Metaclasses can seem like a challenging topic at first, but they offer a lot of power and flexibility when defining how Python classes behave. Let's break this down step by step and focus on metaclasses and custom classes in Python.
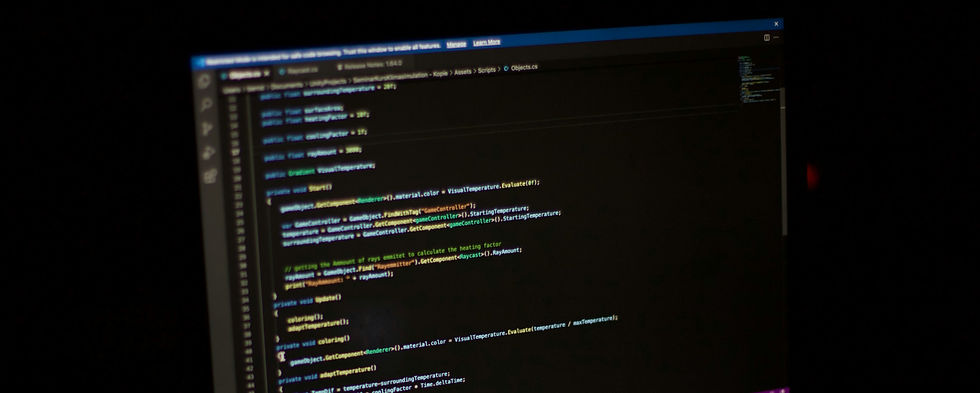
What is a Metaclass in Python?
Metaclasses in Python are a powerful mechanism for defining the behavior of classes themselves. While classes are used to create objects, metaclasses are the "class of a class," responsible for creating and controlling how classes behave. By default, Python uses the built-in type as the metaclass, which handles the creation of new classes. However, developers can define custom metaclasses by subclassing type to modify or extend this process. This allows for enforcing rules, adding methods or attributes, or automatically altering the structure of a class during its creation. Metaclasses achieve this by overriding special methods like new or init, which provide hooks to modify the class definition or perform validation. Although metaclasses are not commonly used, they are invaluable when working on advanced tasks like creating frameworks or APIs that need to standardize or dynamically manipulate class behavior.
When to Use a Metaclass?
You should use a metaclass in Python when you need to customize or control the creation and behavior of classes themselves, rather than their instances. Metaclasses are especially useful for enforcing rules or constraints on class definitions, such as ensuring certain naming conventions for methods or attributes, automatically injecting new methods or properties, or modifying inheritance hierarchies. They are also valuable in frameworks or libraries where you want to provide additional functionality or validation at the class level without requiring explicit changes in each class. For instance, you might use a metaclass to log the creation of all classes, implement a singleton pattern, or dynamically register classes in a central registry for later use. While metaclasses are powerful, they should be used sparingly and only when simpler approaches like decorators or class inheritance cannot achieve the desired level of customization.
To enforce constraints or rules on class definitions.
To automatically modify or enhance classes as they are created.
To implement custom behaviors for a family of classes.
Basic Components of a Metaclass
A metaclass in Python is a class that defines how other classes behave. It is essentially the "class of a class," dictating the creation and customization of class objects. The basic components of a metaclass include new, init, and optionally call. The new method is invoked before the class is created, allowing modifications to the class's attributes and structure during its creation. The init method is called after the class is created, enabling additional initialization or validation of the class itself. Metaclasses inherit from Python's built-in type, which is the default metaclass used when creating classes. By overriding these methods, developers can enforce specific rules, modify behaviors, or dynamically add functionality to classes, providing a powerful way to control and customize class definitions.
type: The default metaclass in Python. When you create a class, Python internally uses type to construct it.
Custom Metaclass: You can create your own metaclass by subclassing type.
How Metaclasses Work
Metaclasses in Python determine how classes are created and behave, functioning as the blueprint for classes themselves. When Python encounters a class statement, it uses a metaclass to construct the class. By default, the built-in type metaclass is used, but developers can specify a custom metaclass by including the metaclass keyword in the class definition. The metaclass controls the process of class creation through two main stages:
new: This method is called first and is responsible for creating the class object. It receives the name of the class, its parent classes (bases), and the dictionary of attributes (dct) defined within the class body. Developers can use new to modify or validate these attributes before the class is created.
init: After new has created the class, init initializes it. This step allows further configuration of the class itself but does not impact the attributes or structure defined in new.
Metaclasses essentially act as factories for classes, enabling dynamic modifications such as adding methods, enforcing naming conventions, or injecting behavior. The resulting classes are then used to instantiate objects, bridging the relationship between metaclasses, classes, and instances
Example: Custom Metaclass
Here’s an example where a metaclass ensures all class attribute names are uppercase:
# Define the metaclass
class UpperCaseAttributesMeta(type):
def __new__(cls, name, bases, dct):
uppercase_attributes = {
key.upper(): value for key, value in dct.items() if not key.startswith('__')
}
return super().__new__(cls, name, bases, uppercase_attributes)
# Define a class using this metaclass
class MyClass(metaclass=UpperCaseAttributesMeta):
my_attribute = "value"
another_attribute = 42
# Check the result
print(MyClass.MY_ATTRIBUTE) # Output: value
print(MyClass.ANOTHER_ATTRIBUTE) # Output: 42
Combining Metaclasses with Custom Classes
You can use metaclasses to dynamically add methods, validate class definitions, or even change inheritance hierarchies.
Example: Adding a Custom Method Dynamically
class MethodInjectorMeta(type):
def __new__(cls, name, bases, dct):
dct['dynamic_method'] = lambda self: f"This is a dynamic method in {name}"
return super().__new__(cls, name, bases, dct)
class CustomClass(metaclass=MethodInjectorMeta):
pass
obj = CustomClass()
print(obj.dynamic_method()) # Output: This is a dynamic method in CustomClass
Combining metaclasses with custom classes allows developers to dynamically modify the behavior or structure of classes at creation time, providing powerful control over how the classes behave. When a class is defined with a specific metaclass (using the metaclass keyword), the metaclass’s logic is applied during the class creation process. This interaction is particularly useful for tasks like adding methods, validating class attributes, enforcing naming conventions, or even altering inheritance hierarchies dynamically. For instance, a metaclass can inject methods into a custom class. Here’s how this works step-by-step:
The custom class specifies a metaclass.
The metaclass’s new method intercepts the class creation, inspects or modifies the attributes and methods defined in the class body, and returns the finalized class.
The resulting class is then initialized using the metaclass’s init, allowing further customization if needed.
class AddMethodMeta(type):
def __new__(cls, name, bases, dct):
# Dynamically add a method to the class
dct['dynamic_method'] = lambda self: f"This method was added by {cls.__name__}"
return super().__new__(cls, name, bases, dct)
# Custom class using the metaclass
class CustomClass(metaclass=AddMethodMeta):
pass
# Create an instance of the custom class
obj = CustomClass()
print(obj.dynamic_method()) # Output: This method was added by AddMethodMeta
In this example, the metaclass AddMethodMeta injects a dynamic_method into the CustomClass. This highlights the synergy between metaclasses and custom classes, showcasing how metaclasses can enhance class functionality seamlessly.
Conclusion
Metaclasses are a powerful feature in Python that allow developers to control and customize the behavior of classes at creation time. By acting as the "class of a class," they provide the means to dynamically modify, validate, or enhance classes in ways that standard class definitions cannot achieve. When combined with custom classes, metaclasses enable functionality like injecting methods, enforcing rules, or altering inheritance hierarchies, making them highly useful for advanced use cases. While they are not commonly required in everyday programming, understanding metaclasses opens the door to deeper insights into Python's object model and its flexibility. By mastering this concept, developers can write more expressive and maintainable code, especially in scenarios involving complex frameworks or libraries.
Comments