Terminal: An Introduction to Bash Scripting
- Samul Black
- 3 days ago
- 6 min read
Ever find yourself typing the same sequence of commands over and over in your terminal? Wishing there was a way to make your computer handle those repetitive tasks for you? Well, you've stumbled upon the magic of Bash scripting and automation!
In the world of Unix-like operating systems (like Linux and macOS), Bash (Bourne Again SHell) isn't just a command interpreter; it's a powerful scripting language waiting to be harnessed. Learning to write Bash scripts is like giving yourself a superpower – the ability to automate tedious processes, manage your system more efficiently, and ultimately, reclaim valuable time.
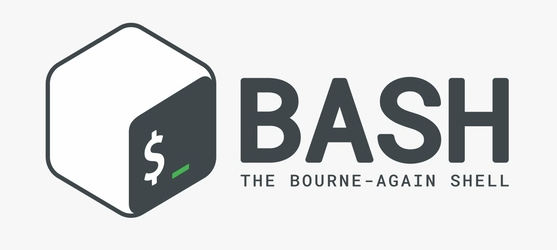
What is Bash Scripting and Automation?
At its core, Bash scripting involves writing a sequence of commands in a file that the Bash interpreter can execute. This allows you to automate tasks that you would otherwise perform manually through the command line. Automation through Bash can range from simple file manipulations to complex system administration tasks.
At its heart, a Bash script is simply a text file containing a series of commands that the Bash interpreter executes sequentially. Think of it as writing down a recipe for your computer to follow. Instead of manually typing each step, you write them down once, and Bash takes care of the rest.
Why Embrace Bash Scripting?
The benefits of venturing into Bash scripting are numerous:
Automation: This is the big one! Automate backups, file manipulations, software installations, system monitoring, and countless other tasks.
Efficiency: Reduce the time and effort spent on repetitive operations. Let your scripts handle the grunt work.
Consistency: Ensure tasks are performed the same way every time, minimizing the risk of human error.
Customization: Tailor scripts to your specific needs and workflows.
System Administration: A fundamental skill for system administrators, enabling them to manage and maintain systems effectively.
Development Workflows: Integrate scripting into your development process for tasks like building, testing, and deploying applications.
The Building Blocks of Bash Scripts
Even without diving into specific automation examples just yet, understanding the core concepts of Bash scripting will lay a solid foundation:
The Shebang: Every good Bash script starts with #!/bin/bash. This crucial line tells the system which program should execute the script.
Commands: Bash scripts are built upon the familiar commands you use in your terminal – ls, cd, mkdir, grep, and many more.
Variables: Store and manipulate data within your scripts using variables. Think of them as containers holding information.
Input and Output: Interact with your scripts by providing input and displaying output using commands like read and echo.
Control Flow: Direct the logic of your scripts using conditional statements (if/elif/else) and loops (for, while, until). These structures allow your scripts to make decisions and repeat actions.
Functions: Organize your code into reusable blocks, making your scripts cleaner and easier to manage.
Command Substitution: Capture the output of commands and use it as input for other commands or variables.
Exit Status: Understand how commands signal success or failure, allowing your scripts to react accordingly.
Getting Started with Bash Script
Getting started with Bash scripting is easier than you might think. All you need is a text editor and a terminal. Create a simple file, add the shebang line, write a few basic commands, make it executable, and run it. That's the first step on your journey to terminal mastery!
Create a File: Open a text editor (like nano, vim, gedit, or even a GUI text editor) and create a new file. Let's name it hello.sh. The .sh extension is a convention for Bash scripts, making them easily identifiable.
The Shebang: The very first line of your script should be the "shebang" line:
#!/bin/bash
This line tells the system which interpreter to use to execute the script. In this case, it's /bin/bash.
Write Your First Command: Let's add a simple command to print a message:
#!/bin/bash
echo "Hello, world!"
Save and Make Executable: Save the file. Now, you need to make it executable. Open your terminal and navigate to the directory where you saved hello.sh. Then, run the following command:
chmod +x hello.sh
The chmod +x command adds execute permissions to the file.
Run Your Script: Now you can execute your script:
./hello.sh
You should see the output:
Hello, world!
Basic Bash Scripting Concepts
Commands: Bash scripts are built from standard Unix/Linux commands. You can use any command you would normally type in the terminal within a script (e.g., ls, cd, mkdir, rm, cp, grep, sed, awk, etc.).
Comments: You can add comments to your scripts using the # symbol. Anything after # on a line is ignored by the interpreter.
#!/bin/bash # This is a comment
echo "This line will be executed." # Another comment here
Variables: You can store and manipulate data using variables.
Assignment: Assign a value to a variable using =:
name="Alice"
age=30
message="Hello, $name! You are $age years old."
echo "$message"
Important: There should be no spaces around the = sign. To access the value of a variable, use a $ prefix (e.g., $name or ${name}). Using curly braces ${} is often safer, especially when the variable name is followed by other characters.
Environment Variables: Bash also has environment variables (e.g., HOME, USER, PATH) that provide information about the system and user environment. You can access them in the same way: $HOME, $USER, etc.
Input and Output (I/O):
echo: Used to display output to the terminal.
read: Used to get input from the user.
#!/bin/bash
echo "Enter your name:"
read username
echo "Hello, $username!"
Control Flow: Bash provides structures to control the flow of execution in your scripts.
if, elif, else, fi (Conditional Statements):
#!/bin/bash
age=25
if [ $age -ge 18 ]; then
echo "You are an adult."
elif [ $age -lt 13 ]; then
echo "You are a child."
else
echo "You are a teenager."
fi
Important: Notice the spaces around the square brackets [ and ], and the use of -ge (greater than or equal), -lt (less than), -eq (equal), -ne (not equal), -gt (greater than), -le (less than or equal) for numerical comparisons. For string comparisons, you can use = (equal) and != (not equal).
for Loops: Used to iterate over a sequence of items.
#!/bin/bash
for fruit in apple banana cherry; do
echo "I like $fruit."
done
for i in {1..5}; do
echo "Number: $i"
done
while Loops: Execute a block of code as long as a condition is true.
#!/bin/bash
count=0
while [ $count -lt 5 ]; do
echo "Count is: $count"
count=$((count + 1))
done
until Loops: Execute a block of code until a condition becomes true.
#!/bin/bash
count=0
until [ $count -ge 5 ]; do
echo "Count is: $count"
count=$((count + 1))
done
case Statements: Useful for handling multiple conditions based on the value of a variable.
#!/bin/bash
os=$(uname -s)
case "$os" in
Linux*)
echo "You are running Linux."
;;
Darwin*)
echo "You are running macOS."
;;
*)
echo "Unknown operating system."
;;
esac
uname is a Unix command that displays system information.
The -s option specifically returns the kernel name (i.e., the operating system name).
(*, ?, etc.), the best approach when you want pattern matching.
Functions: You can define reusable blocks of code as functions.
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "Bob" greet "Charlie"
Here, $1 refers to the first argument passed to the function.
Command Substitution: You can capture the output of a command and use it within your script using $() or backticks `.
#!/bin/bash
current_date=$(date +%Y-%m-%d)
echo "Today's date is: $current_date"
file_count=`ls -l | grep "^-" | wc -l`
echo "Number of regular files in this directory: $file_count"
Exit Status: Every command in Bash returns an exit status. A status of 0 usually indicates success, while non-zero values indicate an error. You can access the exit status of the last executed command using $?.
#!/bin/bash
ls non_existent_file
if [ $? -ne 0 ]; then
echo "Error: The previous command failed."
fi
Conclusion
In essence, Bash scripting empowers you to transform your terminal from a command-line interface into a powerful automation engine. By understanding the core concepts – from the essential shebang to the intricacies of control flow and command substitution – and by putting these concepts into practice, you can unlock a world of efficiency and productivity. The ability to automate repetitive tasks, manage system operations, and customize workflows makes Bash scripting an invaluable skill for anyone working in a Unix-like environment.
👋 Do not Hesitate to Contact us for Further Assistance.
Whether you're just getting started with Bash or you're already experimenting with automation and scripting, we're here to help you grow your skills and tackle your challenges. If you:
Have questions about the concepts in this blog
Need help troubleshooting your shell setup
Want personalised training, tutorials, or consulting
Or simply want to share your feedback or success story
📩 Email us at: contact@colabcodes.com or visit this link for a specified plan.
We're always excited to connect with fellow learners, developers, and tech enthusiasts. Let's keep the conversation going!
Comments