Events are one of the core concepts in JavaScript, allowing developers to make web pages dynamic and interactive. This tutorial will guide you through the basics of JavaScript events, how they work, and how you can use them in your projects.
What are Events?
In the context of web development, an event is an action or occurrence detected by the browser. Examples include:
A user clicking a button.
Hovering over an element.
Submitting a form.
Pressing a key on the keyboard.
Resizing the browser window.
JavaScript provides the ability to listen for these events and respond with custom logic, creating interactive experiences for users.
Event Listeners in JavaScript
Event listeners in JavaScript are mechanisms used to detect and respond to user interactions or other events that occur in the browser, such as clicks, keystrokes, mouse movements, form submissions, or page loads. They allow developers to execute specific code when an event is triggered on an HTML element. The addEventListener method is commonly used to attach an event listener to an element, specifying the type of event to listen for and the function to execute. For example, you can use an event listener to detect when a button is clicked and then execute a function to perform a desired action, enabling dynamic and interactive web experiences..
Syntax
// Adding an event listener to an element
element.addEventListener(eventType, callbackFunction);
element: The DOM element to attach the listener to.
eventType: The type of event to listen for (e.g., "click", "mouseover", "keydown").
callbackFunction: The function that runs when the event occurs.
Example: Click Event
<!DOCTYPE html>
<html>
<head>
<title>Event Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<p id="message"></p>
<script>
const button = document.getElementById('myButton');
const message = document.getElementById('message');
button.addEventListener('click', function() {
message.textContent = 'Button was clicked!';
});
</script>
</body>
</html>
When the button is clicked, the text content of the paragraph element changes as shown in image below:
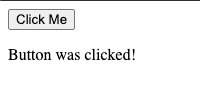
Common Event Types
Mouse Events
click: Triggered when an element is clicked.
dblclick: Triggered on a double-click.
mouseover: Triggered when the mouse pointer moves over an element.
mouseout: Triggered when the pointer moves out of an element.
Keyboard Events
keydown: Triggered when a key is pressed down.
keyup: Triggered when a key is released.
keypress: Deprecated; use keydown or keyup instead.
Form Events
submit: Triggered when a form is submitted.
focus: Triggered when an input field gains focus.
blur: Triggered when an input field loses focus.
Document Events
DOMContentLoaded: Triggered when the initial HTML document has been fully loaded and parsed.
resize: Triggered when the browser window is resized.
Event Object
When an event occurs, JavaScript passes an Event object to the event handler function. This object contains details about the event.
Example: Accessing Event Properties
<!DOCTYPE html>
<html>
<head>
<title>Event Object Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
const button = document.getElementById('myButton');
button.addEventListener('click', function(event) {
console.log('Event type:', event.type);
console.log('Button text:', event.target.textContent);
});
</script>
</body>
</html>
When the button is clicked, the event type and the button text are logged to the console.
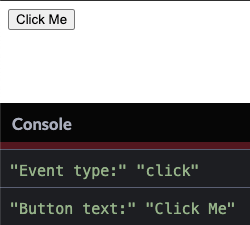
Event Propagation
Event propagation refers to the way events travel through the DOM. There are three phases:
Capturing Phase: The event starts at the root and travels down to the target element.
Target Phase: The event reaches the target element.
Bubbling Phase: The event bubbles up from the target element to the root.
Example: Bubbling and Capturing
<!DOCTYPE html>
<html>
<head>
<title>Event Propagation Example</title>
</head>
<body>
<div id="parent" style="padding: 20px; background-color: lightblue;">
Parent
<button id="child">Child</button>
</div>
<script>
const parent = document.getElementById('parent');
const child = document.getElementById('child');
parent.addEventListener('click', function() {
alert('Parent clicked');
});
child.addEventListener('click', function(event) {
alert('Child clicked');
event.stopPropagation(); // Prevents the event from bubbling to the parent
});
</script>
</body>
</html>
When the child button is clicked, only the child’s alert is shown because stopPropagation() prevents bubbling.
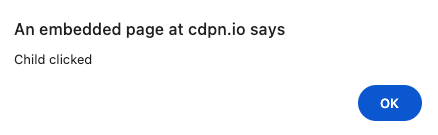
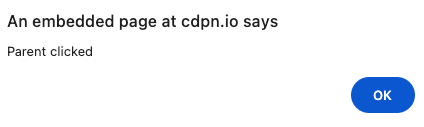
Removing Event Listeners
You can remove an event listener using the removeEventListener method.
Example
function greet() {
alert('Hello!');
}
button.addEventListener('click', greet);
button.removeEventListener('click', greet); // The event listener is removed.
Conclusion
Events are an essential part of JavaScript, enabling dynamic and interactive web applications. By understanding how to use event listeners, handle event propagation, and utilize the event object, you can create robust and user-friendly interfaces.
Experiment with different event types and scenarios to master this fundamental aspect of JavaScript!
Comments