Python is renowned for its simplicity and versatility. However, beneath its straightforward syntax lies a treasure trove of lesser-known tricks that can make your code more efficient and expressive. Whether you're a seasoned developer or just getting started, here are a few Python tricks that can elevate your coding game.
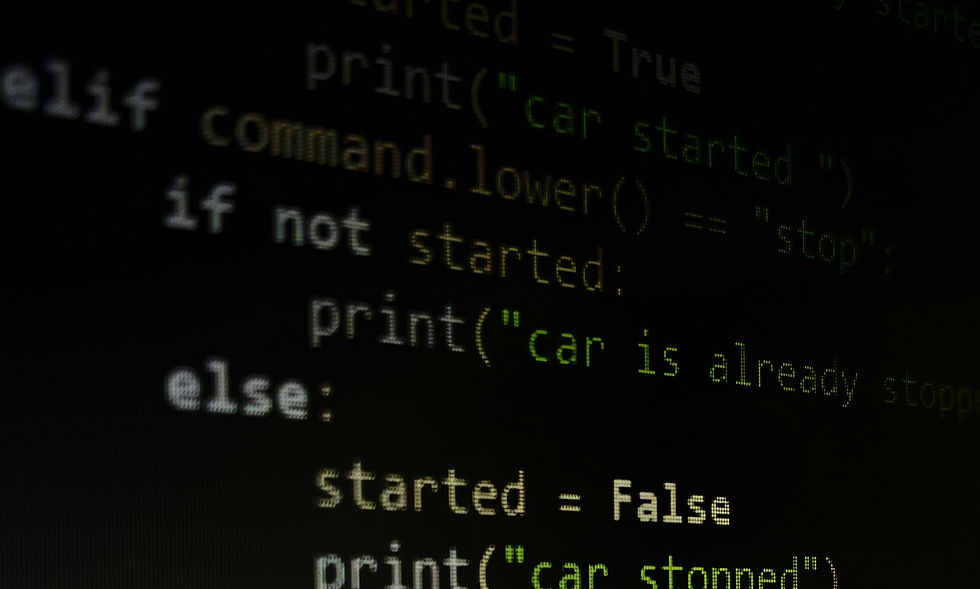
Why Learn Python?
Python is one of the most popular and versatile programming languages, making it an excellent choice for beginners and experienced developers alike. Its simplicity and readability enable rapid learning and easy debugging, while its extensive libraries and frameworks support a wide range of applications, from web development and automation to data analysis, artificial intelligence, and machine learning. Python’s active and supportive community ensures ample resources, tutorials, and problem-solving forums, making it ideal for self-paced learning. Whether you’re aiming to build a career in tech, automate tasks, or dive into cutting-edge fields like AI, learning Python is a step toward limitless opportunities.
Importance of Learning these Basic Tricks
Python is a versatile and intuitive programming language, but its true power lies in the clever tricks that can make your code cleaner, faster, and more efficient. From swapping variables in a single line to flattening nested lists with concise list comprehensions, Python offers elegant solutions for common tasks. You can merge dictionaries effortlessly with the | operator, count occurrences using collections.Counter, and simplify your conditionals with the walrus operator (:=). Chained comparisons and the zip function further enhance readability and functionality. Whether you're optimizing data manipulation or refining your logic, mastering these Python tricks can significantly elevate your development skills. These pythonic tricks are listed below:
1. Swap Variables Without a Temporary Variable
Instead of using a temporary variable to swap two values, Python allows you to do this in one line:
x, y = 5, 10
x, y = y, x
print(x, y) # Output: 10, 5
2. Use zip to Pair and Unpair Data
The zip function can be used to combine two lists into pairs or unzip them back.
# Pairing
names = ["Alice", "Bob", "Charlie"]
scores = [85, 92, 78]
paired = list(zip(names, scores))
print(paired) # Output: [('Alice', 85), ('Bob', 92), ('Charlie', 78)]
# Unpairing
unzipped = list(zip(*paired))
print(unzipped) # Output: (['Alice', 'Bob', 'Charlie'], [85, 92, 78])
3. One-Liner for List Comprehensions with Conditionals
You can filter items while creating a list using a conditional list comprehension.
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [n for n in numbers if n % 2 == 0]
print(even_numbers) # Output: [2, 4, 6]
4. Merge Dictionaries (Python 3.9+)
Python 3.9 introduced the | operator to merge dictionaries.
dict1 = {"a": 1, "b": 2}
dict2 = {"b": 3, "c": 4}
merged = dict1 | dict2
print(merged) # Output: {'a': 1, 'b': 3, 'c': 4}
5. Count Occurrences with collections.Counter
The Counter class from the collections module simplifies counting elements in an iterable.
from collections import Counter
data = ["apple", "banana", "apple", "orange", "banana", "apple"]
count = Counter(data)
print(count) # Output: Counter({'apple': 3, 'banana': 2, 'orange': 1})
6. Use _ as a Throwaway Variable
In Python, _ is often used to indicate a variable whose value you don’t need.
for _ in range(5):
print("Hello!") # Prints "Hello!" 5 times
7. The Walrus Operator (:=)
Introduced in Python 3.8, the walrus operator assigns and returns a value in a single expression.
numbers = [1, 2, 3, 4]
if (n := len(numbers)) > 3:
print(f"List is too long ({n} items).")
8. Get the Most Frequent Item in a List
Combining max with Counter makes finding the most frequent element straightforward.
from collections import Counter
data = [1, 2, 2, 3, 3, 3, 4]
most_frequent = max(Counter(data).items(), key=lambda x: x[1])[0]
print(most_frequent) # Output: 3
9. Simplify Chained Comparisons
Python allows chaining comparisons for cleaner code.
x = 10
if 5 < x < 15:
print("x is between 5 and 15.") # Output: x is between 5 and 15.
10. Easily Flatten a Nested List
Using a list comprehension, you can flatten a nested list.
nested = [[1, 2], [3, 4], [5, 6]]
flattened = [item for sublist in nested for item in sublist]
print(flattened) # Output: [1, 2, 3, 4, 5, 6]
Conclusion
Python’s elegance is in its expressiveness and the numerous ways to accomplish tasks efficiently. These tricks are just the tip of the iceberg. Mastering them can help you write cleaner, faster, and more Pythonic code. Explore, experiment, and let Python do the heavy lifting!
Comments