methods are functions stored in an object property:
const calculator = {
add(a, b) {
return a + b;
},
subtract(a, b) {
return a - b;
}
};
console.log(calculator.add(5, 3)); // 8
console.log(calculator.subtract(5, 3)); // 2
Checking for Properties in JavaScript
"Checking for Properties" refers to the process of verifying specific characteristics or attributes of an object, data structure, or system. This concept is commonly used in programming, mathematics, and software development to ensure that a given entity meets predefined criteria or exhibits expected behavior. Whether it's validating an object’s type, ensuring a list contains unique elements, or confirming compliance with a protocol, property checks are fundamental for maintaining integrity, functionality, and reliability in a system.
1. Using the in Operator
console.log("name" in person); // true
console.log("height" in person); // false
2. Using hasOwnProperty()
console.log(person.hasOwnProperty("name")); // true
Iterating Over Object Properties
Iterating over object properties is a fundamental aspect of programming in JavaScript, allowing developers to access and manipulate the data stored in an object. JavaScript provides several methods to achieve this, such as for...in loops, Object.keys(), Object.values(), and Object.entries(). Each method offers unique capabilities, from iterating over enumerable properties to extracting key-value pairs for more complex operations. Understanding these techniques is essential for efficiently working with objects and enhancing the versatility of your code.
1. Using for...in
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
2. Using Object.keys()
Returns an array of the object’s keys:
const keys = Object.keys(person);
console.log(keys); // ["name", "job"]
3. Using Object.values()
Returns an array of the object’s values:
const values = Object.values(person);
console.log(values); // ["Alice", "Engineer"]
4. Using Object.entries()
Returns an array of key-value pairs:
const entries = Object.entries(person);
console.log(entries); // [["name", "Alice"], ["job", "Engineer"]]
Cloning and Merging Objects in JavaScript
Cloning and merging objects are fundamental operations in JavaScript, allowing developers to efficiently duplicate or combine data structures. These techniques are essential for managing object immutability, ensuring clean code, and avoiding unintended side effects when manipulating objects. Whether you're working with shallow copies for simple cases or deep copies for nested objects, JavaScript offers versatile methods to handle these tasks. Additionally, merging objects provides a powerful way to consolidate properties from multiple sources into a single cohesive structure.
1. Using Object.assign()
const original = { a: 1, b: 2 };
const clone = Object.assign({}, original);
console.log(clone); // { a: 1, b: 2 }
2. Using the Spread Operator
const clone = { ...original };
console.log(clone); // { a: 1, b: 2 }
3. Merging Objects
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const merged = { ...obj1, ...obj2 };
console.log(merged); // { a: 1, b: 3, c: 4 }
Few Related Concepts in JavaScript
1. Nested Objects
const company = {
name: "TechCorp",
location: {
city: "New York",
country: "USA"
}
};
console.log(company.location.city); // "New York"
2. this Keyword
const user = {
name: "Alice",
greet() {
console.log(`Hi, I'm ${this.name}`);
}
};
user.greet(); // "Hi, I'm Alice"
3. Object Destructuring
const { name, job } = person;
console.log(name, job); // "Alice Engineer"
Conclusion
JavaScript objects are the backbone of dynamic and interactive web development. They provide a powerful way to store, manipulate, and organize data, making them an essential tool for developers of all levels.
By mastering JavaScript objects, you unlock the ability to create robust applications that are both efficient and scalable. Whether you're building a simple form handler or designing a complex web application, understanding objects equips you with the flexibility and tools needed to succeed.
As you continue your JavaScript journey, practice creating and using objects in real-world scenarios. Experiment with advanced concepts like classes, constructors, and Object APIs to deepen your knowledge. With consistent learning and hands-on experience, you'll be well on your way to becoming proficient in harnessing the full potential of JavaScript objects.
Comments