Text Classification with Python: A Beginner's Guide
- Samuel Black
- Jul 31, 2024
- 4 min read
Text classification is a common task in natural language processing (NLP) where the goal is to categorize text into predefined classes or categories. It's widely used in various applications, such as spam detection, sentiment analysis, topic categorization, and more. In this beginner's guide, I'll walk you through the basics of text classification with Python, covering key steps and providing code examples.
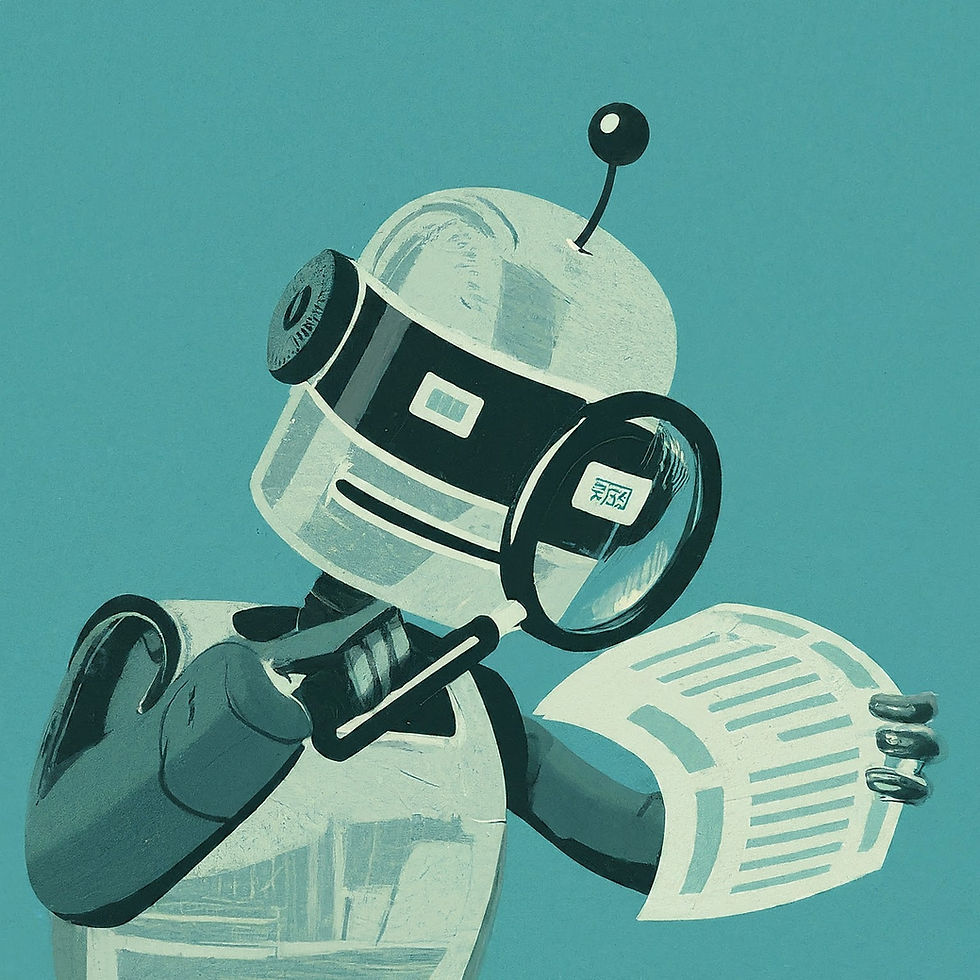
What is Text Classification?
Text classification is a process in natural language processing (NLP) that involves categorizing text into predefined categories or labels based on its content. It is a supervised learning task where a model is trained on a labeled dataset containing text samples and their corresponding labels. The trained model can then predict the category of new, unseen text based on learned patterns and features. Text classification has numerous applications, including spam detection, sentiment analysis, topic identification, and language detection, among others. It plays a crucial role in organizing and filtering information, enabling automated decision-making processes, and enhancing user experiences in various fields such as social media, customer service, and content management.
Text Classification with Python
Text classification with Python involves categorizing text into predefined categories using machine learning techniques. The process typically starts with data collection, followed by preprocessing steps such as tokenization, removal of stopwords, and vectorization to convert text into numerical format. Using libraries like Scikit-learn and NLTK, various machine learning algorithms, such as Naive Bayes, Support Vector Machines, or deep learning models, can be trained on the processed text data. After training, these models can predict the category of new, unseen text. Evaluation metrics like accuracy, precision, and recall help assess the model's performance. Text classification is widely used in applications like spam detection, sentiment analysis, and topic categorization, making it a valuable tool in natural language processing.
Getting Started with Text Classification with Python
To get started with text classification in Python, you need a few essential tools and concepts. First, you'll require a Python development environment, which can be set up using popular integrated development environments (IDEs) like PyCharm or Jupyter Notebook. Next, install key libraries such as NumPy, Pandas, Scikit-learn, and NLTK, which are vital for data manipulation, machine learning, and text processing. Understanding basic NLP concepts like tokenization, stopword removal, and vectorization is crucial, as these are the preprocessing steps that convert raw text into a format suitable for machine learning models. Additionally, you'll need a labeled dataset for training and testing your model, as well as familiarity with evaluation metrics to assess the model's performance. Some key requirements:
Python Development Environment: IDEs like PyCharm or Jupyter Notebook.
Libraries: NumPy, Pandas, Scikit-learn, NLTK.
NLP Concepts: Tokenization, stopword removal, vectorization.
Dataset: Labeled data for training and testing.
Evaluation Metrics: Understanding accuracy, precision, recall, and F1 score.
1. Setting Up the Environment
First, ensure you have Python installed. You'll also need some key libraries:
pip install numpy pandas scikit-learn nltk
NumPy and Pandas: For data manipulation.
Scikit-learn: For machine learning algorithms.
NLTK (Natural Language Toolkit): For text processing.
2. Data Collection
For this guide, let's use the 20 Newsgroups dataset, a common benchmark dataset for text classification.
from sklearn.datasets import fetch_20newsgroups
# Fetch the dataset
newsgroups = fetch_20newsgroups(subset='train', categories=['alt.atheism', 'sci.space'])
X, y = newsgroups.data, newsgroups.target
Here, X contains the text data, and y contains the corresponding labels.
3. Data Preprocessing
Text data needs to be preprocessed before it can be fed into a machine learning model. Common preprocessing steps include:
Tokenization: Splitting text into words.
Removing stopwords: Removing common but unimportant words (e.g., "the", "and").
Vectorization: Converting text into numerical format.
from sklearn.feature_extraction.text import CountVectorizer
from nltk.corpus import stopwords
# Tokenization and stopwords removal
vectorizer = CountVectorizer(stop_words='english')
X_vectorized = vectorizer.fit_transform(X)
Here, CountVectorizer converts text to a matrix of token counts, effectively vectorizing the text.
4. Splitting the Data
It's important to split your data into training and testing sets to evaluate the model's performance.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X_vectorized, y, test_size=0.2, random_state=42)
5. Building and Training the Model
For beginners, a simple yet effective algorithm is Naive Bayes. It works well with text classification due to its simplicity and effectiveness.
from sklearn.naive_bayes import MultinomialNB
# Initialize the model
model = MultinomialNB()
# Train the model
model.fit(X_train, y_train)
6. Evaluating the Model
After training the model, it's essential to evaluate its performance using metrics like accuracy, precision, recall, and F1 score.
from sklearn.metrics import accuracy_score, classification_report
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
report = classification_report(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print('Classification Report:')
print(report)
Output of the above code:
Accuracy: 1.0
Classification Report:
precision recall f1-score support
0 1.00 1.00 1.00 86
1 1.00 1.00 1.00 129
accuracy 1.00 215
macro avg 1.00 1.00 1.00 215
weighted avg 1.00 1.00 1.00 215
7. Improving the Model
You can improve the model by:
Tuning hyperparameters: Adjusting model parameters to optimize performance.
Using different vectorization methods: Such as TF-IDF.
Trying other algorithms: Like Support Vector Machines (SVM), Random Forests, or deep learning methods.
In conclusion, text classification in Python is a powerful technique that enables the automatic categorization of textual data into predefined classes. By leveraging tools and libraries like Scikit-learn and NLTK, alongside understanding key NLP concepts, you can build robust models for a variety of applications, from spam detection to sentiment analysis. This beginner's guide has covered the foundational steps, including setting up your environment, preprocessing text, and training a model. As you gain experience, exploring more sophisticated algorithms, fine-tuning parameters, and experimenting with different datasets will enhance your skills and the accuracy of your models. The journey into text classification is both challenging and rewarding, offering endless opportunities to apply machine learning to real-world problems.
Comments