Recurrent Neural Networks (RNNs) are a powerful class of neural networks designed for sequential data, making them ideal for tasks where the order of inputs matters, such as time series prediction, natural language processing, and speech recognition. Unlike traditional feedforward neural networks, RNNs have connections that form cycles within the network, allowing information to persist and be passed through from one step to the next. In this blog, we'll explore how to implement an RNN using TensorFlow in Python. We'll cover the basics of RNNs, their architecture, and how to apply them to a simple sequence prediction task.
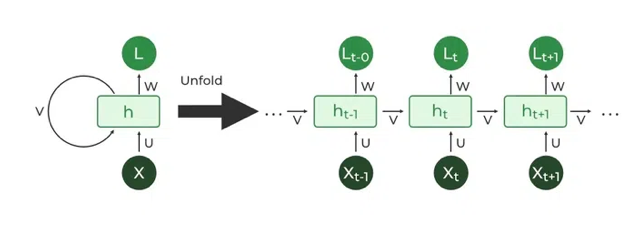
What are Recurrent Neural Networks (RNN's)?
A Recurrent Neural Network (RNN) is a type of artificial neural network specifically designed to handle sequential data, where the order of the data points is crucial. Unlike traditional feedforward neural networks, which assume all inputs are independent of each other, RNNs have a unique architecture that incorporates loops, allowing information to persist across different time steps. This looping mechanism enables the network to maintain a hidden state that captures the essence of the input sequence as it progresses, effectively creating a memory of previous inputs. This memory is critical for tasks like time series prediction, natural language processing, and speech recognition, where the context provided by prior inputs significantly influences the current output. However, RNNs face challenges, particularly with long sequences, due to issues like vanishing and exploding gradients, which can make training difficult. To address these challenges, variants of RNNs, such as Long Short-Term Memory (LSTM) networks and Gated Recurrent Units (GRUs), have been developed, which include mechanisms to better manage the flow of information over extended sequences.
Recurrent Neural Networks (RNN's) Variants
RNNs have evolved to address some of their inherent limitations, particularly with long sequences, leading to the development of several variants that enhance their capabilities. The most common RNN variants are:
Vanilla RNN: This is the basic form of an RNN, where each neuron in the hidden layer has a connection to itself, allowing it to retain information over time. However, Vanilla RNNs often struggle with learning long-term dependencies due to issues like vanishing and exploding gradients, making them less effective for long sequences.
Long Short-Term Memory (LSTM): LSTMs are designed to overcome the limitations of Vanilla RNNs by introducing a more complex architecture that includes three types of gates: input, output, and forget gates. These gates regulate the flow of information into and out of the cell state, effectively allowing the network to retain or forget information over longer periods. LSTMs are highly effective in tasks that require learning long-term dependencies, such as language translation, speech recognition, and time series forecasting.
Gated Recurrent Unit (GRU): GRUs are a simplified version of LSTMs that combine the input and forget gates into a single update gate, and merge the cell state and hidden state. This streamlined architecture reduces the number of parameters and computational complexity while still addressing the vanishing gradient problem. GRUs are often preferred in scenarios where computational efficiency is crucial, and they have shown comparable performance to LSTMs in many tasks.
Each of these RNN variants has its strengths and is chosen based on the specific requirements of the task at hand, whether it involves short or long sequences, computational constraints, or the complexity of the data being processed.
Implementing Recurrent Neural Networks (RNN's) with TensorFlow in Python
Let's walk through the implementation of an RNN using TensorFlow in Python. We'll use a simple sequence prediction task where the model learns to predict the next number in a sequence.
Step 1: Importing Necessary Libraries
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import SimpleRNN, Dense
import numpy as np
Step 2: Preparing the Data
For this example, we'll create a simple dataset where the model will learn to predict the next number in a sequence.
# Generate a simple dataset
def create_dataset(sequence, n_steps):
X, y = [], []
for i in range(len(sequence)):
end_ix = i + n_steps
if end_ix > len(sequence) - 1:
break
seq_x, seq_y = sequence[i:end_ix], sequence[end_ix]
X.append(seq_x)
y.append(seq_y)
return np.array(X), np.array(y)
# Example sequence
sequence = np.array([i for i in range(10)])
# Prepare the input-output pairs
n_steps = 3
X, y = create_dataset(sequence, n_steps)
# Reshape input to be [samples, time steps, features]
X = X.reshape((X.shape[0], X.shape[1], 1))
Step 3: Building the RNN Model
We'll use TensorFlow's Keras API to build the model. Here, we'll create a simple RNN with one hidden layer.
# Define the RNN model
model = Sequential()
model.add(SimpleRNN(50, activation='relu', input_shape=(n_steps, 1)))
model.add(Dense(1))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Summarize the model
model.summary()
Output for the above code:
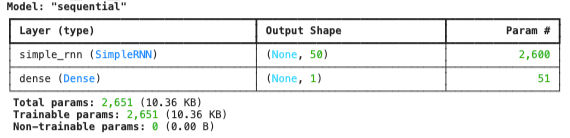
Step 4: Training Recurrent Neural Network Model
Next, we'll train the model on our dataset.
# Train the model
model.fit(X, y, epochs=200, verbose=1)
Output for the above code:
Epoch 184/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 57ms/step - loss: 0.3377
Epoch 185/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 29ms/step - loss: 0.3356
Epoch 186/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 61ms/step - loss: 0.3335
Epoch 187/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 31ms/step - loss: 0.3314
Epoch 188/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 56ms/step - loss: 0.3293
Epoch 189/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 32ms/step - loss: 0.3272
Epoch 190/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 32ms/step - loss: 0.3251
Epoch 191/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 34ms/step - loss: 0.3230
Epoch 192/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 37ms/step - loss: 0.3209
Epoch 193/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 38ms/step - loss: 0.3188
Epoch 194/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 52ms/step - loss: 0.3167
Epoch 195/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 35ms/step - loss: 0.3146
Epoch 196/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 57ms/step - loss: 0.3125
Epoch 197/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 55ms/step - loss: 0.3105
Epoch 198/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 57ms/step - loss: 0.3084
Epoch 199/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 31ms/step - loss: 0.3063
Epoch 200/200
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 57ms/step - loss: 0.3043
Step 5: Making Predictions
After training, the model can predict the next number in the sequence.
# Demonstrate prediction
x_input = np.array([7, 8, 9])
x_input = x_input.reshape((1, n_steps, 1))
yhat = model.predict(x_input, verbose=0)
print(f"Predicted next value: {yhat[0][0]}")
Output for the above code:
Predicted next value: 10.705029487609863
Full Code for Recurrent Neural Networks (RNNs) with TensorFlow in Python
Recurrent Neural Networks (RNNs) in TensorFlow allow you to model sequential data by maintaining a memory of past inputs, ideal for tasks like time series forecasting and language processing. TensorFlow's flexible API makes implementing RNNs, LSTMs, and GRUs straightforward, enabling efficient handling of both short and long sequences. Whether you're predicting the next word in a sentence or the next value in a time series, RNNs with TensorFlow provide the tools you need.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import SimpleRNN, Dense
import numpy as np
# Generate a simple dataset
def create_dataset(sequence, n_steps):
X, y = [], []
for i in range(len(sequence)):
end_ix = i + n_steps
if end_ix > len(sequence) - 1:
break
seq_x, seq_y = sequence[i:end_ix], sequence[end_ix]
X.append(seq_x)
y.append(seq_y)
return np.array(X), np.array(y)
# Example sequence
sequence = np.array([i for i in range(10)])
# Prepare the input-output pairs
n_steps = 3
X, y = create_dataset(sequence, n_steps)
# Reshape input to be [samples, time steps, features]
X = X.reshape((X.shape[0], X.shape[1], 1))
# Define the RNN model
model = Sequential()
model.add(SimpleRNN(50, activation='relu', input_shape=(n_steps, 1)))
model.add(Dense(1))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Summarize the model
model.summary()
# Train the model
model.fit(X, y, epochs=200, verbose=1)
# Demonstrate prediction
x_input = np.array([7, 8, 9])
x_input = x_input.reshape((1, n_steps, 1))
yhat = model.predict(x_input, verbose=0)
print(f"Predicted next value: {yhat[0][0]}")
Conclusion
In conclusion, Recurrent Neural Networks (RNNs) are a fundamental tool in the deep learning toolkit, especially suited for tasks involving sequential data. Their unique architecture allows them to maintain a memory of past inputs, making them invaluable for applications like time series prediction, language modeling, and speech recognition. However, the challenges associated with training RNNs, particularly with long sequences, led to the development of more advanced variants like LSTMs and GRUs. These variants enhance the network's ability to learn long-term dependencies by introducing mechanisms to better manage the flow of information. TensorFlow, with its user-friendly API, provides a powerful platform to implement and experiment with these networks, enabling researchers and developers to push the boundaries of what RNNs can achieve. Whether you're tackling a simple sequence prediction problem or a more complex task like machine translation, understanding and utilizing RNNs and their variants can significantly enhance the performance of your models. As you continue to explore and implement these networks, the possibilities for innovation are vast, making RNNs an exciting area of study and application in the field of deep learning.
Whether you're working with time series data, text, or any other type of sequence, RNNs provide a robust framework for learning patterns over time. With TensorFlow, you can experiment with different architectures, such as LSTMs or GRUs, and tackle more complex tasks like language translation or sentiment analysis.
Comentários