Python's simplicity and readability make it a top choice for beginners and experts alike. At the core of every Python program are its basic building blocks: primitives, variables, and expressions. Understanding these concepts is key to writing efficient code, whether for simple scripts or complex applications. In this blog, we'll explore these essentials, explaining what they are, how they work, and why they matter.
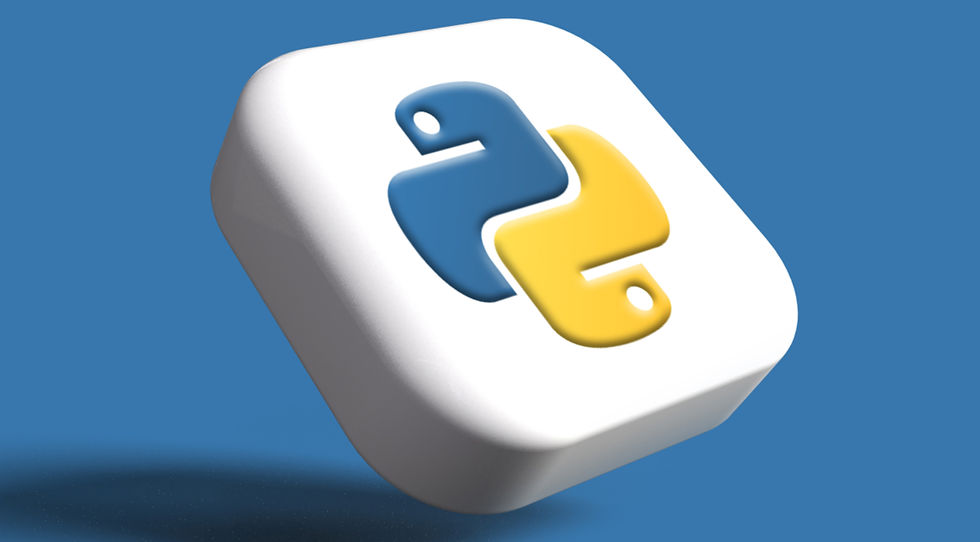
Importance of Python Primitives, Variables, and Expressions
Python primitives, variables, and expressions are the fundamental components of programming in Python, forming the foundation upon which all programs are built. Primitives represent the simplest types of data, like numbers and strings, allowing us to work with information directly. Variables act as containers for storing and manipulating these data types, making our code more flexible and readable. Expressions combine variables and primitives using operators to perform calculations and logical operations, enabling complex decision-making and data processing. A solid grasp of these concepts is crucial for any programmer, as they underpin every aspect of Python coding, from simple scripts to advanced data analysis and machine learning algorithms. By mastering these basics, one can build more complex programs efficiently and lay a strong groundwork for further exploration in computer science and software development.
Python is known for its simplicity and ease of use, especially for beginners. This simplicity extends to its fundamental building blocks: primitives, variables, and expressions. Let’s break down each of these essential elements to help you get started with Python programming.
1. Python Primitives: The Building Blocks
Primitives are the most basic data types that Python supports. They are used to represent simple values and are the foundation of all other data types and structures. Python has four primary primitive data types:
1.1 Integer (int)
An integer is a whole number, either positive or negative, without any decimal or fractional part. In Python, integers can be of any length, constrained only by the memory available.
num1 = 42
num2 = -100
1.2 Float (float)
A float represents a real number that includes a decimal point. Floats are used for values that need more precision, like measurements or monetary values.
pi = 3.14159
temperature = -10.5
1.3 Boolean (bool)
A boolean has only two possible values: True or False. Booleans are primarily used in conditional statements to control the flow of a program.
is_sunny = True
has_coffee = False
1.4 String (str)
A string is a sequence of characters enclosed within single quotes ('...') or double quotes ("..."). Strings can contain letters, numbers, symbols, and even spaces.
name = "Alice"
message = 'Hello, World!'
2. Variables: Storing Data
Variables are containers for storing data values. In Python, you don't need to declare the type of a variable; it is dynamically inferred based on the assigned value. Here’s how you define a variable:
age = 25
height = 5.9
is_student = True
greeting = "Good morning!"
Naming Variables
Variable names can contain letters, numbers, and underscores (_).
They must start with a letter or an underscore.
Variable names are case-sensitive (age and Age are different).
Best Practices for Naming Variables
Use descriptive names: user_name is better than x.
Use lowercase words separated by underscores for readability: total_cost.
Avoid using Python keywords (like if, else, for, etc.) as variable names.
3. Expressions: Combining Values
An expression is a combination of variables, operators, and values that produce another value. Python supports a wide variety of expressions using arithmetic, comparison, and logical operators.
3.1 Arithmetic Expressions
You can use arithmetic operators like +, -, , /, //, %, and * to perform calculations.
a = 10
b = 3
# Basic arithmetic operations
sum_value = a + b # 13
difference = a - b # 7
product = a * b # 30
quotient = a / b # 3.333...
integer_division = a // b # 3
remainder = a % b # 1
power = a ** b # 1000
3.2 Comparison Expressions
These expressions compare values using operators like ==, !=, <, >, <=, and >=. They return a boolean (True or False).
x = 5
y = 10
# Comparison operations
is_equal = (x == y) # False
is_not_equal = (x != y) # True
is_greater = (x > y) # False
is_less_or_equal = (x <= y) # True
3.3 Logical Expressions
Logical operators like and, or, and not are used to combine multiple conditions.
has_money = True
is_hungry = False
# Logical operations
should_eat = has_money and is_hungry # False
can_go_out = has_money or is_hungry # True
not_hungry = not is_hungry # True
4. Constants
In Python, there isn't a built-in constant type, but you can create constants by using variable names in all uppercase to indicate that they shouldn't be modified.
PI = 3.14159
GRAVITY = 9.8
By convention, constants are named using uppercase letters with underscores separating words.
5. Putting It All Together: A Simple Example
Let's create a small script that calculates the area of a circle using Python primitives, variables, and expressions:
# Constants
PI = 3.14159
# Variables
radius = 5.0
# Expression: Calculate the area of the circle
area = PI * (radius ** 2)
# Output the result
print("The area of the circle with radius", radius, "is", area)
Output:
The area of the circle with radius 5.0 is 78.53975
Conclusion
Grasping the different data types—such as integers, floats, strings, and booleans—allows you to manipulate and store information effectively. Variables serve as symbolic names for these data types, making your code more readable and maintainable. Expressions enable you to perform operations on these variables, facilitating dynamic and interactive programming.
As you progress, you'll encounter control structures like loops and conditionals, which rely heavily on your understanding of these fundamental elements. Mastering them paves the way for working with data structures such as lists, dictionaries, and sets, further enhancing your ability to manage and organize information.
Moreover, once you're familiar with these basics, you can explore Python's libraries and frameworks, unlocking the potential to develop web applications, data analysis tools, and machine learning models. Each new concept you learn builds upon this foundational knowledge, empowering you to tackle more ambitious projects and deepen your understanding of computer science principles.
In essence, a solid grasp of primitives, variables, and expressions not only equips you with the skills necessary to write functional code but also fosters a mindset of logical thinking and problem-solving—essential traits for any aspiring programmer. Embrace these basics, and you’ll find yourself well-prepared to take on the exciting challenges that lie ahead in your coding journey.
Comments