Python, renowned for its readability and simplicity, has become a go-to language for beginners and seasoned programmers alike. Its versatility spans from web development and data science to automation and machine learning. Let's embark on a journey to understand the fundamentals of this powerful language.
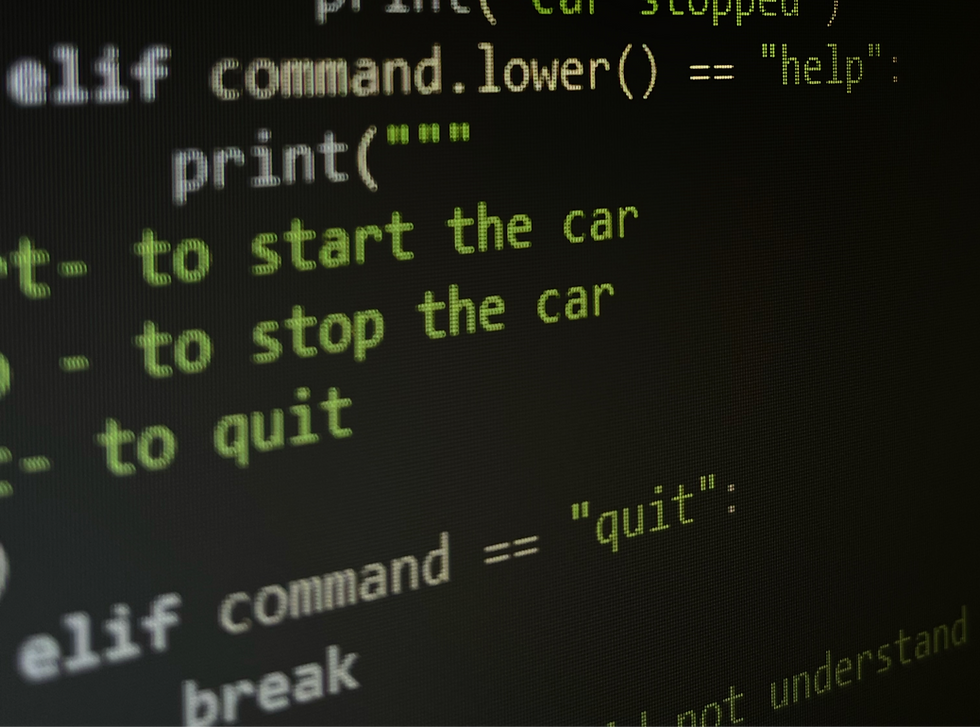
Introduction to Python Basics
Python is a high-level, interpreted programming language known for its readability and simplicity. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python's syntax is designed to be intuitive and mirrors natural language, making it accessible for beginners. Basic concepts in Python include variables, which are used to store data, and data types such as integers, floats, strings, and lists. Python also provides control structures like loops (for and while) and conditional statements (if, elif, else) to control the flow of programs. Functions allow for code reuse and modularity, while libraries and modules extend Python's capabilities to various domains like data analysis, web development, and artificial intelligence. Python's extensive standard library and active community make it a versatile and powerful tool for developers.
Setting Up Your Environment
Before diving into code, ensure you have Python installed. You can download the latest version from https://www.python.org/. For a more streamlined development experience, consider using a code editor or IDE like Visual Studio Code, PyCharm, or Jupyter Notebook.
Hello, World!
Every programming journey begins with a classic:
print("Hello, World!")
This simple line introduces the print() function, which displays the enclosed text on the console.
Python Variables and Data Types
Variables are containers for storing data. Python is dynamically typed, meaning you don't need to specify the data type beforehand.
name = "Alice" # String
age = 30 # Integer
height = 1.65 # Float
is_student = True # Boolean
Python supports various data types:
Numbers: Integers (whole numbers), floats (decimal numbers), complex numbers
Strings: Textual data enclosed in quotes (single or double)
Booleans: True or False values
Lists: Ordered collections of items
Tuples: Immutable ordered collections
Dictionaries: Unordered collections of key-value pairs
Operators in Python
Operators are special symbols or keywords used to perform operations on values and variables in Python. They form the backbone of expressions, which are combinations of operators and operands (values or variables) that produce a result.
Python offers a variety of operators to handle different operations:
Arithmetic Operators
These are used to perform basic mathematical calculations:
+: Addition
-: Subtraction
*: Multiplication
/: Division
%: Modulus (remainder)
//: Floor division (integer division)
**: Exponentiation
Comparison Operators
These operators compare two values and return a Boolean result (True or False):
==: Equal to
!=: Not equal to
<: Less than
>: Greater than
<=: Less than or equal to
>=: Greater than or equal to
Assignment Operators
These operators assign values to variables:
=: Simple assignment
+=: Add and assign
-=: Subtract and assign
*=: Multiply and assign
/=: Divide and assign
%=: Modulus and assign
//=: Floor division and assign
**=: Exponentiation and assign
Logical Operators
These operators are used to combine conditional statements:
and: Logical AND
or: Logical OR
not: Logical NOT
Bitwise Operators
These operators perform bit-by-bit operations on integer values:
&: Bitwise AND
|: Bitwise OR
^: Bitwise XOR
~: Bitwise NOT
<<: Left shift
>>: Right shift
Membership Operators
These operators test for membership in a sequence:
in: Checks if a value is present in a sequence
not in: Checks if a value is not present in a sequence
Identity Operators
These operators compare the identity of two objects:
is: Checks if two variables refer to the same object
is not: Checks if two variables refer to different objects
Control Flow in Python
Control flow statements determine the execution order of your code.
Conditional statements:
if condition:
# code to execute if condition is True
else:
# code to execute if condition is False
for loop: Iterates over a sequence
for item in sequence:
# code to execute for each item
while loop: Repeats as long as a condition is True
while condition:
# code to execute while condition is True
Functions in Python
Functions are reusable blocks of code that perform specific tasks.
def greet(name):
print("Hello,", name + "!")
greet("Bob")
Input and Output Operations in Python
Python allows you to interact with users through input and output operations.
name = input("Enter your name: ")
print("Hello,", name)
Libraries and Modules in Python
Python’s extensive standard library and third-party modules extend its capabilities. For instance, the math module provides mathematical functions, while the pandas library is essential for data analysis.
import math
print(math.sqrt(16)) # Outputs: 4.0
import pandas as pd
data = pd.read_csv("data.csv")
print(data.head())
Lists and Dictionaries
Lists and dictionaries are data structures that allow you to store collections of items.
Lists:
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Outputs: apple
Dictionaries:
person = {"name": "Alice", "age": 30}
print(person["name"]) # Outputs: Alice
In conclusion, mastering the basics of Python is a critical first step on the path to becoming a proficient programmer. Python’s simple and readable syntax allows new learners to focus on understanding fundamental programming concepts without getting bogged down by complex syntax rules. The language's dynamic typing and versatility in supporting different programming paradigms empower developers to write clean, efficient, and reusable code. Through variables, data types, control structures, functions, and data structures like lists and dictionaries, Python provides a robust toolkit for solving a wide range of problems. Additionally, Python’s extensive standard library and active global community mean that solutions and support are readily available, making it easier to overcome challenges and extend your capabilities. As you continue to explore Python, you'll find that these basic building blocks open the door to more advanced areas such as data analysis, machine learning, web development, and automation, making Python a truly indispensable tool in the modern programmer’s arsenal. Whether you aim to build a simple script or a complex application, the foundational knowledge of Python will serve as a strong and enduring base for your programming endeavors.
This is just a glimpse into the vast world of Python. As you continue your learning journey, you'll discover more advanced concepts like object-oriented programming, modules, and libraries. Python's simplicity and readability make it an excellent choice for beginners and professionals alike. By mastering the basics, you lay a solid foundation for more advanced topics like web development, data science, and machine learning. Happy coding!
Remember, practice is key to mastering any programming language. Start with small projects, gradually increasing complexity, and explore the endless possibilities that Python offers.
Commentaires