In recent years, the Generative Pre-trained Transformer (GPT) models have gained significant attention for their ability to generate human-like text. GPT, developed by OpenAI, is based on transformer architecture and has been used in various applications, from chatbots to content creation. In this blog, we will explore how to use GPT in Python, focusing on using the transformers library by Hugging Face, which provides easy access to GPT models.
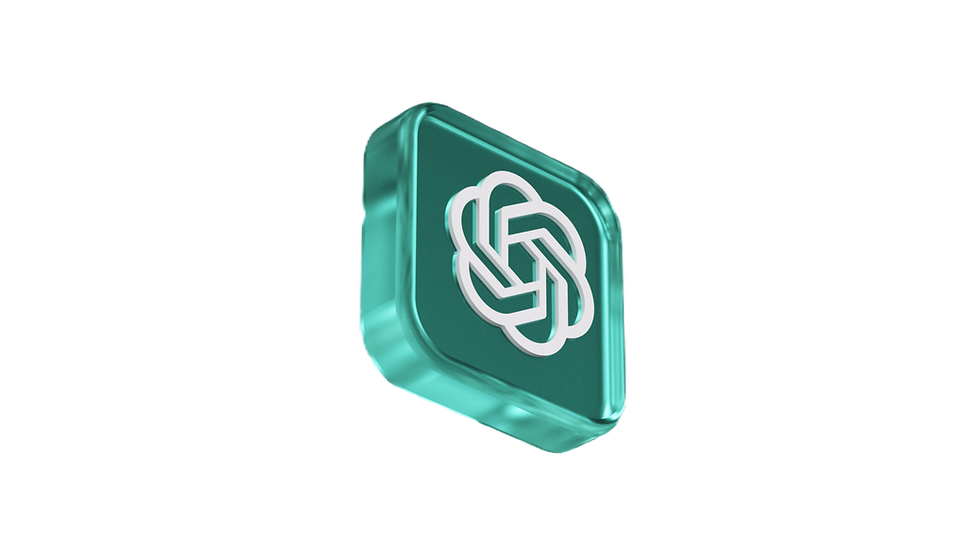
What is GPT (Generative Pre-trained Transformer)?
GPT (Generative Pre-trained Transformer) is a type of deep learning model that has revolutionized the field of natural language processing (NLP). Developed by OpenAI, GPT models are designed to generate human-like text based on a given input. They have been trained on vast amounts of text data from the internet, which allows them to understand and produce text that is coherent, contextually relevant, and often indistinguishable from text written by humans. Key features of GPT include:
Transformer Architecture: GPT is built on the transformer architecture, which is highly effective at handling sequential data such as text. Unlike traditional models that process data in sequence, transformers use self-attention mechanisms to process all words in a sentence simultaneously, allowing for better context understanding.
Pre-training and Fine-tuning: GPT models are first pre-trained on a large corpus of text in an unsupervised manner, learning the general structure and content of the language. They can then be fine-tuned on specific tasks, such as translation, summarization, or sentiment analysis, with relatively small amounts of task-specific data.
Text Generation: One of GPT’s most prominent features is its ability to generate text. Given a prompt, GPT can produce text that continues the input in a coherent and contextually appropriate manner. This makes it useful for a wide range of applications, from creative writing to automated content generation.
Versatility: GPT can be applied to numerous NLP tasks, including text completion, question answering, text classification, and conversation modeling. Its flexibility and adaptability have made it a go-to model for various applications across industries.
Scalability: GPT models have been scaled to different sizes, with the larger versions (such as GPT-3) containing billions of parameters. These larger models tend to perform better on a wider range of tasks but require significant computational resources to train and run.
Getting Started with GPT in Python
To use GPT in Python, we will be using the transformers library by Hugging Face. This library provides pre-trained models and tools for easy integration of GPT into your projects.
1. Installing the Required Libraries
First, you need to install the transformers library along with torch, which is required for running the model.
pip install transformers torch
2. Loading a Pre-trained GPT Model
Once the libraries are installed, you can load a pre-trained GPT model. We will use the GPT-2 model, in this tutorial.
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load pre-trained GPT-2 model and tokenizer
model_name = 'gpt2'
model = GPT2LMHeadModel.from_pretrained(model_name)
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
Output for the above code:
generation_config.json: 100%
124/124 [00:00<00:00, 1.73kB/s]
3. Generating Text with GPT
With the model and tokenizer loaded, you can now generate text. Here's a simple example of how to do it:
# Encode input text
input_text = "Once upon a time"
input_ids = tokenizer.encode(input_text, return_tensors='pt')
# Generate text continuation
output = model.generate(input_ids, max_length=50, num_return_sequences=1)
# Decode and print the generated text
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
Output for the above code:
Once upon a time, the world was a place of great beauty and great danger. The world was a place of great danger, and the world was a place of great danger. The world was a place of great danger
In this example, the model takes the input text "Once upon a time" and generates a continuation of the story.
4. Fine-tuning GPT for Specific Tasks
While GPT-2 can generate general text, you might want to fine-tune it for specific tasks, such as generating poetry or code. Fine-tuning involves training the model on a custom dataset, which can be done using the transformers library as well.
Fine-tuning requires more advanced knowledge and computational resources, but for many applications, the pre-trained model is sufficient.
Full Code for Generating text with GPT in Python
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load pre-trained GPT-2 model and tokenizer
model_name = 'gpt2'
model = GPT2LMHeadModel.from_pretrained(model_name)
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
# Encode input text
input_text = "Once upon a time"
input_ids = tokenizer.encode(input_text, return_tensors='pt')
# Generate text continuation
output = model.generate(input_ids, max_length=50, num_return_sequences=1)
# Decode and print the generated text
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
Use Cases for GPT
GPT models have become highly versatile tools across various industries due to their ability to generate coherent and contextually relevant text. Here are some prominent use cases for GPT:
1. Chatbots and Conversational Agents
GPT can power chatbots that engage in natural, human-like conversations. These chatbots can be used in customer service, virtual assistants, and interactive user interfaces. For example, companies can deploy GPT-based chatbots on their websites to answer customer inquiries, provide product recommendations, and even handle complex queries.
2. Content Creation and Copywriting
One of the most popular uses of GPT is in generating written content. It can create articles, blog posts, social media updates, and product descriptions. Content creators can use GPT to draft initial versions of their work, brainstorm ideas, or even produce entire pieces of content that require minimal editing.
3. Code Generation and Programming Assistance
Developers can leverage GPT to assist with coding tasks. The model can generate code snippets based on given prompts, help debug code, or provide suggestions for functions and algorithms. Tools like GitHub Copilot use GPT-like models to assist developers directly in their Integrated Development Environments (IDEs).
4. Text Summarization
GPT can be used to summarize long documents, articles, or reports into concise versions. This is particularly useful for news aggregation, academic research, and business reporting, where users need quick overviews of lengthy texts.
5. Language Translation
While GPT is not specifically designed for translation, it can be fine-tuned to perform this task. By leveraging its language modeling capabilities, GPT can translate text between different languages, providing a flexible solution for multilingual communication.
6. Creative Writing and Storytelling
Authors and creatives can use GPT to generate poetry, short stories, or even full-length novels. GPT's ability to produce imaginative and contextually appropriate text makes it a valuable tool for overcoming writer's block or exploring new narrative possibilities.
7. Educational Tools and Tutoring
GPT can be employed to create educational content, answer student queries, or even generate practice problems. It can serve as a virtual tutor, providing explanations, solving problems, and guiding students through complex topics in a conversational manner.
8. Marketing and Advertising
In marketing, GPT can be used to generate engaging ad copy, personalized email campaigns, and social media content. It can analyze customer behavior and preferences to create targeted messaging that resonates with specific audiences.
9. Research and Data Analysis
Researchers can use GPT to analyze large datasets of text, extract key insights, and generate summaries. It can also assist in the literature review process by identifying relevant papers and generating concise overviews of the findings.
10. Gaming and Interactive Fiction
In the gaming industry, GPT can be used to create dynamic narratives, generate dialogues for characters, or even design entire game worlds. Interactive fiction platforms can integrate GPT to offer players personalized and evolving storylines based on their choices.
11. Legal Document Drafting
Lawyers and legal professionals can use GPT to draft contracts, agreements, and other legal documents. It can also assist in reviewing documents by identifying key clauses and summarizing lengthy legal texts.
12. Medical and Healthcare Applications
In healthcare, GPT can assist in generating patient reports, summarizing medical literature, and even supporting diagnostic processes by providing relevant medical information based on symptoms or conditions.
13. Social Media Management
GPT can automate the generation of social media content, helping brands maintain an active online presence. It can create posts, respond to comments, and even engage with followers, ensuring consistent and timely communication.
14. Virtual Reality (VR) and Augmented Reality (AR)
In VR and AR applications, GPT can be used to create immersive storytelling experiences. It can generate dialogues for virtual characters or provide real-time narrative adjustments based on user interactions within virtual environments.
Conclusion
GPT models, particularly GPT-2, offer powerful tools for text generation in Python. With the transformers library, integrating GPT into your projects is straightforward and can be adapted to a wide range of applications. he versatility of GPT models makes them suitable for a wide range of applications across industries. Whether it's enhancing customer interaction, streamlining content creation, or assisting with complex tasks like programming and legal document drafting, GPT offers powerful solutions that can be customized to meet specific needs. As these models continue to evolve, their potential applications are likely to expand even further, offering new opportunities for innovation and efficiency in both professional and creative endeavors.
By experimenting with different parameters and fine-tuning, you can tailor GPT to meet your specific needs, unlocking the full potential of this cutting-edge technology.
Comments