Python's simplicity and readability make it a favorite for beginners, but it is equally robust for advanced developers. Mastering Python's advanced topics can significantly enhance your skills, enabling you to write efficient, scalable, and maintainable code. This blog explores some advanced Python topics to help you take your programming expertise to the next level.
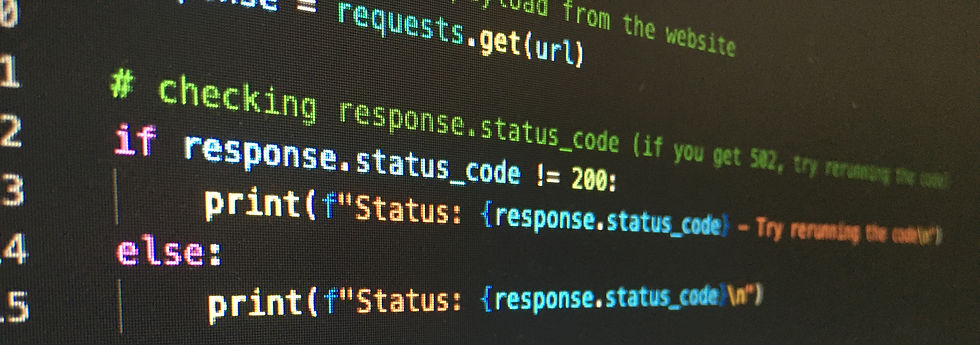
1. Metaclasses and Custom Classes
Metaclasses are the "classes of classes" in Python. They allow you to customize class creation and behavior dynamically. By mastering metaclasses, you can:
Add validations or constraints during class creation.
Automatically register classes or add attributes.
Example:
class Meta(type):
def __new__(cls, name, bases, dct):
if 'required_attr' not in dct:
raise TypeError(f"{name} must have 'required_attr'")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
required_attr = True
2. Decorators and Context Managers
Decorators are powerful for modifying the behavior of functions or methods. They are widely used in frameworks like Flask and Django.
Example:
def logger(func):
def wrapper(*args, **kwargs):
print(f"Executing {func.__name__} with args: {args} kwargs: {kwargs}")
return func(*args, **kwargs)
return wrapper
@logger
def add(a, b):
return a + b
print(add(2, 3)) # Logs the function call and returns 5
Output:
Executing add with args: (2, 3) kwargs: {}
5
Context Managers
Use context managers for managing resources like file handling, database connections, or threading.
Example:
from contextlib import contextmanager
@contextmanager
def managed_resource(name):
print(f"Allocating {name}")
yield
print(f"Deallocating {name}")
with managed_resource("resource"):
print("Using resource")
Output:
Allocating resource
Using resource
Deallocating resource
3. Asynchronous Programming
Python's asyncio module enables concurrent code execution for I/O-bound tasks without multi-threading.
import asyncio
async def fetch_data():
print("Fetching data...")
await asyncio.sleep(2)
print("Data fetched")
async def main():
await asyncio.gather(fetch_data(), fetch_data())
# For environments with an active event loop (like Jupyter), use `await main()`
await main()
Output:
Fetching data...
Fetching data...
Data fetched
Data fetched
4. Generators and Coroutines
Generators produce items one at a time using yield, making them memory-efficient.
Example:
def count_up_to(max):
n = 1
while n <= max:
yield n
n += 1
for num in count_up_to(5):
print(num)
Output:
1
2
3
4
5
Coroutines
Coroutines are a special type of generator that can accept input and yield multiple values.
5. Type Annotations and Static Typing
Type hints, introduced in Python 3.5, improve code readability and facilitate tools like mypy for type checking.
Example:
from typing import List
def average(nums: List[int]) -> float:
return sum(nums) / len(nums)
6. Cython and Python C-API
Cython
Cython is a superset of Python that allows you to compile Python code into C for performance gains.
Python C-API
For extreme performance tuning, you can extend Python with C using its API.
7. Design Patterns in Python
Understanding and implementing design patterns like Singleton, Factory, and Observer can help solve complex problems elegantly.
Example of Singleton:
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if not cls._instance:
cls._instance = super().__new__(cls, *args, **kwargs)
return cls._instance
8. Advanced Data Structures
Leverage Python’s libraries like collections, heapq, and deque for specialized data structures.
Example:
from collections import deque
queue = deque(["a", "b", "c"])
queue.append("d")
print(queue.popleft()) # Outputs: 'a'
9. Testing and Mocking
Learn advanced testing techniques using libraries like unittest and pytest. Mocking external dependencies can help you test edge cases.
Example with Mocking:
from unittest.mock import MagicMock
service = MagicMock()
service.get_data.return_value = {"id": 1, "name": "test"}
print(service.get_data()) # Outputs: {'id': 1, 'name': 'test'}
10. Machine Learning and Data Science
Python is the go-to language for machine learning and data science. Mastering libraries like TensorFlow, PyTorch, and Pandas enables you to create sophisticated ML models.
Example with Pandas:
import pandas as pd
df = pd.DataFrame({"Name": ["Alice", "Bob"], "Age": [25, 30]})
print(df.describe())
Output:
Age
count 2.000000
mean 27.500000
std 3.535534
min 25.000000
25% 26.250000
50% 27.500000
75% 28.750000
max 30.000000
Conclusion
Mastering advanced Python topics requires consistent practice and an eagerness to explore the language's depths. Start small, experiment with these concepts, and integrate them into your projects. With time, you'll be well-equipped to tackle complex challenges and write Python code like a pro!
Are you looking to enhance your Python skills further? Contact us for mentorship programs, project guidance, and freelance support tailored to your needs!
Comments