In the world of machine learning, the perceptron is one of the most fundamental concepts. It forms the building block of more complex neural networks and helps us understand how machines learn from data. In this blog, we'll explore what a perceptron is, how it works, and how to implement it using TensorFlow.
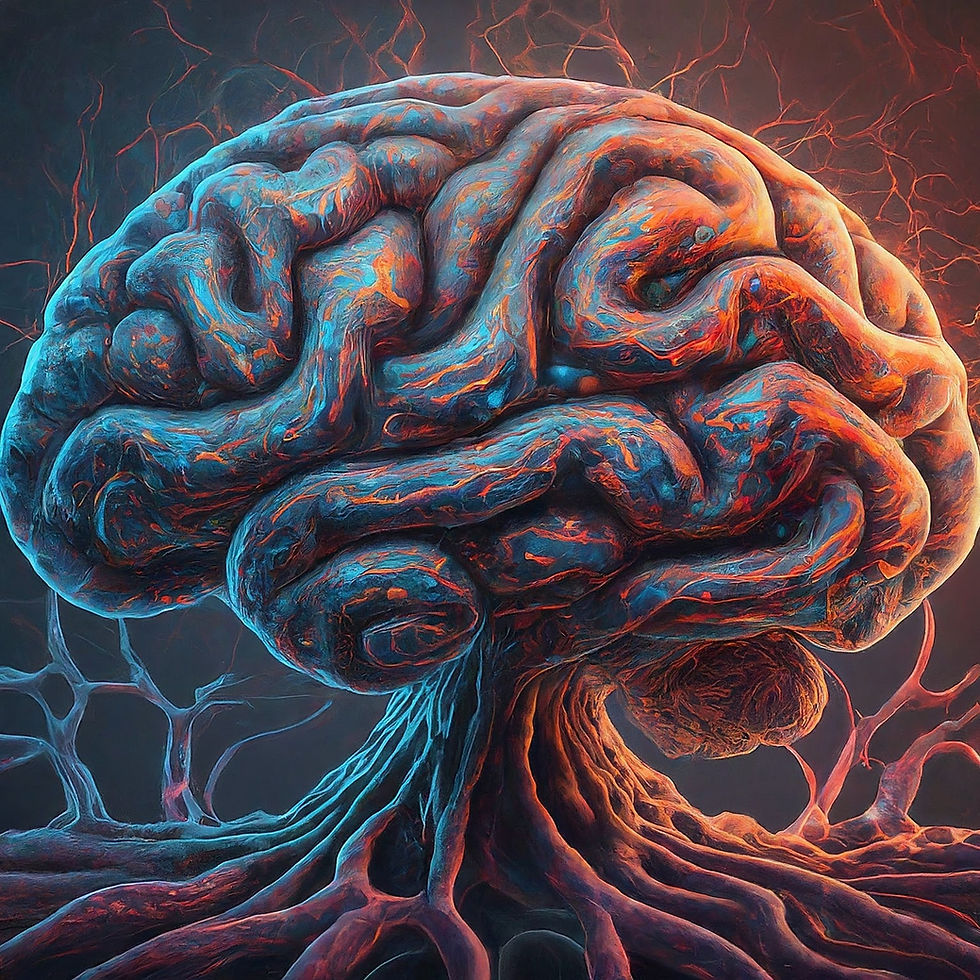
What is a Perceptron?
A perceptron is the most basic type of artificial neural network and serves as a fundamental building block for more complex neural models. It was introduced by Frank Rosenblatt in 1958 and is primarily used for binary classification tasks. The perceptron consists of a single neuron that processes input data through a simple mechanism: it multiplies each input by an associated weight, sums these weighted inputs, and then passes the result through an activation function, typically a step function. The activation function determines the output of the perceptron, which is either 0 or 1, based on whether the weighted sum exceeds a certain threshold. The perceptron can learn from data by adjusting its weights during the training process to minimize the difference between its predicted output and the actual target, thereby enabling it to solve simple linear classification problems.
How Does a Perceptron Work?
A perceptron operates by processing inputs through a series of simple steps. Initially, it takes multiple input values, each of which is multiplied by a corresponding weight. These weighted inputs are then summed together to produce a single value known as the weighted sum. This sum is then passed through an activation function, typically a step function or a sigmoid function, which determines the output of the perceptron. The output is binary, meaning it can be either 0 or 1, based on whether the weighted sum exceeds a certain threshold. During the training phase, the perceptron adjusts its weights based on the difference between the predicted output and the actual target, allowing it to improve its predictions over time. This process of adjusting weights continues iteratively, enabling the perceptron to learn and perform binary classification tasks effectively. A perceptron works through a series of steps:
Input: The perceptron receives multiple inputs, each of which is multiplied by a corresponding weight.
Weighted Sum: All the weighted inputs are summed to create a weighted sum.
Activation Function: The weighted sum is passed through an activation function, usually a step function, which determines the perceptron's output. If the weighted sum is above a certain threshold, the perceptron outputs one class (e.g., 1), and if it's below, it outputs another class (e.g., 0).
Output: The output is then compared to the expected output, and the weights are adjusted accordingly during the training phase.
Implementing a Perceptron using TensorFlow in Python
Implementing a Perceptron using TensorFlow in Python is a straightforward process that introduces you to the fundamentals of neural networks. The perceptron is a simple model used for binary classification, and it consists of an input layer, a single neuron (the perceptron), and an output layer. In TensorFlow, you can create this model using the Sequential API, where you define a dense layer with one unit (the neuron) and an appropriate activation function, such as the sigmoid. Once the model is built, it is compiled with an optimizer (like stochastic gradient descent) and a loss function (such as binary crossentropy). After compiling, the perceptron is trained on a dataset, adjusting its weights to minimize error and improve accuracy. This process involves feeding data through the model, calculating predictions, comparing them to actual outputs, and updating the model's weights accordingly. Implementing a perceptron in TensorFlow not only solidifies your understanding of basic neural networks but also lays the groundwork for building more complex models. TensorFlow is a powerful library for building and training neural networks. Let's walk through the process of implementing a perceptron using TensorFlow.
Step 1: Importing Libraries
First, we'll import the necessary libraries, including TensorFlow.
import tensorflow as tf
import numpy as np
Step 2: Defining the Data
We'll define a simple dataset for binary classification. Here, we'll use the XOR problem, where the output is 1 if the inputs are different and 0 if they are the same.
# Input data
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
# Labels
y = np.array([[0], [1], [1], [0]])
Step 3: Building the Perceptron Model
Next, we'll build the perceptron model. In TensorFlow, this can be done using the Sequential API.
# Creating the perceptron model
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=1, input_shape=(2,), activation='sigmoid')
])
# Compiling the model
model.compile(optimizer='sgd', loss='binary_crossentropy', metrics=['accuracy'])
Step 4: Training the Model
We'll now train the perceptron model using the XOR dataset.
# Training the model
model.fit(X, y, epochs=10)
Output for the above code:
Epoch 7/10
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 56ms/step - accuracy: 0.5000 - loss: 0.7635
Epoch 8/10
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 40ms/step - accuracy: 0.5000 - loss: 0.7634
Epoch 9/10
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 56ms/step - accuracy: 0.5000 - loss: 0.7633
Epoch 10/10
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 37ms/step - accuracy: 0.5000 - loss: 0.7632
Step 5: Evaluating the Model
After training, we can evaluate the model's performance.
# Evaluating the model
loss, accuracy = model.evaluate(X, y)
print(f'Accuracy: {accuracy}')
Step 6: Making Predictions
Finally, we can use the trained perceptron to make predictions on new data.
# Making predictions
predictions = model.predict(X)
print(predictions)
Output for the above code:
/1 ━━━━━━━━━━━━━━━━━━━━ 0s 41ms/step
[[0.5009955 ]
[0.22890633]
[0.7027152 ]
[0.41139105]]
Full Code for Perceptrons with TensorFlow in Python
Implementing a perceptron with TensorFlow in Python involves several key steps. First, import the necessary libraries, including TensorFlow and NumPy. Then, define the perceptron model architecture, typically using a single layer with linear activation or sigmoid. Next, compile the model with an appropriate optimizer (like Adam or SGD) and loss function (such as mean squared error). To train the model, feed it input data and corresponding labels, iteratively adjusting weights through backpropagation. Finally, evaluate the model's performance on a test dataset to assess its accuracy. While TensorFlow provides high-level abstractions for building neural networks, understanding the underlying principles of perceptrons is essential for effective model design and optimization.
import tensorflow as tf
import numpy as np
# Input data
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
# Labels
y = np.array([[0], [1], [1], [0]])
# Creating the perceptron model
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=1, input_shape=(2,), activation='sigmoid')
])
# Compiling the model
model.compile(optimizer='sgd', loss='binary_crossentropy', metrics=['accuracy'])
# Training the model
model.fit(X, y, epochs=10)
# Evaluating the model
loss, accuracy = model.evaluate(X, y)
print(f'Accuracy: {accuracy}')
# Making predictions
predictions = model.predict(X)
print(predictions)
Conclusion
The perceptron is a simple yet powerful concept in machine learning. It serves as the foundation for more complex neural networks and helps us understand the basic principles of how machines learn. By implementing a perceptron in TensorFlow, we gain practical experience in building and training neural networks, paving the way for more advanced projects.
Whether you're a beginner or an experienced developer, understanding the perceptron and how to implement it using TensorFlow is an essential step in your machine learning journey.
Commentaires