Understanding Arrays in JavaScript
- Samul Black
- Dec 16, 2024
- 4 min read
Arrays are one of the most fundamental and versatile data structures in JavaScript. They allow you to store multiple values in a single variable and perform various operations efficiently. In this tutorial, we will explore what arrays are, how to use them, and some of the most common methods available to manipulate arrays in JavaScript.
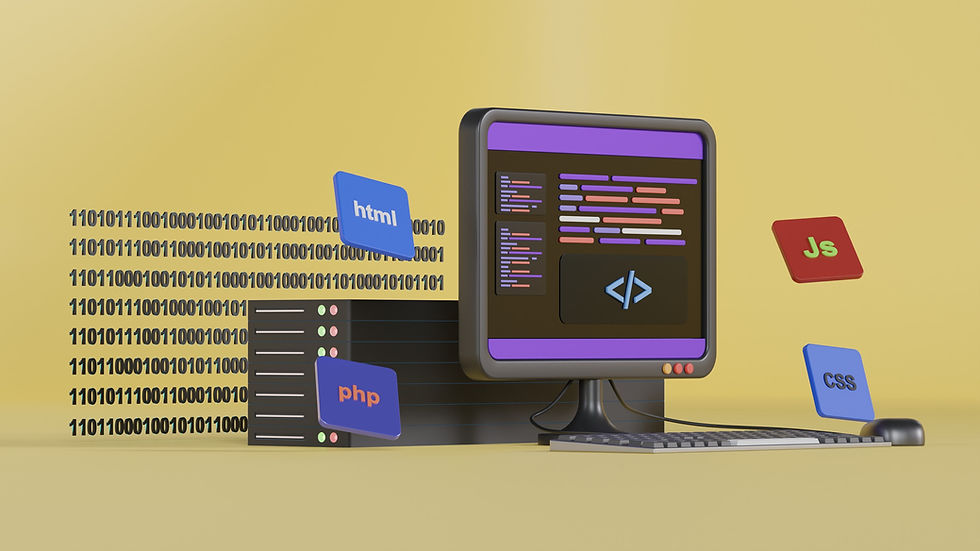
What are Arrays in JavaScript?
An array is a special data structure in JavaScript that allows you to store a collection of values in a single variable. These values can be of any data type, including numbers, strings, objects, or even other arrays. Arrays are ordered, meaning that the position of each value (known as its index) is fixed within the array. This ordering makes it easy to access, modify, or iterate over the elements of an array.
Arrays in JavaScript are dynamic, meaning their size can grow or shrink as needed. Additionally, unlike many other programming languages, arrays in JavaScript can hold mixed data types. This flexibility makes them particularly useful for various programming scenarios. Key Characteristics of Arrays include:
Indexed Collection: Elements are stored in a sequence, and each element can be accessed using its index, starting from 0.
Dynamic Size: You can add or remove elements without declaring a fixed size beforehand.
Heterogeneous Data: Arrays can store different data types together, such as numbers and strings in the same array.
Example:
let mixedArray = ["Hello", 42, true, { name: "Alice" }, [1, 2, 3]];
console.log(mixedArray);
// Output: ["Hello", 42, true, { name: "Alice" }, [1, 2, 3]]
Creating Arrays
You can create arrays in JavaScript in several ways:
Using Square Brackets (Preferred):
let fruits = ["apple", "banana", "cherry"];
Using the Array Constructor:
let numbers = new Array(1, 2, 3, 4);
Empty Arrays:
let emptyArray = [];
Accessing Array Elements
Array elements are accessed using their index. In JavaScript, array indices start at 0.
let colors = ["red", "green", "blue"];
console.log(colors[0]); // Output: red
console.log(colors[2]); // Output: blue
Modifying Arrays
You can update an element by assigning a value to a specific index:
let fruits = ["apple", "banana", "cherry"];
fruits[1] = "mango";
console.log(fruits); // Output: ["apple", "mango", "cherry"]
Common Array Methods
JavaScript provides a rich set of methods to manipulate arrays. Here are some of the most commonly used ones:
1. Adding and Removing Elements
push(): Adds an element to the end of an array.
let fruits = ["apple", "banana"];
fruits.push("cherry");
console.log(fruits); // Output: ["apple", "banana", "cherry"]
pop(): Removes the last element of an array.
let fruits = ["apple", "banana", "cherry"];
fruits.pop();
console.log(fruits); // Output: ["apple", "banana"]
unshift(): Adds an element to the beginning of an array.
let fruits = ["banana", "cherry"];
fruits.unshift("apple");
console.log(fruits); // Output: ["apple", "banana", "cherry"]
shift(): Removes the first element of an array.
let fruits = ["apple", "banana", "cherry"];
fruits.shift();
console.log(fruits); // Output: ["banana", "cherry"]
2. Iterating Over Arrays
for Loop:
let numbers = [1, 2, 3, 4];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
forEach() Method:
let numbers = [1, 2, 3, 4];
numbers.forEach((num) => console.log(num)); // Output: 1 2 3 4
3. Transforming Arrays
map(): Creates a new array by applying a function to each element.
let numbers = [1, 2, 3, 4];
let squared = numbers.map((num) => num * num);
console.log(squared); // Output: [1, 4, 9, 16]
filter(): Creates a new array with elements that pass a test.
let numbers = [1, 2, 3, 4];
let even = numbers.filter((num) => num % 2 === 0);
console.log(even); // Output: [2, 4]
4. Finding and Checking Elements
find(): Returns the first element that satisfies a condition.
let numbers = [1, 2, 3, 4];
let greaterThanTwo = numbers.find((num) => num > 2);
console.log(greaterThanTwo); // Output: 3
includes(): Checks if an array contains a value.
let fruits = ["apple", "banana", "cherry"];
console.log(fruits.includes("banana")); // Output: true
5. Sorting and Reversing
sort(): Sorts the elements of an array.
let fruits = ["banana", "apple", "cherry"];
fruits.sort();
console.log(fruits); // Output: ["apple", "banana", "cherry"]
reverse(): Reverses the order of elements in an array.
let fruits = ["apple", "banana", "cherry"];
fruits.reverse();
console.log(fruits); // Output: ["cherry", "banana", "apple"]
Multidimensional Arrays
JavaScript arrays can contain other arrays, allowing for multidimensional data structures.
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[1][2]); // Output: 6
Conclusion
Arrays are a powerful tool in JavaScript that allow you to store, access, and manipulate collections of data with ease. Their dynamic nature and rich set of methods make them an indispensable part of JavaScript programming. Whether you're managing lists, performing complex data transformations, or working with multidimensional data, arrays provide the flexibility and efficiency you need.
By mastering arrays and their various methods, you can enhance your problem-solving skills and write cleaner, more efficient code. The key to becoming proficient is consistent practice and exploring real-world scenarios where arrays can simplify your logic.
Start experimenting with arrays today, and soon you’ll find them to be one of your favorite tools in the JavaScript toolkit!
Kommentare