Dijkstra’s Algorithm is a classic and fundamental algorithm in computer science used for finding the shortest path from a starting node to all other nodes in a weighted graph. Named after its creator, Edsger W. Dijkstra, the algorithm is widely used in various applications such as network routing, geographical mapping, and optimization problems. In this blog, we’ll explore the core concepts of Dijkstra’s Algorithm, its implementation in Python, and some practical applications.
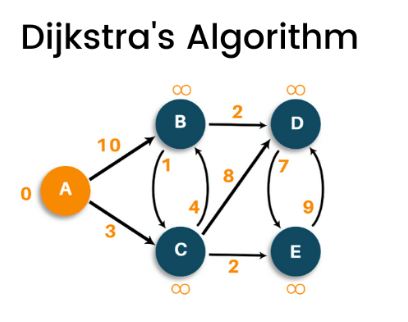
What is Dijkstra’s Algorithm?
Dijkstra’s Algorithm is a graph search algorithm that computes the shortest paths from a single source node to all other nodes in a graph with non-negative edge weights. It is particularly useful in scenarios where you need to find the shortest path in a network, such as finding the shortest route on a map or optimizing network traffic.
Key Concepts in Dijkstra’s Algorithm
Shortest Path: The minimum distance between two nodes in a graph.
Priority Queue: A data structure used to efficiently retrieve the node with the smallest distance.
Distance Vector: An array that keeps track of the shortest distance from the source node to each node in the graph.
Algorithm Steps for Dijkstra’s Algorithm
Initialization:
Set the distance to the source node to zero and all other nodes to infinity.
Initialize a priority queue with the source node.
Processing:
Extract the node with the smallest distance from the priority queue.
Update the distances of its neighbors if a shorter path is found.
Add the updated neighbors to the priority queue.
Termination:
The algorithm terminates when all nodes have been processed or the priority queue is empty.
Python Implementation for Dijkstra’s Algorithm
Let’s implement Dijkstra’s Algorithm in Python using a priority queue for efficient retrieval of the node with the smallest distance.
import heapq
def dijkstra(graph, start):
# Number of nodes in the graph
num_nodes = len(graph)
# Distance dictionary initialized to infinity
distances = {node: float('inf') for node in range(num_nodes)}
distances[start] = 0
# Priority queue for nodes to be processed
priority_queue = [(0, start)]
while priority_queue:
current_distance, current_node = heapq.heappop(priority_queue)
# If a shorter path to the current node has already been found, skip it
if current_distance > distances[current_node]:
continue
# Process each neighbor of the current node
for neighbor, weight in graph[current_node]:
distance = current_distance + weight
# Update the distance if a shorter path is found
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return distances
# Example graph represented as an adjacency list
graph = {
0: [(1, 4), (2, 1)],
1: [(3, 1)],
2: [(1, 2), (3, 5)],
3: []
}
start_node = 0
distances = dijkstra(graph, start_node)
print("Shortest distances from node", start_node, ":", distances)
Output for the above code:
Shortest distances from node 0 : {0: 0, 1: 3, 2: 1, 3: 4}
Explanation
Graph Representation: The graph is represented as an adjacency list, where each key is a node and the associated value is a list of tuples representing its neighbors and the weights of the edges.
Distance Initialization: A dictionary is used to store the shortest distance from the start node to each node, initialized to infinity. The distance to the start node is set to zero.
Priority Queue: A min-heap priority queue is used to efficiently get the node with the smallest distance. The heap operations ensure that we always process the node with the smallest known distance.
Processing Neighbors: For each node, its neighbors are evaluated. If a shorter path to a neighbor is found, the distance is updated, and the neighbor is added to the priority queue.
Output: The final distances from the start node to all other nodes are printed.
Applications of Dijkstra’s Algorithm
Network Routing: Used in routing algorithms for finding the shortest path in communication networks.
GPS Navigation: Helps in calculating the shortest driving route between locations.
Graph Analysis: Useful in various fields like transportation planning, logistics, and urban development.
Conclusion
Dijkstra’s Algorithm is a fundamental tool for solving shortest path problems in weighted graphs. Its efficiency and effectiveness make it a go-to choice for applications requiring optimal pathfinding. With the Python implementation provided, you can start applying Dijkstra’s Algorithm to your own problems and explore its many uses in real-world scenarios.
Comments