Natural Language Processing (NLP) is an exciting field of Artificial Intelligence that involves the interaction between computers and human languages. Whether you're analyzing sentiment in social media, building chatbots, or creating text classifiers, NLP is a key technology. One of the most widely used libraries in Python for NLP is the Natural Language Toolkit, or NLTK.
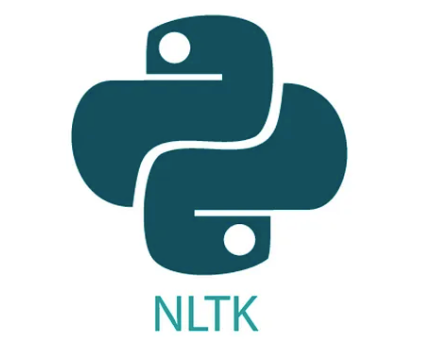
What is Natural Language Toolkit (NLTK) in Python?
The Natural Language Toolkit (NLTK) is a comprehensive and widely-used Python library for working with human language data, also known as natural language processing (NLP). Designed for both novice and experienced developers, NLTK offers a suite of tools and resources that facilitate various NLP tasks, such as tokenization, stemming, lemmatization, part-of-speech tagging, and text classification. It also provides access to a vast array of text corpora and lexical resources like WordNet. NLTK's ease of use, extensive documentation, and powerful capabilities make it an essential tool for those looking to explore or advance in the field of NLP, whether for research, academic purposes, or real-world applications like sentiment analysis, chatbots, and language modeling.
Installing Natural Language Toolkit (NLTK) in Python
Before you can start using NLTK, you'll need to install it. You can do this using pip:
Natural Language Toolkit (NLTK) in Python
Once installed, you'll also want to download the necessary datasets and models. This can be done using the following commands in Python:
import nltk
nltk.download('all')
Downloading all resources might take some time, but it's recommended if you're new to the library. You can also download specific datasets or models later as needed.
Key Features of Natural Language Toolkit (NLTK) in Python
The Natural Language Toolkit (NLTK) in Python is a comprehensive library designed to make the processing and analysis of textual data both accessible and powerful. Among its key features are tokenization, which breaks down text into individual words or sentences, and stop words removal, which filters out common words that don't contribute much meaning. NLTK also offers stemming and lemmatization tools that reduce words to their root forms, making it easier to analyze the core meaning of text. Another essential feature is Part of Speech (POS) tagging, which assigns grammatical categories like nouns and verbs to each word, enabling deeper linguistic analysis. Additionally, Named Entity Recognition (NER) allows users to identify and categorize entities like names, dates, and locations within a text. NLTK's robust text classification capabilities, including built-in algorithms for tasks like sentiment analysis and topic categorization, further enhance its utility, making it an indispensable tool for anyone working in Natural Language Processing.
Tokenization with NLTK
Tokenization is the process of breaking text into smaller pieces, such as words or sentences. NLTK provides simple functions to do this:
from nltk.tokenize import word_tokenize, sent_tokenize
text = "Hello! Welcome to the world of Natural Language Processing with NLTK. It's amazing, isn't it?"
# Tokenize into words
words = word_tokenize(text)
print(words)
# Tokenize into sentences
sentences = sent_tokenize(text)
print(sentences)
Output for the above code:
['Hello', '!', 'Welcome', 'to', 'the', 'world', 'of', 'Natural', 'Language', 'Processing', 'with', 'NLTK', '.', 'It', "'s", 'amazing', ',', 'is', "n't", 'it', '?']
['Hello!', 'Welcome to the world of Natural Language Processing with NLTK.', "It's amazing, isn't it?"]
Stop Words Removal with NLTK
Stop words are common words like "and", "the", "is" that usually do not carry much meaning. Removing them can help in focusing on the important words in a text.
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
print(filtered_words)
Output for the above code:
['Hello', '!', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'NLTK', '.', "'s", 'amazing', ',', "n't", '?']
Stemming and Lemmatization with NLTK
Stemming and lemmatization are techniques to reduce words to their root form. For example, "running" becomes "run".
from nltk.stem import PorterStemmer, WordNetLemmatizer
ps = PorterStemmer()
lemmatizer = WordNetLemmatizer()
stemmed_words = [ps.stem(word) for word in filtered_words]
lemmatized_words = [lemmatizer.lemmatize(word) for word in filtered_words]
print("Stemmed Words:", stemmed_words)
print("Lemmatized Words:", lemmatized_words)
Output for the above code:
Stemmed Words: ['hello', '!', 'welcom', 'world', 'natur', 'languag', 'process', 'nltk', '.', "'s", 'amaz', ',', "n't", '?']
Lemmatized Words: ['Hello', '!', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'NLTK', '.', "'s", 'amazing', ',', "n't", '?']
Part of Speech Tagging (POS Tagging) with NLTK
POS tagging involves labeling words with their corresponding part of speech, like nouns, verbs, adjectives, etc.
from nltk import pos_tag
pos_tags = pos_tag(filtered_words)
print(pos_tags)
Output for the above code:
[('Hello', 'NN'), ('!', '.'), ('Welcome', 'NNP'), ('world', 'NN'), ('Natural', 'NNP'), ('Language', 'NNP'), ('Processing', 'NNP'), ('NLTK', 'NNP'), ('.', '.'), ("'s", 'POS'), ('amazing', 'NN'), (',', ','), ("n't", 'RB'), ('?', '.')]
Named Entity Recognition (NER) with NLTK
Named Entity Recognition identifies entities like names of people, organizations, locations, dates, etc., in the text.
from nltk import ne_chunk
ner_tags = ne_chunk(pos_tags)
print(ner_tags)
Output for the above code:
(S
(GPE Hello/NN)
!/.
Welcome/NNP
world/NN
Natural/NNP
Language/NNP
Processing/NNP
NLTK/NNP
./.
's/POS
amazing/NN
,/,
n't/RB
?/.)
Text Classification with NLTK
NLTK also provides tools to build text classifiers. Here's a simple example using NLTK’s built-in movie review dataset:
from nltk.corpus import movie_reviews
import random
import nltk
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
random.shuffle(documents)
all_words = nltk.FreqDist(w.lower() for w in movie_reviews.words())
word_features = list(all_words)[:2000]
def document_features(document):
document_words = set(document)
features = {}
for word in word_features:
features[f'contains({word})'] = (word in document_words)
return features
featuresets = [(document_features(d), c) for (d, c) in documents]
train_set, test_set = featuresets[100:], featuresets[:100]
classifier = nltk.NaiveBayesClassifier.train(train_set)
print(nltk.classify.accuracy(classifier, test_set))
classifier.show_most_informative_features(5)
Output for the above code:
0.77
Most Informative Features
contains(outstanding) = True pos : neg = 10.9 : 1.0
contains(mulan) = True pos : neg = 9.0 : 1.0
contains(seagal) = True neg : pos = 8.2 : 1.0
contains(wonderfully) = True pos : neg = 6.7 : 1.0
contains(damon) = True pos : neg = 5.9 : 1.0
Applications of NLTK in Python
NLTK (Natural Language Toolkit) is a powerful library in Python that supports a wide range of Natural Language Processing (NLP) tasks. Here are some key applications of NLTK in Python:
1. Text Preprocessing
Tokenization: Breaking down text into words or sentences.
Stop Words Removal: Filtering out common words that do not add much meaning to the text.
Stemming and Lemmatization: Reducing words to their base or root form.
2. Text Classification
Spam Detection: Classifying emails or messages as spam or not spam based on their content.
Sentiment Analysis: Determining the sentiment expressed in a text, whether positive, negative, or neutral.
Topic Classification: Categorizing text documents into predefined topics.
3. Named Entity Recognition (NER)
Identifying and classifying entities in text into categories such as names of people, organizations, locations, dates, etc.
4. Part of Speech (POS) Tagging
Assigning parts of speech to each word in a text, such as nouns, verbs, adjectives, etc.
5. Text Parsing and Syntax Analysis
Dependency Parsing: Analyzing the grammatical structure of a sentence and establishing relationships between "head" words and words which modify those heads.
Context-Free Grammar Parsing: Parsing text based on predefined grammatical rules.
6. Text Summarization
Automatically generating a concise summary of a longer text while preserving key information.
7. Language Modeling
Creating probabilistic models to predict the next word in a sequence, which is useful in applications like text generation and autocomplete.
8. Machine Translation
Translating text from one language to another using statistical or rule-based approaches.
9. Speech Recognition and Generation
Converting spoken language into text (speech recognition) and generating speech from text (text-to-speech).
10. Building Chatbots
Developing conversational agents that can engage in dialogue with users by understanding and generating human language.
11. Information Retrieval
Extracting relevant information from large datasets, such as retrieving documents containing certain keywords from a corpus.
12. Text Mining
Extracting meaningful patterns and knowledge from unstructured text data, which is useful in domains like bioinformatics, social media analysis, and market research.
13. Text Generation
Generating new, coherent text based on a given input, which can be used for creative writing, chatbots, and content creation.
14. Plagiarism Detection
Identifying and highlighting copied or similar content across documents by comparing text fragments.
15. Corpus Management
Working with large text corpora, including processing, searching, and analyzing linguistic data stored in corpora like Project Gutenberg, WordNet, etc.
16. Language Detection
Detecting the language of a given text, which is useful in multilingual applications.
These applications demonstrate the versatility of NLTK and its importance in various NLP tasks. Whether you're working on academic research, developing NLP-based applications, or analyzing social media data, NLTK provides a robust foundation for your work in Python.
Conclusion
The NLTK toolkit is a powerful library that simplifies the process of working with textual data in Python. Whether you are a beginner or an experienced data scientist, NLTK offers a broad range of tools to help you with your NLP projects. By mastering the basics covered in this guide, you’ll be well on your way to tackling more advanced NLP tasks.
Start exploring NLTK today and unlock the potential of text data in your projects!
Comments