Prototypes in JavaScript: A Complete Guide
- Samul Black
- Jan 20
- 4 min read
Updated: Jan 31
JavaScript is a dynamic and flexible language, and one of its most powerful features is its prototype-based inheritance model. While many developers are familiar with classes and objects, understanding prototypes can significantly enhance your JavaScript skills. In this tutorial, we’ll dive deep into prototypes, explaining their purpose, how they work, and why they’re essential.
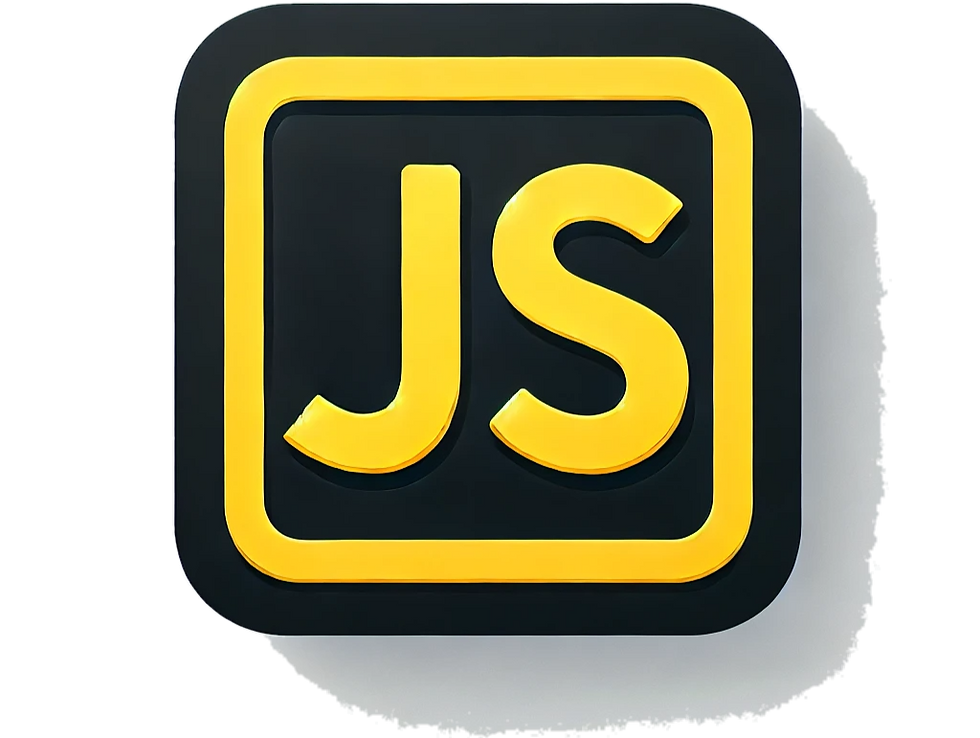
What Are Prototypes in JavaScript?
In JavaScript, every object is connected to another object called its prototype. This prototype acts as a blueprint, allowing the object to inherit properties and methods. These connections form what’s known as the prototype chain, a fundamental concept in JavaScript’s object-oriented design.
Prototypes are at the core of how inheritance works in JavaScript. Rather than duplicating properties and methods for each object, prototypes allow objects to share functionality seamlessly. This not only makes your code more efficient but also ensures that updates to a prototype automatically reflect across all objects that inherit from it. Imagine having a single source of truth for shared methods—prototypes make this possible.
Understanding prototypes can feel a bit abstract at first, but once you get the hang of it, the possibilities are endless. Whether you’re building reusable components, optimizing memory usage, or exploring modern features like ES6 classes (which are built on prototypes), this concept is essential for writing clean and effective JavaScript code.
Creating Objects and Prototypes
Let’s start with a simple example:
const person = {
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
const john = Object.create(person);
john.name = "John";
john.greet(); // Output: Hello, my name is John
In this example:
person acts as the prototype.
john is an object that inherits from person.
The greet method is available to john via the prototype chain.
The Prototype Chain
When you try to access a property or method on an object, JavaScript first checks the object itself. If the property is not found, it looks up the prototype chain. Here’s an example:
console.log(john.hasOwnProperty('name')); // Output: true
console.log(john.hasOwnProperty('greet')); // Output: false
console.log(Object.getPrototypeOf(john) === person); // Output: true
The prototype Property and Function Constructors
In JavaScript, functions have a special property called prototype. This property is used when creating objects with constructors:
function Animal(type) {
this.type = type;
}
Animal.prototype.speak = function() {
console.log(`I am a ${this.type}`);
};
const dog = new Animal('dog');
dog.speak(); // Output: I am a dog
Here:
Animal is a constructor function.
Animal.prototype contains methods shared by all instances created by new Animal().
Using ES6 Classes
Although ES6 introduced the class syntax, it’s built on top of the prototype system:
class Vehicle {
constructor(type) {
this.type = type;
}
describe() {
console.log(`This is a ${this.type}`);
}
}
const car = new Vehicle('car');
car.describe(); // Output: This is a car
console.log(Object.getPrototypeOf(car) === Vehicle.prototype); // Output: true
Classes provide a cleaner syntax while maintaining the prototype-based inheritance model.
Prototypes in Built-In Objects
Prototypes are used in built-in objects like Array, Object, and String. For example:
const arr = [1, 2, 3];
console.log(arr.__proto__ === Array.prototype); // Output: true
console.log(Array.prototype.__proto__ === Object.prototype); // Output: true
Here, arr inherits methods like map, filter, and reduce from Array.prototype, which in turn inherits from Object.prototype.
Modifying Prototypes
You can add or override properties and methods on an object’s prototype:
Array.prototype.sum = function() {
return this.reduce((total, num) => total + num, 0);
};
const numbers = [1, 2, 3, 4];
console.log(numbers.sum()); // Output: 10
Caution: Modifying built-in prototypes can lead to conflicts and unexpected behavior, so it’s best to avoid doing this in production code.
Checking and Setting Prototypes
You can inspect and manipulate prototypes with these methods:
Object.getPrototypeOf(obj): Returns the prototype of obj.
Object.setPrototypeOf(obj, proto): Sets the prototype of obj to proto.
Example:
const obj = {};
const proto = { greet: () => console.log('Hello!') };
Object.setPrototypeOf(obj, proto);
obj.greet(); // Output: Hello!
Benefits and Pitfalls of Prototypes in Javascript
Prototypes in JavaScript provide a powerful mechanism for sharing properties and methods across objects, enabling efficient memory usage and fostering code reuse. By utilizing prototypes, developers can define methods on the prototype object, ensuring that all instances of a constructor share the same method rather than duplicating it in memory. This approach leads to more maintainable and performant code. However, prototypes come with pitfalls that can challenge developers, especially those new to JavaScript. For instance, modifying native prototypes (e.g., Array.prototype or Object.prototype) can lead to unexpected behavior and conflicts in the codebase. Additionally, the prototype chain, while flexible, can introduce complexity, making debugging and understanding inheritance relationships more difficult. Developers must balance the benefits of prototypes with careful consideration of their potential impact on readability and maintainability.
Benefits of Prototypes
Memory Efficiency: Shared methods and properties reduce memory usage.
Dynamic Behavior: Changes to the prototype reflect in all derived objects.
Powerful Inheritance: Enables a flexible inheritance model without rigid class hierarchies.
Common Pitfalls
Prototype Pollution: Avoid inadvertently modifying the prototype of global objects.
Overuse of __proto__: While widely used, __proto__ is deprecated. Use Object.getPrototypeOf and Object.setPrototypeOf instead.
Understanding Scope: Properties on the prototype are shared across all instances, so changes affect all objects.
Conclusion
Prototypes are at the heart of JavaScript’s inheritance model, offering a powerful and efficient way to share functionality among objects. While they might seem complex at first, a solid grasp of prototypes will deepen your understanding of JavaScript and open doors to writing cleaner, more effective code. By leveraging prototypes effectively, you can create scalable applications that follow DRY (Don't Repeat Yourself) principles, reducing redundancy and improving maintainability.
Keep experimenting with prototypes and applying them in real-world scenarios to truly master their use. Explore their behavior in edge cases, such as how they handle property lookups or prototype chain modifications, to gain a nuanced understanding. Embracing prototypes can also enhance your ability to work with modern JavaScript features, such as ES6 classes, which are built on the prototype model. Ultimately, prototypes are not just a foundational concept—they are a tool that empowers developers to craft sophisticated and efficient solutions.
Kommentarer