Support Vector Machines (SVM) are a powerful class of supervised learning algorithms used for classification, regression, and outlier detection tasks. SVMs are known for their ability to create hyperplanes in a high-dimensional space that effectively separate different classes of data. Despite their complexity, SVMs are widely used due to their effectiveness, especially in problems where the data is not linearly separable.
In this blog, we’ll dive into the fundamentals of SVMs, understand how they work, and explore how to implement them using Python.
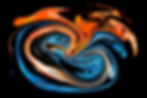
What is a Support Vector Machine (SVM)?
A Support Vector Machine is a supervised machine learning algorithm that can classify data points into different categories. The key idea behind SVMs is to find the hyperplane that best separates the data points into classes. In a two-dimensional space, this hyperplane is simply a line, but in higher dimensions, it's a plane or even a hyperplane.
Key Concepts of SVM:
Support Vectors: These are the data points that are closest to the hyperplane. They are critical in defining the optimal hyperplane.
Margin: The margin is the distance between the hyperplane and the nearest support vectors. SVM tries to maximize this margin to improve the classifier's generalization ability.
Hyperplane: This is the boundary that separates the different classes in the feature space.
Types of SVM:
Support Vector Machines (SVM) come in various types depending on the complexity of the problem and the nature of the data. The two main types of SVM are Linear SVMÂ and Non-Linear SVM.
Linear SVMÂ is used when the data is linearly separable, meaning it can be divided into distinct classes using a straight line (in 2D) or a hyperplane (in higher dimensions). Linear SVM aims to find the hyperplane that maximizes the margin between the classes. It works well for simple classification tasks where the classes can be easily separated by a straight line or plane.
Non-Linear SVM is used when the data cannot be separated linearly. In such cases, SVM employs a technique known as the kernel trick to map the data into a higher-dimensional space where it becomes linearly separable. Common kernels used in non-linear SVM include the Radial Basis Function (RBF), Polynomial, and Sigmoid kernels. These kernels allow SVM to handle complex problems such as image recognition and text classification, where the decision boundary is not a straight line. Both types of SVM are powerful tools in machine learning, with the choice of which to use depending on the nature of the data and the problem at hand.
How SVM Works
SVM aims to find the optimal hyperplane that best divides the data into distinct classes. In simple terms, SVM tries to find the line (in 2D) or plane (in 3D) that maximizes the distance between data points of different classes. The hyperplane with the maximum margin is considered the best one. The process involves:
Training: The algorithm first learns from the data by finding the support vectors and calculating the margin.
Classification: Once the optimal hyperplane is found, the model can classify new data points based on which side of the hyperplane they fall.
Kernel Trick:
For data that isn’t linearly separable, SVMs use a technique called the kernel trick. This technique transforms the data into a higher-dimensional space, making it easier to find a separating hyperplane. Popular kernels include:
Linear Kernel: Used when data is linearly separable.
Polynomial Kernel: Maps data to a higher-dimensional space.
Radial Basis Function (RBF) Kernel: Effective for non-linear data.
Implementing SVM in Python
Let’s now look at how to implement a simple SVM for a classification task using Python and the scikit-learn library.
Example: SVM for Iris Dataset
# Import necessary libraries
import numpy as np
import matplotlib.pyplot as plt
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import classification_report, accuracy_score
# Load the iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create the SVM model with a linear kernel
svm_model = SVC(kernel='linear')
# Train the model
svm_model.fit(X_train, y_train)
# Make predictions
y_pred = svm_model.predict(X_test)
# Evaluate the model
print("Accuracy:", accuracy_score(y_test, y_pred))
print("Classification Report:\n", classification_report(y_test, y_pred))
Explanation:
We load the Iris dataset, which contains data about different species of iris flowers, including features like petal length and width.
The dataset is split into training and testing sets using train_test_split.
An SVM model is created using a linear kernel. You can also experiment with other kernels like RBF.
The model is trained using the fit method, and predictions are made on the test data.
Finally, we evaluate the model’s performance using the accuracy_score and classification_report methods.
Advantages of SVM
Effective in high-dimensional spaces: SVMs work well when there are more features (dimensions) than data points.
Memory Efficient: Since SVM only uses support vectors to construct the hyperplane, it’s memory efficient.
Robust to Overfitting: By focusing on the margin and support vectors, SVM tends to generalize well, even with fewer data points.
Disadvantages of SVM
Training Time: SVM can be slow when working with large datasets due to the complexity of finding the optimal hyperplane.
Choosing the Right Kernel: Selecting an appropriate kernel and tuning its parameters (like the regularization parameter) can be challenging.
Sensitive to Noise: SVM can be sensitive to noise in the data, especially if there are outliers.
Conclusion
Support Vector Machines (SVM) are a powerful and versatile tool in machine learning, widely used for classification tasks. They are particularly useful for high-dimensional data and work well when the data is linearly separable. Although SVMs can be computationally expensive and sensitive to noisy data, their ability to create robust classifiers with large margins makes them a popular choice in many applications.
By understanding how SVM works, experimenting with different kernels, and learning how to implement it in Python, you can add a valuable tool to your machine learning toolkit!