Sets in Python are an essential data structure that offer unique features for handling collections of elements. Unlike lists or tuples, sets are unordered and unindexed, meaning they do not allow duplicates and do not maintain any specific order. This makes sets particularly useful when you need to ensure that your data contains only unique items. In this blog, we’ll explore the key features of sets, how to create and manipulate them, and some of the most common operations you can perform using Python sets.
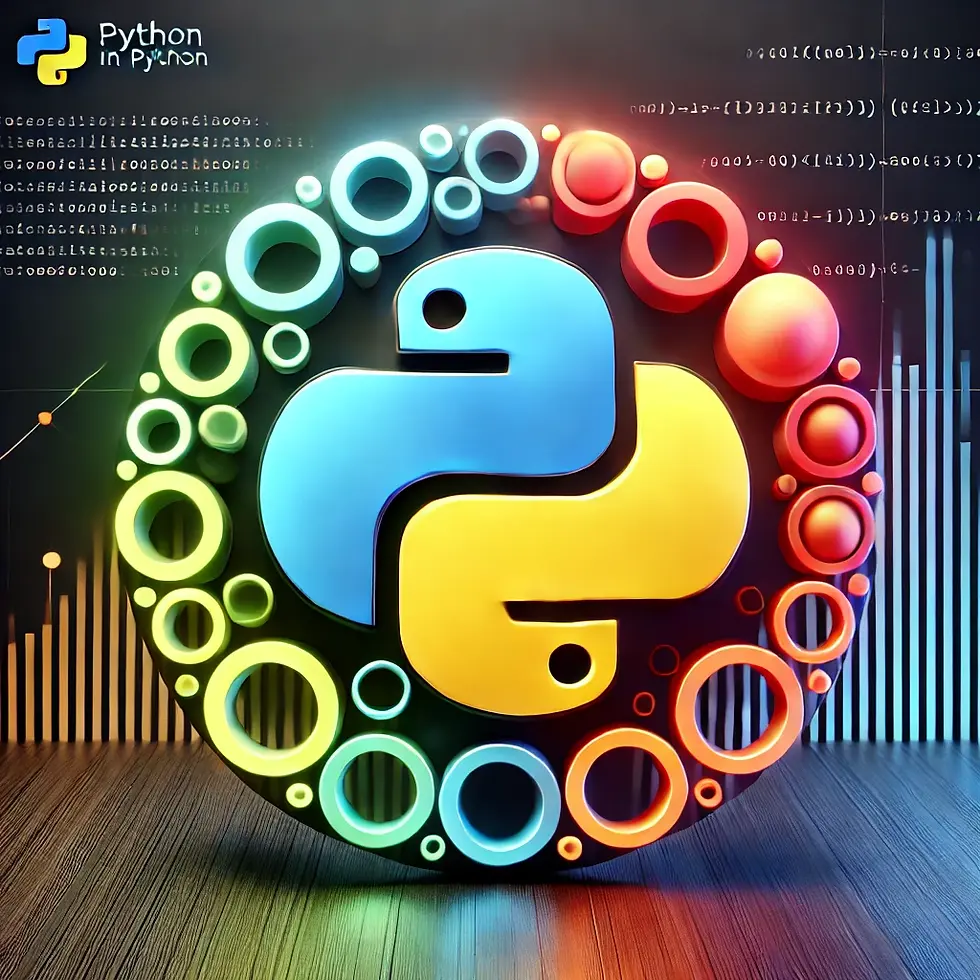
Table of Contents
What is a Set?
Creating a Set
Set Operations
Methods in Sets
Set Comprehension
When to Use Sets
Conclusion
1. What is a Set in Python?
A set in Python is a built-in data structure that holds an unordered collection of unique elements. Unlike lists or tuples, sets do not allow duplicates, meaning each item can only appear once within a set. Sets are mutable, allowing for the addition and removal of elements, but they do not maintain any specific order and cannot be indexed or sliced. Due to their unordered nature, sets are optimized for fast membership testing and are useful in scenarios where the uniqueness of items is important, such as eliminating duplicates from a list or performing set operations like union, intersection, and difference.
2. Creating a Set in Python
There are a few ways to create sets in Python:
Using Curly Braces
You can define a set using curly braces {}:
my_set = {1, 2, 3, 3, 4}
print(my_set) # Output: {1, 2, 3, 4} (duplicates removed)
Notice that the set automatically removed duplicate values.
Using the set() Function
You can create a set from an iterable such as a list or a string by passing it to the set() function:
# From a list
my_list = [1, 2, 2, 3, 4]
my_set = set(my_list)
print(my_set) # Output: {1, 2, 3, 4}
# From a string
my_str_set = set("hello")
print(my_str_set) # Output: {'h', 'e', 'o', 'l'}
3. Set Operations in Python
Sets support several mathematical operations like union, intersection, and difference. These operations are crucial when working with collections of data.
Union
The union of two sets is a set containing all unique elements from both sets.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set) # Output: {1, 2, 3, 4, 5}
Intersection
The intersection of two sets contains only the elements that are present in both sets.
intersection_set = set1.intersection(set2)
print(intersection_set) # Output: {3}
Difference
The difference between two sets contains the elements present in the first set but not in the second.
difference_set = set1.difference(set2)
print(difference_set) # Output: {1, 2}
Symmetric Difference
The symmetric difference contains elements that are in either of the sets but not in both.
sym_diff_set = set1.symmetric_difference(set2)
print(sym_diff_set) # Output: {1, 2, 4, 5}
4. Methods in Sets in Python
Python sets come with built-in methods that allow for easy manipulation and analysis of data.
add(): Adds an element to the set.
remove(): Removes an element from the set. Raises a KeyError if the element is not present.
discard(): Removes an element from the set. Does not raise an error if the element is not present.
pop(): Removes and returns a random element from the set.
clear(): Removes all elements from the set.
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
my_set.remove(3)
print(my_set) # Output: {1, 2, 4}
5. Set Comprehension in Python
Just like list comprehension, Python also supports set comprehension, which allows you to generate sets in a concise manner.
Example:
# Set comprehension to create a set of squares
squares = {x**2 for x in range(1, 6)}
print(squares) # Output: {1, 4, 9, 16, 25}
6. When to Use Sets in Python
Sets are particularly useful in the following scenarios:
Removing Duplicates: If you need to eliminate duplicate elements from a collection, sets automatically handle that.
Membership Testing: Checking whether an item is in a set is faster compared to lists or tuples because sets are optimized for this kind of operation.
Set Operations: When you need to perform mathematical operations like union, intersection, or difference, sets are the go-to data structure.
Conclusion
In conclusion, sets in Python offer a robust and efficient way to handle collections of unique elements. Their key features, such as automatic removal of duplicates, fast membership testing, and support for mathematical operations like union, intersection, and difference, make them an invaluable tool for developers. Whether you're working on data analysis, processing large datasets, or simply ensuring the uniqueness of elements, sets can significantly simplify your code and improve its performance. By mastering sets and their operations, you can write more concise, optimized, and maintainable Python programs.
By understanding how to create and manipulate sets, as well as when to use them, you can take advantage of their unique properties to improve your code’s performance and efficiency.
Comments