Python is a versatile and widely-used programming language, known for its simplicity and readability. One of the fundamental data types in Python is the string, which represents text. In this blog post, we will explore strings in Python, how to manipulate them, and the powerful built-in functions Python provides to work with strings.
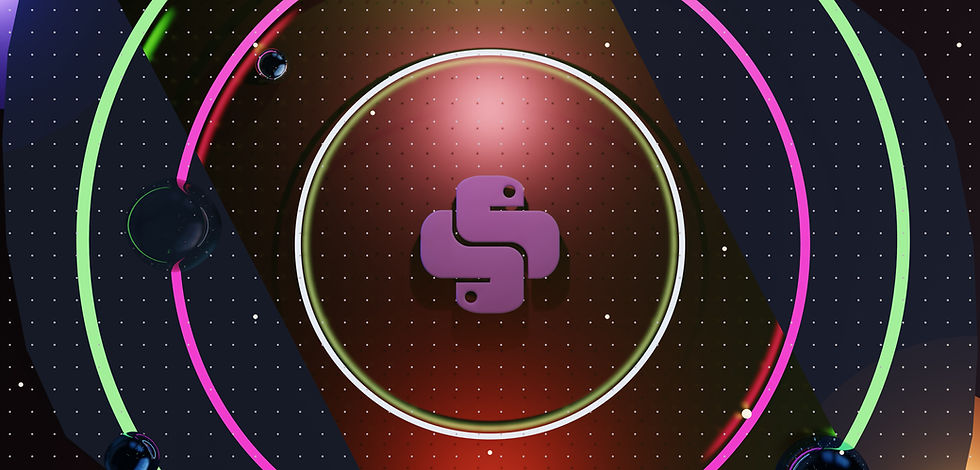
What is a String in Python?
In Python, a string is a sequence of characters enclosed in either single quotes (') or double quotes ("). Strings are immutable, meaning once they are created, their content cannot be changed.
# Examples of strings
string1 = "Hello, World!"
string2 = 'Python is awesome!'
Multiline Strings
Python also supports multiline strings, which are enclosed in triple quotes (""" or '''). These strings can span across multiple lines.
multiline_string = """This is a
multiline string in Python."""
print(multiline_string)
Output
This is a
multiline string in Python.
Basic String Operations in Python
1. Concatenation
Strings can be concatenated using the + operator.
greeting = "Hello"
name = "Alice"
message = greeting + ", " + name + "!"
print(message) # Output: Hello, Alice!
2. Repetition
You can repeat a string multiple times using the * operator.
repeated_string = "Ha" * 3
print(repeated_string) # Output: HaHaHa
3. String Length
To find the length of a string (number of characters), you can use the len() function.
length = len("Hello, World!")
print(length) # Output: 13
4. Accessing Characters
You can access individual characters of a string using indexing. Python uses zero-based indexing, meaning the first character of a string is at index 0.
word = "Python"
first_char = word[0] # Output: 'P'
last_char = word[-1] # Output: 'n'
5. Slicing Strings
You can extract a substring by using slicing. The syntax for slicing is [start:end], where start is the index of the first character, and end is the index of the character just after the last one you want to include.
word = "Python"
print(word[0:2]) # Output: 'Py'
print(word[2:]) # Output: 'thon'
print(word[:4]) # Output: 'Pyth'
Common String Methods in Python
Python provides a wide variety of methods for string manipulation. Here are some of the most commonly used ones:
1. upper() and lower()
These methods convert the string to uppercase and lowercase, respectively.
text = "Hello, World!"
print(text.upper()) # Output: 'HELLO, WORLD!'
print(text.lower()) # Output: 'hello, world!'
2. strip()
This method removes leading and trailing whitespace (or other characters).
text = " Hello, World! "
print(text.strip()) # Output: 'Hello, World!'
3. replace()
The replace() method replaces all occurrences of a substring with another substring.
text = "Hello, World!"
new_text = text.replace("World", "Python")
print(new_text) # Output: 'Hello, Python!'
4. split() and join()
split() breaks a string into a list of substrings based on a delimiter.
join() combines a list of strings into a single string with a specified delimiter.
text = "Python is fun"
words = text.split() # Splits at spaces by default
print(words) # Output: ['Python', 'is', 'fun']
sentence = " ".join(words)
print(sentence) # Output: 'Python is fun'
5. find() and count()
find() returns the index of the first occurrence of a substring, or -1 if not found.
count() returns the number of occurrences of a substring.
text = "Hello, World!"
index = text.find("World") # Output: 7
occurrences = text.count("o") # Output: 2
String Formatting in Python
Python provides multiple ways to format strings for output. Some of the most common methods include:
1. f-strings (Python 3.6+)
F-strings are a concise and readable way to embed expressions inside string literals using curly braces {}.
name = "Alice"
age = 25
message = f"My name is {name} and I am {age} years old."
print(message) # Output: 'My name is Alice and I am 25 years old.'
2. format()
The format() method is another way to format strings.
message = "My name is {} and I am {} years old.".format("Bob", 30)
print(message) # Output: 'My name is Bob and I am 30 years old.'
String Immutability in Python
As mentioned earlier, strings in Python are immutable, which means their content cannot be changed after they are created. If you need to modify a string, you must create a new one.
text = "Hello"
text[0] = "J" # This will raise an error
To work around this, you can concatenate or slice and combine strings.
new_text = "J" + text[1:]
print(new_text) # Output: 'Jello'
Conclusion
Strings are an essential part of Python programming, and understanding how to manipulate them effectively can greatly enhance your coding efficiency. In this post, we covered basic string operations, useful string methods, formatting options, and more.
With Python's rich set of string manipulation tools, you can easily perform complex text-based tasks with minimal code. Whether you're working on data processing, natural language processing, or web development, strings will be a fundamental aspect of your code.
Comments