Neural networks, inspired by the human brain, have revolutionized the field of machine learning. They excel at complex pattern recognition tasks, making them the backbone of many applications, from image recognition to natural language processing. Python, with its rich ecosystem of libraries, is the preferred language for building and training neural networks. In this blog, we'll delve into the basics of neural networks and explore their implementation using Python.
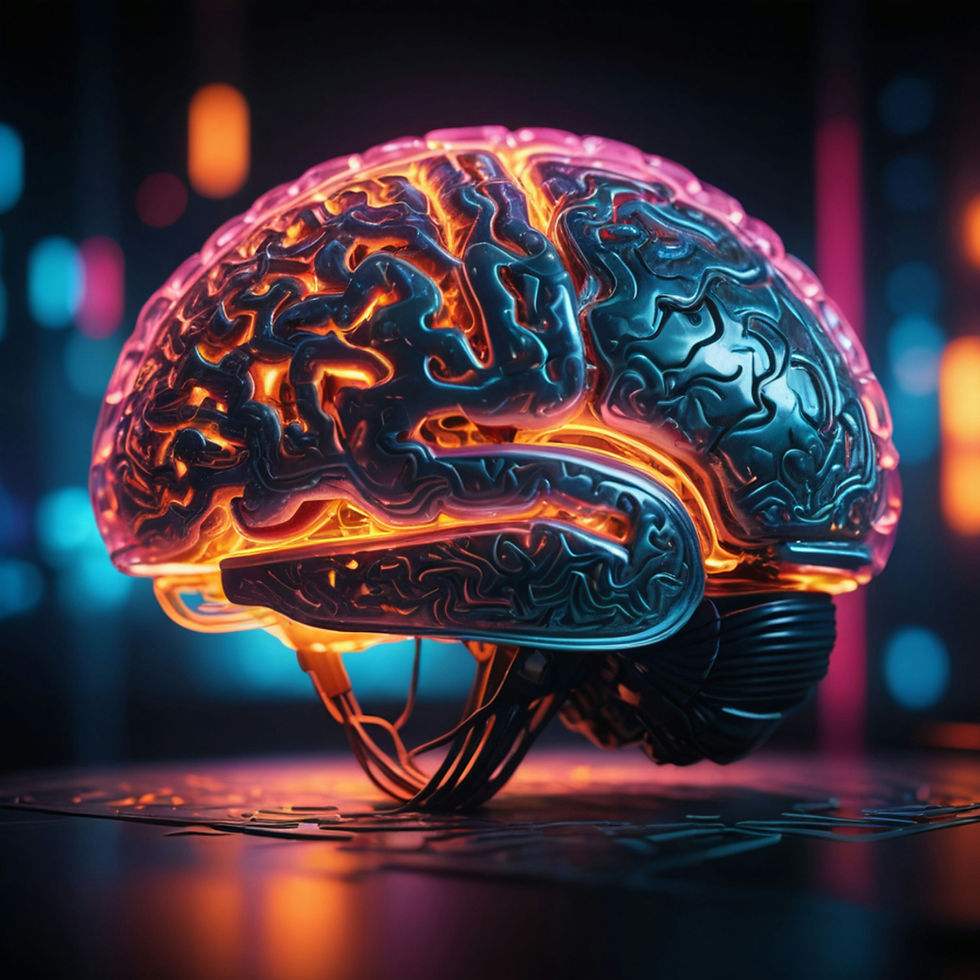
What is a Neural Network?
A neural network is a computational model inspired by the structure and function of the human brain. It consists of interconnected nodes, or artificial neurons, organized into layers. These neurons process information by receiving inputs, performing calculations, and passing the results to connected neurons. Neural networks learn from data through a process called training, where the network adjusts its internal parameters to minimize errors between its predictions and actual outcomes. This ability to learn from data enables neural networks to excel at tasks like image recognition, natural language processing, and complex pattern recognition, making them a cornerstone of modern artificial intelligence. Neural networks are the cornerstone of modern artificial intelligence, enabling machines to learn and make decisions in ways that mimic human cognition. Their ability to process complex patterns and extract meaningful information from vast datasets has revolutionized fields from image and speech recognition to natural language processing and medical diagnostics. By modeling intricate relationships between inputs and outputs, neural networks excel at tasks that were once considered the exclusive domain of humans. This has led to breakthroughs in areas like self-driving cars, fraud detection, and drug discovery, underscoring their immense potential to drive innovation and solve some of the world's most challenging problems.
Neural networks in Python
Neural networks in Python are computational models inspired by the human brain, designed to recognize patterns and make decisions. Python's rich ecosystem, including libraries like TensorFlow, Keras, and PyTorch, provides powerful tools to construct and train these networks. By interconnecting nodes (neurons) into layers, neural networks process input data, extract features, and produce outputs. Libraries like TensorFlow/Keras simplify this process by offering high-level APIs for defining network architectures, compiling models, and training them efficiently on large datasets. These networks excel at tasks like image recognition, natural language processing, and predictive modeling, making Python a preferred choice for data scientists and machine learning practitioners.
Some key concepts for getting started with Neural Networks:
These components work together to create a powerful and adaptable machine learning model.
Activation Functions: Most of these mathematical functions introduce non-linearity to the neural network, enabling it to learn complex patterns. Examples include ReLU (Rectified Linear Unit), sigmoid, and tanh. They determine whether a neuron should be activated based on the input.
Loss Function: This function quantifies the error between the network's predicted output and the actual target values. The goal is to minimize this error during training. Common loss functions are mean squared error (MSE) for regression problems and categorical cross-entropy for classification tasks.
Optimizer: An optimizer adjusts the network's parameters (weights and biases) to minimize the loss function. Popular optimizers include Gradient Descent, Adam, and RMSprop. They define how the model learns from its mistakes.
Back-propagation: This algorithm calculates the gradient of the loss function with respect to the network's parameters. It efficiently propagates the error backward through the network, allowing for weight updates. Backpropagation is the foundation of training neural networks.
Hyperparameter Tuning: Hyperparameters are settings that determine the network's architecture and training process. Examples include learning rate, number of layers, and batch size. Hyperparameter tuning involves finding the optimal values for these parameters to maximize the model's performance. Techniques like grid search and random search can be used for this purpose.
Building a Simple Neural Network in Python
We'll use the popular library TensorFlow and its high-level API Keras to build a simple neural network.
Step 1: Install Required Python Libraries
First, install TensorFlow. You can do this using pip:
pip install tensorflow
Step 2: Import Libraries
import numpy as np
import tensorflow as tf
from tensorflow import keras
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.datasets import load_iris
Step 3: Prepare the Dataset
For this example, we'll use the popular Iris dataset.
# Load the Iris dataset
data = load_iris()
X = data.data
y = data.target
# One-hot encode the target variable
y = keras.utils.to_categorical(y)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
Step 4: Build the Neural Network
# Define the model
model = keras.Sequential([
keras.layers.Dense(8, input_shape=(X_train.shape[1],), activation='relu'),
keras.layers.Dense(3, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
Step 5: Train the Model
# Train the model
history = model.fit(X_train, y_train, epochs=50, validation_split=0.2)
Output for the above code (last few iterations):
3/3 [==============================] - 0s 25ms/step - loss: 0.8970 - accuracy: 0.6667 - val_loss: 0.7931 - val_accuracy: 0.6667 Epoch 44/50 3/3 [==============================] - 0s 20ms/step - loss: 0.8876 - accuracy: 0.6667 - val_loss: 0.7854 - val_accuracy: 0.6667 Epoch 45/50 3/3 [==============================] - 0s 15ms/step - loss: 0.8784 - accuracy: 0.6667 - val_loss: 0.7775 - val_accuracy: 0.6667 Epoch 46/50 3/3 [==============================] - 0s 21ms/step - loss: 0.8694 - accuracy: 0.6771 - val_loss: 0.7698 - val_accuracy: 0.6667 Epoch 47/50 3/3 [==============================] - 0s 30ms/step - loss: 0.8604 - accuracy: 0.6771 - val_loss: 0.7622 - val_accuracy: 0.6667 Epoch 48/50 3/3 [==============================] - 0s 27ms/step - loss: 0.8514 - accuracy: 0.6771 - val_loss: 0.7547 - val_accuracy: 0.6667 Epoch 49/50 3/3 [==============================] - 0s 20ms/step - loss: 0.8427 - accuracy: 0.6771 - val_loss: 0.7475 - val_accuracy: 0.6667 Epoch 50/50 3/3 [==============================] - 0s 28ms/step - loss: 0.8340 - accuracy: 0.6771 - val_loss: 0.7405 - val_accuracy: 0.6667
Step 6: Evaluate the model
# Evaluate the model on the test set
test_loss, test_acc = model.evaluate(X_test, y_test)
print(f'Test accuracy: {test_acc}')
Output for the above code:
1/1 [==============================] - 0s 39ms/step - loss: 0.7431 - accuracy: 0.7333 Test accuracy: 0.7333333492279053
Step 7: Make Predictions
# Make predictions
predictions = model.predict(X_test)
print([i.argmax() for i in predictions])
Output for the above code:
1/1 [==============================] - 0s 43ms/step [2, 0, 2, 2, 2, 0, 1, 2, 2, 2, 2, 0, 0, 0, 0, 2, 2, 2, 2, 2, 0, 2, 0, 2, 2, 2, 2, 2, 0, 0]
In conclusion, neural networks are a powerful tool for solving complex problems. Python, with its rich ecosystem, makes it accessible to build and experiment with these models. While the basics are covered here, there's a vast world to explore in the realm of neural networks. Keep learning and experimenting to unlock their full potential!
You can experiment with different architectures, activation functions, and optimization algorithms to improve performance. If you have any specific questions or want to delve deeper into any part of neural networks, feel free to contact us!
Comments