In this blog, we’ll explore why promises are so important, how they work, and how you can use them to write more readable, maintainable, and error-resistant code. Plus, I’ll walk you through several examples so you can see how useful they really are in real-world scenarios.
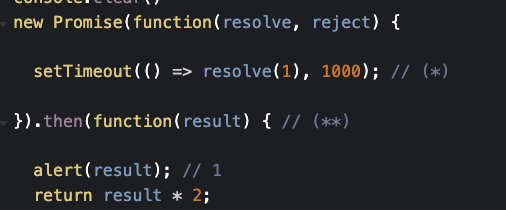
What Exactly is a Promise in JavaScript?
If you’ve been coding in JavaScript for a while, you’ve probably run into situations where you need to wait for something to happen before moving on—like waiting for data to load from an API or for a file to be read. In the early days, developers used callbacks to handle this, but that often led to messy, hard-to-read code. Enter Promises—a game-changer in the world of JavaScript that makes dealing with asynchronous tasks so much easier and cleaner.A Promise is essentially an object that represents the eventual outcome of an asynchronous operation. It’s like saying, “I promise I’ll get this done—either I’ll complete it successfully, or something will go wrong, but either way, you’ll know when I’m done.”
A promise can be in one of three states:
Pending: The initial state—it's still in progress.
Fulfilled: It finished successfully.
Rejected: Something went wrong.
Promises help avoid what’s known as “callback hell,” where you end up nesting multiple callbacks inside each other, leading to deeply indented, hard-to-read code.
Why Should You Care About Promises?
1. Avoiding Callback Hell
Before promises, developers handled asynchronous operations using callbacks, but that led to this kind of messy code:
doSomething(function(result) {
doSomethingElse(result, function(newResult) {
doAnotherThing(newResult, function(finalResult) {
console.log('Final result:', finalResult);
});
});
});
This quickly becomes a nightmare to read and maintain. But with promises, you can flatten this out beautifully:
doSomething()
.then(result => doSomethingElse(result))
.then(newResult => doAnotherThing(newResult))
.then(finalResult => console.log('Final result:', finalResult))
.catch(error => console.error('Error:', error));
Look at how much cleaner that is! By chaining .then() methods, you avoid the deep nesting, making the flow much easier to follow.
2. Cleaner Error Handling
With callbacks, error handling can get messy because you have to handle errors at every level of nesting. Promises solve this by letting you handle all errors in one place with .catch().
fetchData()
.then(data => processData(data))
.then(result => saveData(result))
.catch(error => console.error('An error occurred:', error));
This way, if anything goes wrong at any step, the .catch() will handle it. It keeps your error-handling logic in one spot, making your code cleaner and more organized.
3. More Readable Code
Promises allow you to write asynchronous code that reads almost like synchronous code, which makes it easier to understand and maintain.
getUser()
.then(user => getOrders(user.id))
.then(orders => getOrderDetails(orders[0].id))
.then(details => console.log('Order Details:', details))
.catch(error => console.error('Error:', error));
This chain is so much easier to follow than a series of nested callbacks. You can clearly see the flow of the program, making it easier to read and debug.
How to Create and Use Promises
You can create a promise using the new Promise() constructor. It takes a function with two arguments: resolve and reject. Here’s a simple example:
const promise = new Promise((resolve, reject) => {
const success = true;
if (success) {
resolve('Operation successful!');
} else {
reject('Operation failed.');
}
});
promise
.then(result => console.log(result))
.catch(error => console.error(error));
Output:
Operation successful!
Here’s what’s happening:
resolve() is called if the operation is successful, moving the promise to the Fulfilled state.
reject() is called if something goes wrong, moving it to the Rejected state.
This allows you to handle success and error cases separately in a clean, organised way.
Real-World Examples
1. Fetching Data from an API
One of the most common uses of promises is with the Fetch API, which returns a promise.
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(posts => console.log('Posts:', posts))
.catch(error => console.error('Fetch error:', error));
Here’s what’s happening:
The first .then() checks if the response is okay.
The second .then() parses the JSON data.
.catch() handles any network or processing errors in one place.
2. Chaining Promises
You can chain promises to handle multiple asynchronous operations in sequence.
new Promise(function(resolve, reject) {
setTimeout(() => resolve(1), 1000); // (*)
}).then(function(result) { // (**)
alert(result); // 1
return result * 2;
}).then(function(result) { // (***)
alert(result);
return result * 2;
}).then(function(result) {
alert(result); // 4
return result * 2;
});
The idea is that the result is passed through the chain of .then handlers.
Here the flow is:
The initial promise resolves in 1 second (*),
Then the .then handler is called (**), which in turn creates a new promise (resolved with 2 value).
The next then (***) gets the result of the previous one, processes it (doubles) and passes it to the next handler.
…and so on.
3. Running Multiple Promises Simultaneously
You can use Promise.all() to run multiple promises in parallel and wait for all of them to complete:
const promise1 = fetch('https://jsonplaceholder.typicode.com/users').then(res => res.json());
const promise2 = fetch('https://jsonplaceholder.typicode.com/posts').then(res => res.json());
Promise.all([promise1, promise2])
.then(results => {
console.log('Users:', results[0]);
console.log('Posts:', results[1]);
})
.catch(error => console.error('Error:', error));
This is super handy when you need to wait for multiple independent asynchronous tasks to complete.
Conclusion
Promises have fundamentally changed how JavaScript handles asynchronous operations. They offer:
Cleaner and more readable code by avoiding callback hell.
Centralized error handling.
The ability to run asynchronous tasks in sequence or concurrently.
They also paved the way for async and await, which build on promises to make asynchronous code even easier to write and understand. If you’re serious about writing modern JavaScript, mastering promises is a must.
So go ahead, start using promises in your projects, and see how they make your life as a developer so much easier!
Comments