Working with files is a fundamental skill in programming, allowing you to store and retrieve data. Python provides straightforward methods to read from and write to files, making it easy to handle file operations. In this blog, we'll explore the basics of file reading and writing in Python, including how to open files, read content, write data, and handle file exceptions.
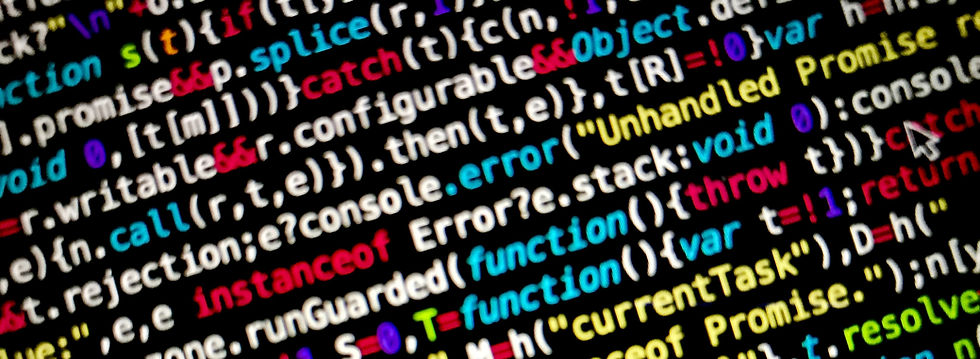
Reading and Writing Text Files in Python
Reading and writing text files in Python is a fundamental skill that facilitates the handling of data in a variety of applications. Python's built-in open() function, along with context managers (with statement), simplifies file operations by ensuring that files are properly opened and closed. Reading a file can be done in multiple ways, such as reading the entire content at once using read(), reading line by line using a loop, or reading into a list with readlines(). Writing to a file is equally straightforward, with modes like 'w' for writing (which overwrites existing content) and 'a' for appending new data to the end of the file. By using these methods, developers can efficiently manage text data, whether it's for logging, configuration, or data storage purposes.
Opening a Text File in Python
Before you can read from or write to a file, you need to open it. Python's built-in open() function is used to open a file, and it requires two arguments: the file path and the mode in which to open the file. Common file modes include:
'r' : Read (default mode)
'w': Write (truncates the file if it exists)
'a' : Append (adds content to the end of the file)
'b' : Binary mode (used with other modes, e.g., 'rb' or 'wb')
file = open('example.txt', 'r') # Open file for reading
Reading a Text File in Python
Python provides multiple methods to read from a file. Here are a few common ones:
Reading the entire file:
Using with open ensures the file is properly closed after reading.
with open('example.txt', 'r') as file:
print(content)
Output for the above code
Hello, World! This is a sample text file.
Reading line by line:
The strip() method removes any trailing newline characters.
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
Reading into a list:
This method reads all lines into a list, where each line is an element.
with open('example.txt', 'r') as file:
lines = file.readlines()
print(lines)
Output for the above code
['Hello, World!\n', 'This is a sample text file.']
Writing a Text File in Python
Writing to a file is equally straightforward. You can use the write() method to add content to a file.
Writing text:
with open('example.txt', 'w') as file:
file.write("Hello, World!\n This is a new file.")
Appending text:
This mode overwrites the existing content. To add new content without erasing the existing data, use the append mode ('a').
with open('example.txt', 'a') as file:
file.write("\nThis line is appended.")
Handling Exceptions with Text File in Python
When working with files, it's essential to handle potential exceptions, such as file not found errors. You can use try-except blocks to manage these scenarios.
try:
with open('example.txt', 'r') as file:
print(content)
except FileNotFoundError:
print("The file was not found.")
Output for the above code
Hello, World!
This is a new file.
This line is appended.
In Conclusion, file reading and writing are essential skills in Python programming. Whether you're processing text files, managing logs, or handling binary data, Python's built-in functions and context managers make these tasks simple and efficient. Remember to handle exceptions gracefully to ensure your programs can deal with unexpected issues, such as missing files or permission errors.
By mastering file operations in Python, you'll be well-equipped to handle a wide range of data processing tasks, making your programs more versatile and powerful.
Comments