Binary search is a powerful and efficient algorithm used to find a target value within a sorted array or list. By repeatedly dividing the search interval in half, binary search reduces the number of comparisons needed to find the target, making it significantly faster than linear search for large datasets. In this blog, we'll delve into the binary search algorithm, its implementation in Python, and its applications.
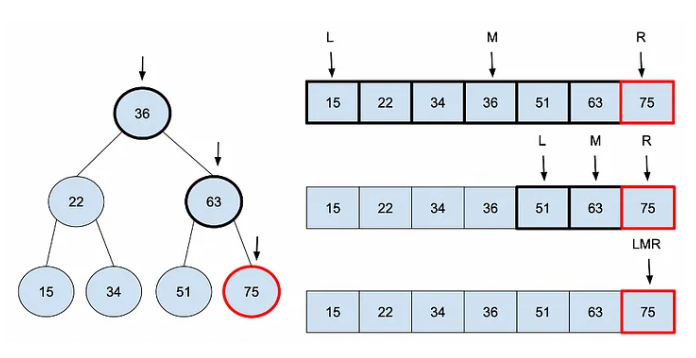
What is Binary Search?
Binary search is an algorithm that operates on a sorted array or list. It works by dividing the search range in half, comparing the target value with the middle element, and then deciding which half of the range to continue the search in. This process is repeated until the target is found or the search range is empty.
Key Concepts
Sorted Array: Binary search requires the array or list to be sorted in ascending order.
Divide and Conquer: The algorithm reduces the problem size by half with each step.
Efficiency: Binary search operates in O(log n) time complexity, making it much more efficient than O(n) algorithms for large datasets.
Binary Search - Algorithm Steps
Initialization: Define the start and end indices of the search range.
Loop:
Calculate the middle index of the current search range.
Compare the middle element with the target value.
Adjust the search range based on whether the target is less than or greater than the middle element.
Termination: The search ends when the target is found or the search range is exhausted.
Binary Search in Python - Implementation
Let’s implement binary search in Python. We’ll create a function that performs binary search on a sorted list and returns the index of the target value.
Initialization: We define the left and right pointers to represent the search range, initially set to the start and end of the list, respectively.
Loop: In each iteration, we calculate the middle index and compare the middle element with the target value:
If they match, we return the middle index.
If the target is greater than the middle element, we adjust the left pointer to search the right half.
If the target is less than the middle element, we adjust the right pointer to search the left half.
Termination: The loop continues until left exceeds right, indicating that the target is not in the list, and we return -1.
Full Code for Binary Search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
# Check if the target is present at mid
if arr[mid] == target:
return mid
# If target is greater, ignore left half
elif arr[mid] < target:
left = mid + 1
# If target is smaller, ignore right half
else:
right = mid - 1
# Target is not present in the array
return -1
# Example usage
sorted_list = [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
target_value = 7
index = binary_search(sorted_list, target_value)
if index != -1:
print(f"Target found at index {index}.")
else:
print("Target not found in the list.")
Output for the code above:
Target found at index 3.
Applications Binary Search
Searching in Databases: Binary search is used in database indexing to quickly locate records.
Algorithmic Problems: Commonly used in competitive programming and algorithm design for efficient search operations.
Software Engineering: Applied in various applications where fast search operations on sorted data are required.
Conclusion
Binary search is a fundamental algorithm that efficiently finds elements in a sorted array or list by leveraging the divide-and-conquer strategy. Its logarithmic time complexity makes it a valuable tool in scenarios where performance is critical. By understanding and implementing binary search in Python, you can enhance your problem-solving skills and optimize search operations in your applications.
Comments