Text preprocessing is a crucial step in Natural Language Processing (NLP) and machine learning. It involves preparing raw text data for analysis or modeling by transforming it into a format that is more suitable for processing. Effective preprocessing can significantly impact the performance of your NLP models. In this blog, we'll explore various text preprocessing techniques using Python, primarily focusing on libraries like NLTK and spaCy.
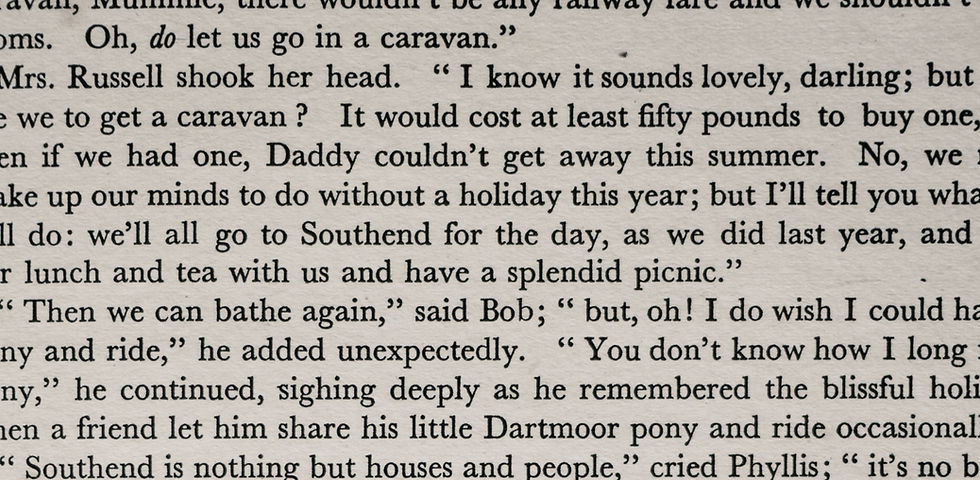
What is Text Preprocessing?
Text preprocessing is the initial step in preparing raw text data for analysis or machine learning. It involves transforming unstructured text into a structured format that can be effectively analyzed by models. This process includes various techniques such as tokenization, stop words removal, stemming, lemmatization, and normalization. The goal of text preprocessing is to clean and standardize the data, reduce its complexity, and enhance the performance of NLP models by ensuring that the input is consistent and meaningful. Raw text data often contains a lot of noise and variability. Preprocessing helps to:
Standardize Text: Convert different forms of text into a consistent format.
Reduce Complexity: Simplify text by removing unnecessary details.
Improve Model Performance: Enhance the quality of input data for better results in NLP models.
Importance of Preprocessing in NLP
Preprocessing is a critical step in Natural Language Processing (NLP) because it directly impacts the effectiveness and accuracy of NLP models. Raw text data is often messy and inconsistent, containing noise like punctuation, special characters, and irrelevant words that can confuse models and degrade performance. Preprocessing techniques such as tokenization, stop words removal, and lemmatization help standardize and clean the text, making it more suitable for analysis. By simplifying and structuring the data, preprocessing not only improves the quality of the input but also enhances the model's ability to learn patterns, leading to more reliable and meaningful outcomes in NLP tasks.
Key Text Preprocessing Techniques in Python using spaCy and nltk Libraries
Key text preprocessing techniques in Python are essential for transforming raw text data into a format suitable for analysis or machine learning. These techniques include tokenization, which breaks text into words or sentences; stop words removal, which filters out common, insignificant words like "the" or "and"; stemming and lemmatization, which reduce words to their root forms to unify variations of the same word; and removing punctuation and special characters, which cleans the text by eliminating irrelevant symbols. These processes help standardize and simplify text data, ultimately improving the performance of NLP models and analyses.
1. Tokenization
Tokenization is the process of breaking text into smaller units, such as words or sentences. This is the foundational step in text preprocessing.
Using NLTK:
import nltk
from nltk.tokenize import word_tokenize, sent_tokenize
text = "Hello! Welcome to the world of Natural Language Processing with Python. It's amazing, isn't it?"
# Sentence tokenization
sentences = sent_tokenize(text)
print("Sentences:", sentences)
# Word tokenization
words = word_tokenize(text)
print("Words:", words)
Output for the above code:
Sentences: ['Hello!', 'Welcome to the world of Natural Language Processing with Python.', "It's amazing, isn't it?"]
Words: ['Hello', '!', 'Welcome', 'to', 'the', 'world', 'of', 'Natural', 'Language', 'Processing', 'with', 'Python', '.', 'It', "'s", 'amazing', ',', 'is', "n't", 'it', '?']
Using spaCy:
import spacy
# Load spaCy model
nlp = spacy.load('en_core_web_sm')
# Tokenization
doc = nlp(text)
sentences = [sent.text for sent in doc.sents]
words = [token.text for token in doc]
print("Sentences:", sentences)
print("Words:", words)
Output for the above code:
Sentences: ['Hello!', 'Welcome to the world of Natural Language Processing with Python.', "It's amazing, isn't it?"]
Words: ['Hello', '!', 'Welcome', 'to', 'the', 'world', 'of', 'Natural', 'Language', 'Processing', 'with', 'Python', '.', 'It', "'s", 'amazing', ',', 'is', "n't", 'it', '?']
2. Stop Words Removal
Stop words are common words that often don't contribute significant meaning to a text and are usually removed to focus on the important words.
Using NLTK:
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
print("Filtered Words:", filtered_words)
Output for the above code:
Filtered Words: ['Hello', '!', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', '.', "'s", 'amazing', ',', "n't", '?']
Using spaCy:
filtered_words = [token.text for token in doc if not token.is_stop]
print("Filtered Words:", filtered_words)
Output for the above code:
Filtered Words: ['Hello', '!', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', '.', 'amazing', ',', '?']
3. Stemming and Lemmatization
Stemming and lemmatization reduce words to their base or root forms. Stemming removes suffixes (e.g., "running" becomes "run"), while lemmatization considers the context (e.g., "better" becomes "good").
Using NLTK(Stemming):
from nltk.stem import PorterStemmer
ps = PorterStemmer()
stemmed_words = [ps.stem(word) for word in filtered_words]
print("Stemmed Words:", stemmed_words)
Output for the above code:
Stemmed Words: ['hello', '!', 'welcom', 'world', 'natur', 'languag', 'process', 'python', '.', 'amaz', ',', '?']
Using NLTK(Lemmatization):
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in filtered_words]
print("Lemmatized Words:", lemmatized_words)
Output for the above code:
Lemmatized Words: ['hello', '!', 'welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', '.', 'amazing', ',', '?']
Using spaCy(Stemming):
lemmatized_words = [token.lemma_ for token in doc if not token.is_stop]
print("Lemmatized Words:", lemmatized_words)
Output for the above code:
Lemmatized Words: ['hello', '!', 'welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', '.', 'amazing', ',', '?']
4. Removing Punctuation and Special Characters
Punctuation and special characters can be removed to clean up the text.
Using NLTK:
import string
cleaned_words = [word for word in filtered_words if word not in string.punctuation]
print("Cleaned Words:", cleaned_words)
Output for the above code:
Cleaned Words: ['Hello', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', 'amazing']
Using spaCy:
print("Cleaned Words:", cleaned_words)
Output for the above code:
Cleaned Words: ['Hello', 'Welcome', 'world', 'Natural', 'Language', 'Processing', 'Python', 'amazing']
5. Text Normalization
Normalization involves converting text to a consistent format, such as lowercasing all words.
Using NLTK:
normalized_words = [word.lower() for word in cleaned_words]
print("Normalized Words:", normalized_words)
Output for the above code:
Normalized Words: ['hello', 'welcome', 'world', 'natural', 'language', 'processing', 'python', 'amazing']
Using spaCy:
normalized_words = [token.text.lower() for token in doc if not token.is_punct and not token.is_stop]
print("Normalized Words:", normalized_words)
Output for the above code:
Normalized Words: ['hello', 'welcome', 'world', 'natural', 'language', 'processing', 'python', 'amazing']
Example Workflow for Text Preprocessing in Python using NLTK and spaCy
Here’s a complete example demonstrating text preprocessing using NLTK and spaCy:
NLTK Example:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
import string
# Sample text
text = "NLTK is a powerful Python library for text processing. It provides tools for tokenization, stemming, and more."
# Tokenization
words = word_tokenize(text)
# Stop words removal
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
# Removing punctuation
cleaned_words = [word for word in filtered_words if word not in string.punctuation]
# Stemming
ps = PorterStemmer()
stemmed_words = [ps.stem(word) for word in cleaned_words]
print("Processed Text:", ' '.join(stemmed_words))
Output for the above code:
Processed Text: nltk power python librari text process provid tool token stem
spaCy Example:
import spacy
# Load spaCy model
nlp = spacy.load('en_core_web_sm')
# Sample text
text = "NLTK is a powerful Python library for text processing. It provides tools for tokenization, stemming, and more."
# Processing text
doc = nlp(text)
# Tokenization, stop words removal, and lemmatization
filtered_words = [token.lemma_.lower() for token in doc if not token.is_stop and not token.is_punct]
print("Processed Text:", ' '.join(filtered_words))
Output for the above code:
Processed Text: nltk powerful python library text processing provide tool tokenization stem
Conclusion
Text preprocessing is an indispensable component of any Natural Language Processing (NLP) workflow. As we’ve explored, raw text data often contains a multitude of inconsistencies, noise, and unnecessary information that can hinder the performance of NLP models. By implementing a comprehensive text preprocessing pipeline, we can transform this raw data into a cleaner, more structured, and meaningful format, ultimately leading to better analysis and more accurate results.
In this guide, we covered essential preprocessing techniques such as tokenization, stop words removal, stemming, lemmatization, and text normalization. Each of these techniques plays a vital role in preparing text data by reducing complexity, eliminating irrelevant information, and standardizing the text. Tokenization breaks down the text into manageable units, while stop words removal filters out common words that don't contribute much to the meaning. Stemming and lemmatization reduce words to their root forms, ensuring consistency across different forms of a word. Finally, normalization further ensures that the text is uniform, regardless of case or formatting differences.
We also demonstrated how to implement these techniques using popular Python libraries like NLTK and spaCy. Both libraries provide robust and user-friendly tools that make preprocessing straightforward, even for large datasets. Whether you're working on sentiment analysis, text classification, or any other NLP task, understanding and applying these preprocessing steps is crucial to achieving high-quality results.
In summary, the importance of text preprocessing in NLP cannot be overstated. It not only enhances the performance of your models but also ensures that the insights you derive from text data are accurate and reliable. By investing time in thorough preprocessing, you set a solid foundation for your entire NLP project, leading to more effective and efficient analysis. As you continue to develop your skills in NLP, mastering these preprocessing techniques will be a key factor in your success.
Comments