TensorFlow in Python: A Powerful Framework for Deep Learning
- Samuel Black
- Aug 12, 2024
- 5 min read
In the world of deep learning, TensorFlow stands out as one of the most powerful and widely-used frameworks. Developed by the Google, TensorFlow has become the go-to tool for building machine learning and deep learning models, offering a robust and flexible platform for researchers, developers, and data scientists. In this blog, we’ll explore what TensorFlow is, why it’s so widely adopted, and how to get started with building your first deep learning model using TensorFlow in Python.
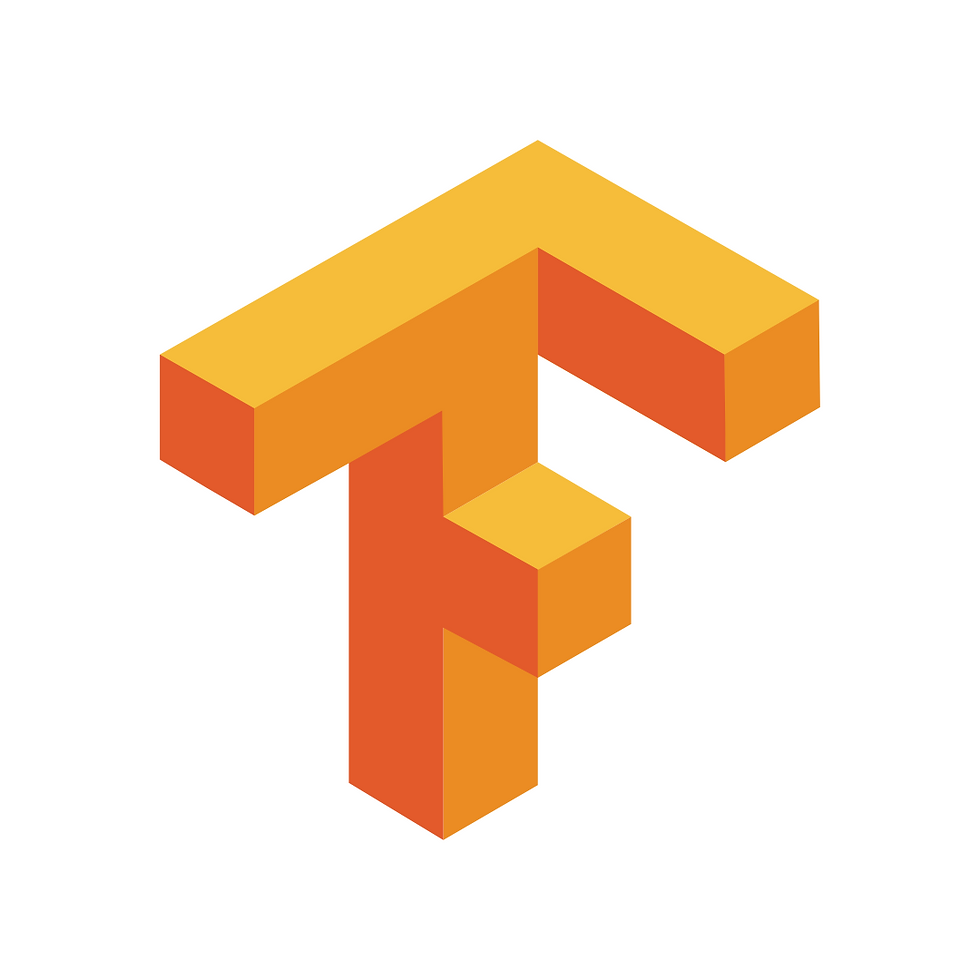
What is TensorFlow in Python?
TensorFlow is an open-source framework designed for machine learning and deep learning, widely used for building and deploying a variety of models. Created by the Google, TensorFlow provides a comprehensive and flexible ecosystem for both researchers and developers to create and implement complex neural networks and machine learning algorithms.
In Python, TensorFlow acts as a powerful tool for handling large-scale numerical computations, especially those involving matrix operations and neural network training. It supports a range of applications from simple linear regression to intricate models like convolutional neural networks (CNNs) and recurrent neural networks (RNNs). TensorFlow's architecture allows it to run on multiple platforms, including CPUs, GPUs, and even specialized hardware like TPUs (Tensor Processing Units), making it highly scalable for both small and large projects.
One of TensorFlow's strengths is its ability to represent computations as data flow graphs, where nodes represent mathematical operations and edges represent the data (tensors) flowing between these operations. This graph-based approach enables efficient computation, distributed execution, and easy visualization, making TensorFlow a preferred choice for implementing deep learning models in Python. Some of the key features of tensorflow include:
Scalability: TensorFlow is designed to handle large-scale machine learning tasks, making it suitable for both research and production environments. It supports distributed computing, allowing models to be trained across multiple devices and clusters.
Flexibility: TensorFlow provides a flexible framework for defining computational graphs, which can be used to create a wide range of machine learning models. This includes everything from simple linear models to complex multi-layer neural networks.
Extensive Ecosystem: TensorFlow has a rich ecosystem of tools and libraries, such as TensorFlow Lite for mobile and embedded devices, TensorFlow.js for JavaScript development, and TensorFlow Extended (TFX) for end-to-end ML pipelines.
Community and Support: With a large and active community, TensorFlow benefits from extensive documentation, tutorials, and third-party support. The framework is continuously updated with new features and improvements, driven by both Google and the broader open-source community.
Why Use TensorFlow?
TensorFlow is chosen by many developers and researchers because of its versatility, scalability, and extensive support for machine learning tasks. Whether you're building a simple model for a small dataset or a complex deep learning architecture for big data, TensorFlow provides the tools and resources needed to succeed. Its ability to scale from individual GPUs to large clusters makes it ideal for both research and production environments.
Additionally, TensorFlow’s integration with other Google technologies, like TensorFlow Serving for model deployment and TensorFlow Hub for reusable model components, makes it a powerful choice for building and deploying machine learning applications in real-world settings.
Getting Started with TensorFlow in Python
Getting started with TensorFlow in Python is straightforward, making it accessible for both beginners and experienced developers. To begin, you need to install TensorFlow using pip, preferably within a virtual environment to manage dependencies effectively. With TensorFlow installed, you can immediately start building and training machine learning models. TensorFlow’s high-level API, Keras, simplifies the process of constructing neural networks, allowing you to focus on designing and optimizing your models rather than worrying about the underlying complexities. Whether you're working on small projects or scaling up to larger applications, TensorFlow provides the tools and resources to help you get up and running quickly.
Installation : Following command installs the latest version of TensorFlow, which you can then import into your Python scripts.
pip install tensorflow
Building a Simple Neural Network with TensorFlow in Python
Building a simple neural network with TensorFlow in Python is an excellent way to dive into deep learning. Using TensorFlow’s high-level Keras API, you can quickly create a neural network model with just a few lines of code. Start by defining the model using a Sequential structure, which allows you to stack layers easily. A common approach is to use a Flatten layer to convert input data into a one-dimensional array, followed by one or more Dense layers for processing, with ReLU as the activation function. The final layer typically uses softmax activation for multi-class classification. After defining the model, compile it with an optimizer (like Adam) and a loss function, then train it on your dataset. This simple approach lets you build a neural network to solve tasks like image classification, making TensorFlow a powerful yet accessible tool for deep learning projects. Let’s walk through creating a simple neural network model to classify images from the MNIST dataset, a classic dataset in the field of machine learning.
Step 1: Import Libraries
# Import necessary packages
import tensorflow as tf
from tensorflow.keras import layers, models
from tensorflow.keras.datasets import mnist
Step 2: Load and Preprocess the Data
# Load mnist dataset
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# Normalize data
train_images = train_images.reshape((60000, 28, 28, 1)).astype('float32') / 255
test_images = test_images.reshape((10000, 28, 28, 1)).astype('float32') / 255
Step 3: Define the Model
# Define sequential model model = models.Sequential([
layers.Flatten(input_shape=(28, 28, 1)),
layers.Dense(128, activation='relu'),
layers.Dense(10, activation='softmax')
])
Step 4: Compile the Model
# Model compilation
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Step 5: Train the Model
# Train the model
model.fit(train_images, train_labels, epochs=5, batch_size=32)
Output for the above code:
Epoch 1/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 6s 3ms/step - accuracy: 0.8761 - loss: 0.4347
Epoch 2/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 10s 3ms/step - accuracy: 0.9633 - loss: 0.1231
Epoch 3/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 10s 3ms/step - accuracy: 0.9772 - loss: 0.0772
Epoch 4/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 11s 3ms/step - accuracy: 0.9834 - loss: 0.0559
Epoch 5/5
1875/1875 ━━━━━━━━━━━━━━━━━━━━ 5s 3ms/step - accuracy: 0.9870 - loss: 0.0435
Step 6: Evaluate the Model
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f'Test accuracy: {test_acc}')
Output for the above code:
313/313 ━━━━━━━━━━━━━━━━━━━━ 1s 2ms/step - accuracy: 0.9737 - loss: 0.0798
Test accuracy: 0.9778000116348267
This code demonstrates how to build a simple neural network using TensorFlow’s high-level Keras API. The model is trained on the MNIST dataset, which consists of 28x28 grayscale images of handwritten digits, and evaluated on a separate test set to measure its accuracy.
Conclusion
TensorFlow in Python provides a robust and versatile platform for developing and deploying deep learning models. Its combination of flexibility, scalability, and ease of use makes it an ideal choice for a wide range of applications, from research to production. By leveraging TensorFlow’s high-level Keras API, developers can quickly build, train, and optimize neural networks with minimal complexity. Whether you're just starting out in machine learning or looking to tackle advanced AI challenges, TensorFlow equips you with the tools needed to succeed in the ever-evolving field of deep learning. Embrace TensorFlow to unlock the full potential of your data and drive innovation in your projects. By offering robust support for large-scale computations and a wide range of applications, TensorFlow continues to be a leading tool in the AI community. Whether you're just starting with deep learning or looking to implement complex models in production, TensorFlow provides the tools and resources needed to achieve your goals.
Comments