Chatbots are increasingly popular for providing instant user interaction. With HTML, CSS, and JavaScript, you can create a functional chatbot that responds to user inputs. This tutorial will walk you through building a simple chatbot interface and adding basic interactivity.
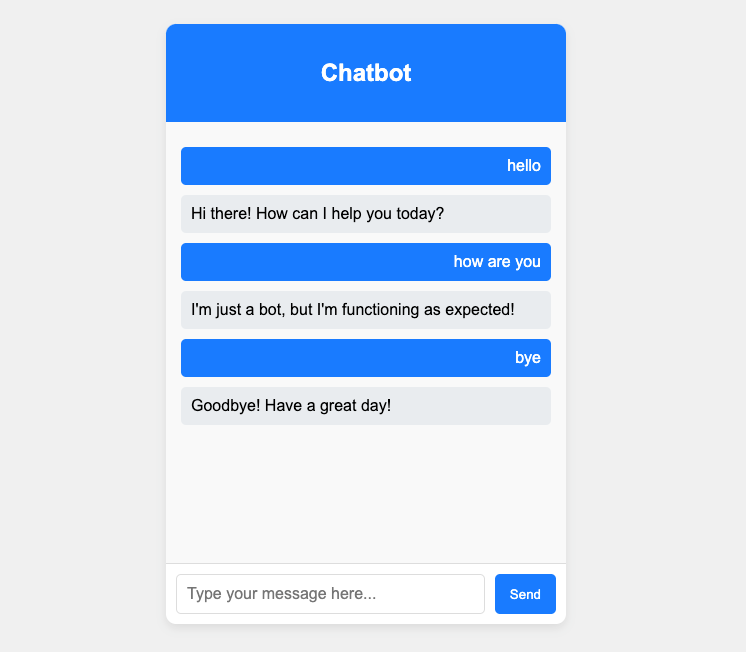
Getting Started with Building Chatbot with JavaScript, HTML, and CSS
Building a Simple Chatbot Using JavaScript, HTML, and CSS is an exciting way to dive into web development and create something interactive and fun. Using HTML, you can design the structure of the chatbot, like the chat window where messages appear, the input box for typing messages, and a button to send them. CSS allows you to bring the chatbot to life visually, adding colors, fonts, and layouts to make it engaging and user-friendly. JavaScript is where the magic happens—it processes user input, generates responses, and updates the chat in real time, making the experience dynamic. Whether it’s for a project, learning, or just experimenting, building a chatbot lets you explore how these technologies work together to create something functional and interactive, all while enhancing your coding skills in a creative way. Key features of a simple chatbot:
Interactive Chatbot Interface: A user-friendly chat window that displays messages in a clean and visually appealing format.
User Input Handling: Processes user messages entered into the chat and triggers appropriate responses.
Simple Pre-Defined Responses: Offers basic, pre-set replies for common inputs, showcasing fundamental chatbot functionality.
Step 1: Set Up Your Project
Create the following file structure:
chatbot/
├── index.html
├── style.css
├── script.js
Step 2: HTML Structure
Create the basic structure of the chatbot in index.html. The following HTML code is a simple yet effective structure for a chatbot interface. It includes a clean layout with a header displaying the chatbot's title, a main chat box for displaying conversation messages, and an input section where users can type and send their messages. The chat-container keeps all elements organized, while external CSS (style.css) is linked to ensure the design can be easily customized. Additionally, the script file (script.js) is linked at the end, enabling dynamic functionality, such as handling user input and updating the chat box. This thoughtful structure ensures a user-friendly and interactive experience.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chatbot</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="chat-container">
<div class="chat-header">
<h2>Chatbot</h2>
</div>
<div class="chat-box" id="chatBox"></div>
<div class="chat-input">
<input type="text" id="userInput" placeholder="Type your message here...">
<button id="sendBtn">Send</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 3: CSS Styling
Style the chatbot interface in style.css. This CSS stylesheet beautifully styles the chatbot interface, creating a clean, user-friendly design. The body ensures the layout is centered and responsive with a neutral background that enhances readability. The chat-container is styled as a white, card-like box with rounded corners and a subtle shadow for a polished appearance.
The chat-header uses a bold blue background with white text, giving the chatbot a professional and inviting feel. The chat-box section is scrollable to accommodate long conversations, with clear distinctions between user and bot messages through colors and alignment, making it easy to follow dialogues.
The chat-input section is both functional and attractive, with a flexible input field and a neatly styled button that changes color on hover, encouraging interaction. Overall, these styles combine simplicity and elegance to create a smooth and enjoyable user experience.
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.chat-container {
width: 400px;
height: 600px;
background-color: white;
border-radius: 10px;
box-shadow: 0 4px 10px rgba(0, 0, 0, 0.1);
display: flex;
flex-direction: column;
}
.chat-header {
background-color: #007bff;
color: white;
padding: 15px;
border-radius: 10px 10px 0 0;
text-align: center;
}
.chat-box {
flex: 1;
padding: 15px;
overflow-y: auto;
background-color: #f9f9f9;
}
.chat-box .message {
margin: 10px 0;
padding: 10px;
border-radius: 5px;
}
.chat-box .user {
background-color: #007bff;
color: white;
text-align: right;
}
.chat-box .bot {
background-color: #e9ecef;
text-align: left;
}
.chat-input {
display: flex;
padding: 10px;
border-top: 1px solid #ddd;
}
.chat-input input {
flex: 1;
padding: 10px;
font-size: 16px;
border: 1px solid #ddd;
border-radius: 5px;
}
.chat-input button {
padding: 10px 15px;
margin-left: 10px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.chat-input button:hover {
background-color: #0056b3;
}
Step 4: Add JavaScript Functionality
Write the chatbot's logic in script.js. This JavaScript code provides the core functionality for a simple chatbot, enabling a smooth and interactive experience.
HTML Element References:It selects key elements like the chat box (chatBox), the user input field (userInput), and the send button (sendBtn), ensuring they can be dynamically updated.
Predefined Responses:A dictionary of responses (botResponses) allows the bot to provide specific replies to common inputs like "hello" or "bye." For unrecognized inputs, it defaults to a generic response.
Dynamic Message Addition:The addMessage function creates and styles new message elements based on whether they are from the user or the bot. It also keeps the chat box scrolled to the latest message, ensuring smooth usability.
User Input Handling:The handleUserInput function retrieves and processes user messages. It trims and converts input to lowercase for consistent matching, displays the user’s message, clears the input field, and triggers the bot’s response with a slight delay for a natural conversational feel.
Event Listeners:
The sendBtn listener triggers the handleUserInput function when the button is clicked.
The keypress event listens for the "Enter" key, allowing users to send messages quickly without relying on the button.
const chatBox = document.getElementById('chatBox');
const userInput = document.getElementById('userInput');
const sendBtn = document.getElementById('sendBtn');
// Predefined responses
const botResponses = {
"hello": "Hi there! How can I help you today?",
"how are you": "I'm just a bot, but I'm functioning as
expected!",
"bye": "Goodbye! Have a great day!",
"default": "I'm sorry, I didn't understand that. Can you try rephrasing?"
};
// Function to add messages to the chatbox
function addMessage(message, sender) {
const messageDiv = document.createElement('div');
messageDiv.classList.add('message', sender);
messageDiv.textContent = message;
chatBox.appendChild(messageDiv);
chatBox.scrollTop = chatBox.scrollHeight; // Scroll to the
latest message
}
// Function to handle user input
function handleUserInput() {
const userText = userInput.value.trim().toLowerCase();
if (userText) {
addMessage(userInput.value, 'user');
userInput.value = '';
// Get bot response
const botResponse = botResponses[userText] || botResponses["default"];
setTimeout(() => addMessage(botResponse, 'bot'), 500);
}
}
// Event listener for send button
sendBtn.addEventListener('click', handleUserInput);
// Handle "Enter" key press
userInput.addEventListener('keypress', (event) => {
if (event.key === 'Enter') {
handleUserInput();
}
});
Step 5: Test the Chatbot
Open index.html in your browser.
Type a message like "hello" or "bye" in the input field and click Send or press Enter.
See the chatbot respond with pre-defined messages.
Step 6: Enhance Your Chatbot (Optional)
Here are some ideas to improve your chatbot:
Dynamic Responses: Use an AI API like OpenAI’s ChatGPT or Dialogflow to generate intelligent responses.
Styling: Add animations to the chat messages or customize the color scheme.
Storage: Save chat history using localStorage or a backend database.
Voice Input: Integrate voice recognition for more interactive communication.
Conclusion
You've successfully built a chatbot using JavaScript, HTML, and CSS, showcasing how these technologies work together to create an interactive experience. While this is a simple example, it lays the foundation for more sophisticated features. You can enhance its capabilities by integrating external APIs, such as those for weather updates, language translation, or AI-driven natural language processing. Adding functionalities like user authentication, saving conversation histories, or implementing a more advanced design can elevate the user experience further. Let me know if you'd like to explore these advanced features or brainstorm creative ways to expand your chatbot's potential!
Comments