Functions are a fundamental aspect of programming in Python. They help organize code, improve readability, and promote reusability. In this blog, we’ll explore what functions are, how to define and call them, and various advanced concepts associated with functions in Python.
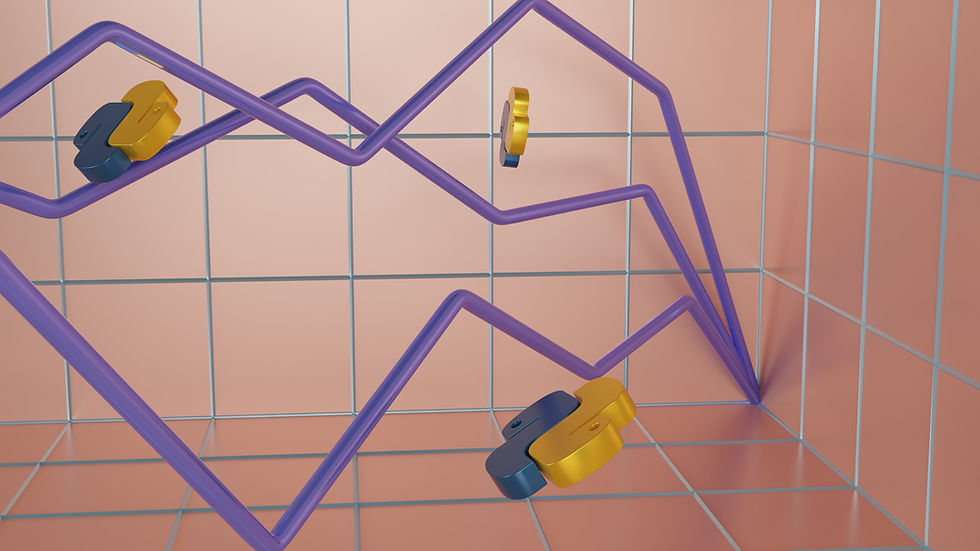
What is a Function?
A function is a reusable block of code designed to perform a specific task. When you call a function, the program executes the code within it. Functions can take inputs, process them, and return outputs, allowing you to break your program into smaller, manageable pieces.
Why Mastering Functions Matters
Enhanced Problem-Solving Skills: Understanding how to break down problems into smaller, manageable functions improves your problem-solving skills. It encourages a systematic approach to coding, allowing you to tackle complex issues more effectively.
Collaboration and Teamwork: In collaborative environments, functions help ensure that your code integrates smoothly with others'. When everyone uses well-defined functions, the project becomes more organized, and understanding each other's work becomes easier.
Testing and Debugging: Functions can be tested individually, which simplifies the debugging process. You can isolate issues more easily when they are encapsulated within functions, making it simpler to identify where things go wrong.
Performance Optimization: Functions allow for easier implementation of optimizations. You can identify which functions are bottlenecks and optimize them without needing to overhaul your entire codebase.
Exploring Advanced Concepts
As you become more comfortable with basic functions, consider exploring advanced concepts such as:
Higher-Order Functions: Functions that take other functions as arguments or return them as results. This concept is central to functional programming and can lead to powerful abstractions.
Decorators: Functions that modify the behavior of other functions. They are widely used for logging, enforcing access control, and modifying inputs or outputs.
Generators: A type of function that returns an iterator, allowing you to iterate over a sequence of values without storing them in memory all at once.
Benefits of Using Functions
Code Reusability: Write a piece of code once and use it multiple times throughout your program.
Modularity: Functions help break down complex problems into smaller, manageable parts.
Readability: Well-named functions make your code easier to read and understand.
Maintainability: Changes can be made in one place without affecting the entire codebase.
Defining a Function
In Python, you define a function using the def keyword followed by the function name and parentheses. Here's the basic syntax:
def function_name(parameters):
# Code block
return output
Example:
Let’s define a simple function that adds two numbers:
def add_numbers(a, b):
return a + b
Calling a Function
Once a function is defined, you can call it by using its name followed by parentheses. You can pass arguments inside the parentheses.
result = add_numbers(5, 3)
print(result) # Output: 8
Function Parameters
Functions can take parameters, which are inputs that the function can use. You can define functions with various types of parameters:
1. Positional Parameters
These are the most common type of parameters. They are matched with arguments based on their position.
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
2. Default Parameters
You can provide default values for parameters. If an argument isn’t passed during the function call, the default value is used.
def greet(name="Stranger"):
print(f"Hello, {name}!")
greet() # Output: Hello, Stranger!
greet("Bob") # Output: Hello, Bob!
3. Keyword Parameters
You can also pass arguments using the name of the parameters, allowing for more flexible function calls.
def introduce(name, age):
print(f"My name is {name} and I am {age} years old.")
introduce(age=25, name="Charlie") # Output: My name is Charlie and I am 25 years old.
4. Variable-Length Arguments
If you want to allow a function to take an arbitrary number of arguments, you can use args and *kwargs.
*args allows for any number of positional arguments.
**kwargs allows for any number of keyword arguments.
def display_fruits(*fruits):
for fruit in fruits:
print(fruit)
display_fruits("Apple", "Banana", "Cherry")
def display_info(**info):
for key, value in info.items():
print(f"{key}: {value}")
display_info(name="Eve", age=30, city="New York")
Returning Values
Functions can return values using the return statement. If no return statement is provided, the function returns None by default.
def square(x):
return x * x
result = square(4)
print(result) # Output: 16
Lambda Functions
In addition to regular functions, Python also supports anonymous functions, known as lambda functions. They are often used for short, throwaway functions.
square = lambda x: x * x
print(square(5)) # Output: 25
Conclusion
Functions are a powerful feature in Python that enhance code organization and efficiency. By utilizing functions, you can create cleaner, more maintainable, and reusable code. Whether you’re a beginner or an experienced developer, mastering functions is essential for effective programming in Python. To deepen your understanding of functions in Python, consider the following actions:
Practice: Implement small projects or coding challenges that require the use of functions. Websites like LeetCode, HackerRank, and Codecademy offer excellent resources for practice.
Read: Dive into Python’s documentation or books focused on Python programming. Look for chapters specifically on functions and modules.
Experiment: Try out different types of functions, play with lambda expressions, and create your own decorators or generators. The best way to learn is through hands-on experience.
Feel free to share your thoughts or ask questions about functions in Python in the comments below! Engaging with the community can provide new insights and learning opportunities. Happy coding, and may your functions always return the results you expect!
Comentarios