Sentiment Analysis with Python: Unlocking Insights from Text Data
- Samuel Black
- Aug 21, 2024
- 6 min read
In today's digital age, the volume of text data generated daily is staggering, from social media posts and product reviews to customer feedback and news articles. This vast amount of textual information holds valuable insights into public opinion, customer satisfaction, and brand perception. But how do we effectively extract and analyze these insights? This is where sentiment analysis comes into play, and Python provides the tools to do it efficiently.
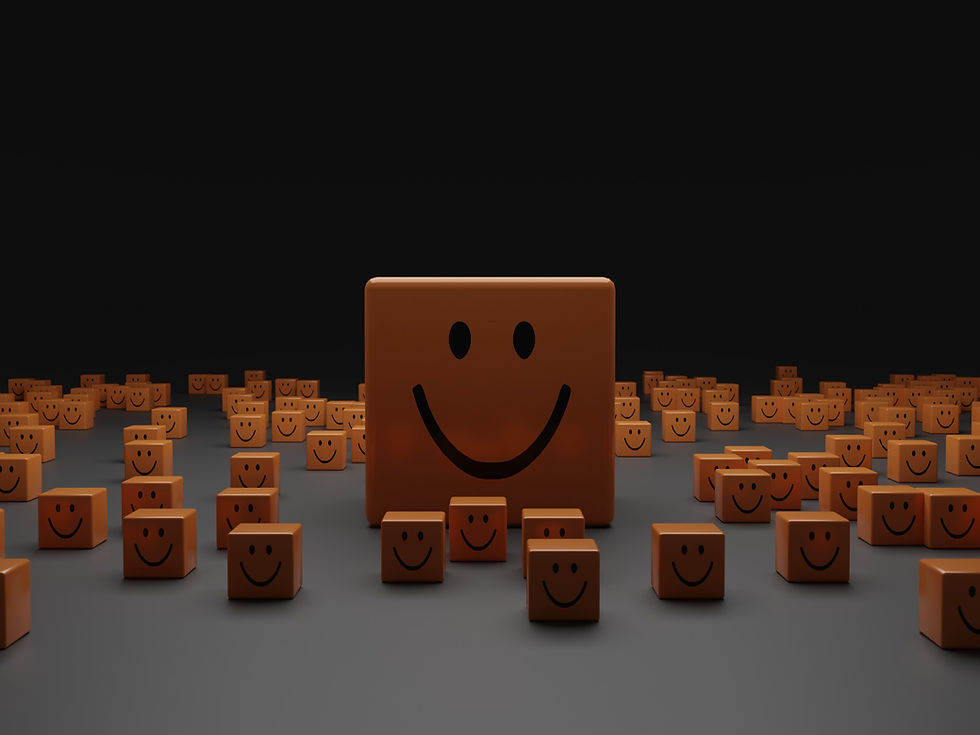
What is Sentiment Analysis?
Sentiment analysis, also known as opinion mining, is a natural language processing (NLP) technique used to determine the emotional tone behind a piece of text. It involves classifying text into categories such as positive, negative, or neutral, allowing organizations to understand the sentiment expressed in customer reviews, social media posts, emails, and other forms of textual communication. By analyzing sentiments, businesses can gauge public opinion, track brand perception, and respond to customer feedback more effectively. Sentiment analysis is widely used in various industries for market research, customer service, and social media monitoring, providing valuable insights that drive decision-making and strategy development.
Why Python for Sentiment Analysis?
Python is one of the most popular programming languages for sentiment analysis, thanks to its simplicity, extensive libraries, and strong community support. Libraries like NLTK (Natural Language Toolkit), TextBlob, and VADER (Valence Aware Dictionary for Sentiment Reasoning) offer pre-built functionalities to process and analyze text data efficiently. Moreover, Python's data manipulation libraries like Pandas and NumPy further simplify the handling of large datasets, making it an ideal choice for sentiment analysis.
Applications of Sentiment Analysis
Business Intelligence: Companies use sentiment analysis to monitor customer feedback and reviews, helping them improve products and services. Understanding customer sentiment allows businesses to respond proactively to potential issues and capitalize on positive trends.
Social Media Monitoring: With billions of users worldwide, social media platforms are goldmines of public opinion. Sentiment analysis helps brands track and analyze social media mentions to understand how their products, services, or campaigns are perceived in real-time.
Market Research: Sentiment analysis is invaluable in market research, allowing companies to gauge consumer sentiment toward competitors, trends, and market changes. This can inform strategic decisions and help businesses stay ahead of the curve.
Political Analysis: Sentiment analysis is also used to analyze public opinion on political issues, candidates, and policies. It helps political analysts and strategists understand voter sentiment and adjust campaign strategies accordingly.
Customer Support: By analyzing sentiment in customer support interactions, businesses can identify dissatisfied customers and prioritize their issues, leading to improved customer satisfaction and retention.
The Process of Sentiment Analysis
Sentiment analysis typically involves several steps, from data collection to model training and analysis. Here's a high-level overview:
Data Collection: The first step is gathering the text data you want to analyze. This can come from various sources, such as social media, customer reviews, emails, or surveys.
Text Preprocessing: Raw text data is often messy, containing noise like punctuation, stopwords, and special characters. Preprocessing involves cleaning the data by removing or transforming these elements to ensure accurate analysis. Tokenization, stemming, and lemmatization are common preprocessing techniques.
Feature Extraction: Once the text is cleaned, the next step is to convert it into a format that a machine learning model can understand. This involves extracting features from the text, such as word frequency, n-grams, or TF-IDF (Term Frequency-Inverse Document Frequency).
Model Training: In this step, a machine learning model is trained on the preprocessed and feature-extracted text data. Popular models for sentiment analysis include Naive Bayes, Support Vector Machines, and neural networks.
Sentiment Classification: After the model is trained, it can be used to classify new text data into positive, negative, or neutral sentiment. The results can be analyzed to derive insights and inform decision-making.
Evaluation and Refinement: It's essential to evaluate the model's performance using metrics like accuracy, precision, recall, and F1 score. Based on the evaluation, the model can be fine-tuned and improved.
Challenges in Sentiment Analysis
While sentiment analysis is powerful, it comes with its challenges:
Sarcasm and Irony: Detecting sarcasm and irony is difficult for machines, as the intended sentiment often contrasts with the literal meaning of the text.
Context Dependency: The sentiment of a word or phrase can change depending on the context, making it challenging to accurately classify sentiment without understanding the surrounding text.
Ambiguity: Words can have multiple meanings (e.g., "bank" can refer to a financial institution or the side of a river), leading to potential misclassification of sentiment.
Language and Cultural Nuances: Sentiment analysis models trained on one language or cultural context may not perform well in another due to differences in language use and cultural expressions.
Sentiment Analysis in Python Full Script
Here's a Python script that demonstrates how to perform sentiment analysis using an inbuilt dataset. We'll use the nltklibrary and its movie_reviews dataset, which is a collection of movie reviews labeled as positive or negative. This script will involve preprocessing the text data, extracting features, training a model, and evaluating its performance.
import nltk
from nltk.corpus import movie_reviews
from nltk.classify import NaiveBayesClassifier
from nltk.classify.util import accuracy
from nltk import FreqDist, classify
from nltk.tokenize import word_tokenize
# Download the necessary NLTK data (if not already downloaded)
nltk.download('movie_reviews')
nltk.download('punkt')
# Load the movie_reviews dataset
def load_movie_reviews():
reviews = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
return reviews
# Define a function to extract features from the text
def extract_features(words):
return {word: True for word in words}
# Load the dataset
reviews = load_movie_reviews()
# Shuffle the dataset to ensure randomness
import random
random.shuffle(reviews)
# Define the feature sets
feature_sets = [(extract_features(review), category) for (review, category) in reviews]
# Split the dataset into training and testing sets (80% training, 20% testing)
train_set_size = int(len(feature_sets) * 0.8)
train_set, test_set = feature_sets[:train_set_size], feature_sets[train_set_size:]
# Train a Naive Bayes classifier
classifier = NaiveBayesClassifier.train(train_set)
# Evaluate the classifier on the test set
print(f"Accuracy: {accuracy(classifier, test_set):.2f}")
# Show the most informative features
classifier.show_most_informative_features(10)
# Function to classify new reviews
def classify_review(review):
features = extract_features(word_tokenize(review))
return classifier.classify(features)
# Test the classifier with some sample reviews
print(classify_review("This movie was absolutely wonderful, full of excitement and fun!"))
print(classify_review("This was a terrible movie, I hated every moment of it."))
Output for the above code:
Accuracy: 0.67
Most Informative Features
insulting = True neg : pos = 17.0 : 1.0
seagal = True neg : pos = 12.1 : 1.0
astounding = True pos : neg = 11.9 : 1.0
gripping = True pos : neg = 11.2 : 1.0
captures = True pos : neg = 11.0 : 1.0
outstanding = True pos : neg = 10.6 : 1.0
sucks = True neg : pos = 10.2 : 1.0
schumacher = True neg : pos = 10.0 : 1.0
seamless = True pos : neg = 10.0 : 1.0
turkey = True neg : pos = 9.8 : 1.0
pos
neg
Steps included in the script:
Once everything is set up, you can execute the script and see how the classifier performs on the inbuilt dataset. You can also modify the script to experiment with different classifiers, feature extraction techniques, or datasets.
Loading the Dataset: The script begins by loading the movie_reviews dataset from NLTK. This dataset contains 2,000 movie reviews, categorized as positive or negative.
Feature Extraction: We define a function, extract_features, which creates a feature set from a list of words. Each word in the review is treated as a feature with a boolean value of True.
Training and Testing: The dataset is shuffled to ensure randomness, then split into training and testing sets. We use 80% of the data for training and 20% for testing.
Training the Model: We train a Naive Bayes classifier on the training set. Naive Bayes is a simple yet effective algorithm for text classification tasks like sentiment analysis.
Evaluating the Model: The script prints the accuracy of the classifier on the test set. It also displays the most informative features, which are the words most indicative of positive or negative sentiment.
Classifying New Reviews: Finally, the script includes a classify_review function that allows you to classify the sentiment of new movie reviews.
This simple yet effective script provides a solid foundation for sentiment analysis in Python, demonstrating how to preprocess text, train a model, and make predictions on new data.
Conclusion
Sentiment analysis is a powerful tool that enables businesses, researchers, and analysts to gain valuable insights from text data by understanding the underlying emotions and opinions. By leveraging Python and its robust libraries, sentiment analysis becomes accessible and efficient, allowing for the processing of large datasets and the extraction of actionable insights. Whether applied to customer feedback, social media monitoring, or market research, sentiment analysis helps organizations make informed decisions, improve customer experiences, and stay ahead of trends. As the volume of text data continues to grow, mastering sentiment analysis will be increasingly essential for anyone looking to unlock the full potential of this data-driven world.
By embracing sentiment analysis, you can turn unstructured text into actionable insights, giving you a competitive edge in today's data-driven world.
Comments